【Advanced Chapter】Speech Enhancement and Noise Reduction Techniques in MATLAB
发布时间: 2024-09-14 06:38:47 阅读量: 50 订阅数: 82 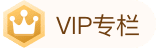
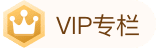
# 2.1 Time-Domain Filtering Algorithms
Time-domain filtering algorithms directly process the time series of speech signals by eliminating or suppressing noise components to enhance the speech signal. Time-domain filtering algorithms mainly include:
- Average filtering: Calculate the average value of neighboring samples for each sample point in the signal and replace the original sample with this average value.
- Median filtering: Calculate the median of neighboring samples for each sample point in the signal and replace the original sample with this median.
- Wiener filtering: A linear filter that estimates the signal by minimizing the mean square error of the difference between the signal and noise.
# 2. MATLAB Speech Enhancement and Denoising Algorithms
MATLAB, as a powerful scientific computing software, offers a wealth of speech enhancement and denoising algorithms, covering various types such as time-domain filtering, frequency-domain filtering, and statistical model algorithms.
### 2.1 Time-Domain Filtering Algorithms
Time-domain filtering algorithms process the time-domain data of speech signals directly to enhance speech by filtering out noise components.
#### 2.1.1 Average Filtering
Average filtering is a simple time-domain filtering algorithm that smooths the signal by taking the average of neighboring data points of the signal, thereby suppressing noise. In MATLAB, the `filter` function is used to implement average filtering, with the syntax as follows:
```
y = filter(b, a, x)
```
Here, `x` is the input signal, and `b` and `a` are the numerator and denominator coefficients of the filter, respectively. For average filtering, `b` is a vector with a length equal to the filter order, where all elements are 1; `a` is a vector with a length of 1, whose element is the filter order.
**Code Block:**
```
% Input signal
x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
% Average filter order
order = 3;
% Average filtering
y = filter(ones(1, order) / order, 1, x);
% Plot original signal and filtered signal
figure;
plot(x, 'b', 'LineWidth', 2);
hold on;
plot(y, 'r', 'LineWidth', 2);
legend('Original Signal', 'Average Filtered Signal');
xlabel('Sampling Points');
ylabel('Amplitude');
title('Average Filtering Effect');
```
**Logical Analysis:**
* The `filter` function uses the numerator coefficient `b` and the denominator coefficient `a` to filter the input signal `x`, outputting the filtered signal `y`.
* The order of the average filter determines the smoothness of the filter; the higher the order, the more pronounced the smoothing effect.
* Plotting the original and filtered signals allows for a直观 observation of the average filtering effect.
#### 2.1.2 Median Filtering
Median filtering is a nonlinear time-domain filtering algorithm that smooths the signal by taking the median of neighboring data points of the signal, thereby suppressing noise. In MATLAB, the `medfilt1` function is used to implement median filtering, with the syntax as follows:
```
y = medfilt1(x, order)
```
Here, `x` is the input signal, and `order` is the filter order, indicating the size of the filter window.
**Code Block:**
```
% Input signal
x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
% Median filter order
order = 3;
% Median filtering
y = medfilt1(x, order);
% Plot original signal and filtered signal
figure;
plot(x, 'b', 'LineWidth', 2);
hold on;
plot(y, 'r', 'LineWidth', 2);
legend('Original Signal', 'Median Filtered Signal');
xlabel('Sampling Points');
ylabel('Amplitude');
title('Median Filtering Effect');
```
**Logical Analysis:**
* The `medfilt1` function uses the filter order `order` to perform median filtering on the input signal `x`, outputting the filtered signal `y`.
* The median filter order `order` determines the size of the filter window; the larger the window, the more pronounced the smoothing effect.
* Plotting the original and filtered signals allows for a直观 observation of the median filtering effect.
#### 2.1.3 Wiener Filtering
Wiener filtering is a time-domain filtering algorithm that designs a filter by estimating the power spectral density function (PSD) of noise to suppress noise. In MATLAB, the `wiener2` function is used to implement Wiener filtering, with the syntax as follows:
```
y = wiener2(x, nsd)
```
Here, `x` is the input signal, and `nsd` is the noise power spectral density function.
**Code Block:**
```
% Input signal
x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
% Noise power spectral density function (PSD)
nsd = 0.1;
% Wiener filtering
y = wiener2(x, nsd);
% Plot original signal and filtered signal
figure;
plot(x, 'b', 'LineWidth', 2);
hold on;
plot(y, 'r', 'LineWidth', 2);
legend('Original Signal', 'Wiener Filtered Signal');
xlabel('Sampling Points');
ylabel('Amplitude');
title('Wiener Filtering Effect');
```
**Logical Analysis:**
* The `wiener2` function uses the noise power spectral density function `nsd` to perform Wiener filtering on the input signal `x`, outputting the filtered signal `y`.
* The noise power spectral density function `nsd` determines the characteristics of the filter; the higher the `nsd`, the more pronounced the filtering effect.
* Plotting the original and filtered signals allows for a直观 observation of the Wiener filtering effect.
# 3. MATLAB Speech Enhancement and Denoising Practice
### 3.1 Noise Estimation
Noise estimation is the first step in speech enhancement and denoising, ***mon noise estimation methods include:
#### 3.1.1 Silence Segment Detection
Silence segment detection utilizes the energy distribution characteristics of the speech signal to identify the silence segments within the speech signal. In silence segments, the energy of the speech signal is low, while the noise energy is relatively high. Therefore, by detecting silence segments, the noise power spectrum can be estimated.
```
% Silence segment detection
[silence_start, silence_end] = detect_silence(speech_signal, threshold);
% Estimate noise power spectrum
noise_psd = estimate_noise_psd(speech_signal, silence_start, silence_end);
```
#### 3.1.2 Power Spectrum Estimation
Power spectrum estimation is another commonly used noise estimation method. It util***mon power spectrum estimation methods include:
- **Periodogram Method:** Segment the speech signal into frames, calculate the power spectrum of each frame, and then take
0
0