[Practical Exercise] MATLAB Design and Simulation of a Wireless Communication Link
发布时间: 2024-09-14 06:58:07 阅读量: 89 订阅数: 82 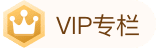
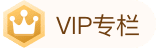
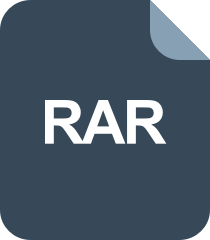
Intelligent Control Design and MATLAB Simulation
# 2.1 Wireless Channel Models in MATLAB
MATLAB offers a variety of wireless channel models to simulate real-world wireless propagation environments. These models can be categorized into the following types:
- **Flat Fading Channel Models:** Assume the channel does not have multipath propagation, and the signal experiences constant attenuation and phase shift as it travels through the channel. The typical representative is the AWGN (Additive White Gaussian Noise) channel model.
- **Frequency-Selective Fading Channel Models:** Assume the channel has multipath propagation, and the signal experiences different attenuation and phase shifts on different paths, leading to distortion of the signal in the frequency domain. The typical representative is the Rayleigh fading channel model.
- **Time-Selective Fading Channel Models:** Assume the channel has multipath propagation, and the signal experiences different time delays on different paths, leading to distortion of the signal in the time domain. The typical representative is the multipath fading channel model.
# 2. Basic Wireless Communication Link Simulation in MATLAB
### 2.1 Wireless Channel Models in MATLAB
The wireless channel is the medium through which signals are transmitted in a wireless communication link, and its characteristics greatly impact communication performance. MATLAB provides a rich set of wireless channel models for simulating different types of channel conditions.
#### 2.1.1 AWGN Channel
The AWGN (Additive White Gaussian Noise) channel is the simplest channel model, which assumes that noise is additive, independent, and follows a Gaussian distribution with a mean of 0 and a variance of σ². The AWGN channel model is commonly used to simulate the impact of Signal-to-Noise Ratio (SNR) on communication performance.
```matlab
% Create an AWGN channel object
awgnChannel = comm.AWGNChannel;
% Set the SNR
awgnChannel.EbNo = 10; % in decibels
% Transmit a signal
txSignal = randi([0 1], 1000, 1);
% Send signal through the channel
rxSignal = awgnChannel(txSignal);
% Calculate the Bit Error Rate (BER)
ber = sum(txSignal ~= rxSignal) / length(txSignal);
```
#### 2.1.2 Rayleigh Fading Channel
The Rayleigh fading channel simulates the fluctuations in signal amplitude caused by multipath propagation in a wireless channel. The Rayleigh fading channel model assumes that the signal envelope follows a Rayleigh distribution.
```matlab
% Create a Rayleigh fading channel object
rayleighChannel = comm.RayleighChannel;
% Set fading parameters
rayleighChannel.PathDelays = [0 1e-6 2e-6];
rayleighChannel.AveragePathGains = [0 -1 -3];
% Transmit a signal
txSignal = randi([0 1], 1000, 1);
% Send signal through the channel
rxSignal = rayleighChannel(txSignal);
% Calculate the Bit Error Rate (BER)
ber = sum(txSignal ~= rxSignal) / length(txSignal);
```
#### 2.1.3 Multipath Fading Channel
The multipath fading channel models the time dispersion caused by the signal propagating over different paths in a wireless channel. The multipath fading channel model represents the channel as multiple paths with different time delays and gains.
```matlab
% Create a multipath fading channel object
multipathChannel = comm.MultipathChannel;
% Set path parameters
multipathChannel.PathDelays = [0 1e-6 2e-6];
multipathChannel.AveragePathGains = [0 -1 -3];
multipathChannel.SampleRate = 1e6;
% Transmit a signal
txSignal = randi([0 1], 1000, 1);
% Send signal through the channel
rxSignal = multipathChannel(txSignal);
% Calculate the Bit Error Rate (BER)
ber = sum(txSignal ~= rxSignal) / length(txSignal);
```
### 2.2 Modulation and Demodulation Techniques in MATLAB
Modulation and demodulation techniques convert digital signals into analog signals (modulation) and recover digital signals from analog signals (demodulation). MATLAB provides various modulation and demodulation techniques for simulating different communication systems.
#### 2.2.1 BPSK Modulation
BPSK (Binary Phase Shift Keying) modulation is a simple digital modulation technique that maps binary data onto two different phases.
```matlab
% Create a BPSK modulator object
bpskModulator = comm.BPSKModulator;
% Transmit a signal
txSignal = randi([0 1], 1000, 1);
% Modulate the signal
modSignal = bpskModulator(txSignal);
```
#### 2.2.2 QPSK Modulation
QPSK (Quadrature Phase Shift Keying) modulation is a quadrature modulation technique that maps binary data onto four different phases.
```matlab
% Create a QPSK modulator object
qpskModulator = comm.QPSKModulator;
% Transmit a signal
txSignal = randi([0 1], 1000, 2); % 2-bit binary data
% Modulate the signal
modSignal = qpskModulator(txSignal);
```
#### 2.2.3 OFDM Modulation
OFDM (Orthogonal Frequency Division Multiplexing) modulation is a multicarrier modulation technique that transmits data in parallel over multiple subcarriers.
```matlab
% Create an OFDM modulator object
ofdmModulator = comm.OFDMModulator;
% Set OFDM parameters
ofdmModulator.FFTLength = 64;
ofdmModulator.NumDataCarriers = 48;
% Transmit a signal
txSignal = randi([0 1], 1000, 48); % 48-bit binary data
% Modulate the signal
modSignal = ofdmModulator(txSignal);
```
# 3. Practical Wireless Communication Link Simulation in MATLAB
### 3.1 Establishing and Simulating Channel Models
Channel models are a crucial component of wir
0
0
相关推荐







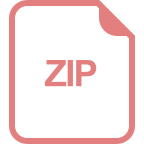