【Practical Exercise】Design and Implementation of a Basic Digital Communication System Using MATLAB
发布时间: 2024-09-14 07:02:41 阅读量: 29 订阅数: 71 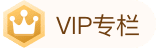
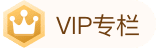
# 2.1 Modulation Principles and Basic Concepts
### 2.1.1 Modulation and Demodulation
Modulation is the process of converting digital signals into analog signals for transmission over analog channels. Demodulation is the reverse process of modulation, restoring analog signals back into digital signals.
### 2.1.2 Types of Modulation
Modulation types are categorized based on the characteristics of the carrier signal:
- Amplitude Shift Keying (ASK): Alters the amplitude of the carrier signal
- Frequency Shift Keying (FSK): Alters the frequency of the carrier signal
- Phase Shift Keying (PSK): Alters the phase of the carrier signal
# 2. MATLAB Digital Modulation Techniques
### 2.1 Modulation Principles and Basic Concepts
#### 2.1.1 Modulation and Demodulation
Modulation is a process that converts digital signals into analog signals for transmission through analog channels. Demodulation, the inverse of modulation, is the process of restoring analog signals back into digital signals.
#### 2.1.2 Types of Modulation
There are various modulation techniques, which can be classified based on different modulation parameters:
- Amplitude Modulation (ASK)
- Frequency Modulation (FSK)
- Phase Modulation (PSK)
### 2.2 Common Digital Modulation Techniques
#### 2.2.1 ASK Modulation
ASK modulation represents digital signals by varying the amplitude of the carrier.
```matlab
% ASK modulation
carrier_frequency = 1000; % Carrier frequency
bit_rate = 100; % Bit rate
data = [***]; % Digital signal
% Generate ASK modulated signal
t = 0:1/carrier_frequency:length(data)/bit_rate;
modulated_signal = data .* cos(2*pi*carrier_frequency*t);
% Plot the modulated signal
figure;
plot(t, modulated_signal);
title('ASK Modulated Signal');
xlabel('Time (s)');
ylabel('Amplitude');
```
**Logical Analysis:**
* `carrier_frequency`: Carrier frequency
* `bit_rate`: Bit rate
* `data`: Digital signal
* `t`: Time vector
* `modulated_signal`: ASK modulated signal
#### 2.2.2 FSK Modulation
FSK modulation represents digital signals by varying the frequency of the carrier.
```matlab
% FSK modulation
carrier_frequency = 1000; % Carrier frequency
frequency_deviation = 100; % Frequency deviation
bit_rate = 100; % Bit rate
data = [***]; % Digital signal
% Generate FSK modulated signal
t = 0:1/carrier_frequency:length(data)/bit_rate;
modulated_signal = zeros(1, length(t));
for i = 1:length(data)
if data(i) == 0
modulated_signal(i) = cos(2*pi*(carrier_frequency - frequency_deviation)*t(i));
else
modulated_signal(i) = cos(2*pi*(carrier_frequency + frequency_deviation)*t(i));
end
end
% Plot the modulated signal
figure;
plot(t, modulated_signal);
title('FSK Modulated Signal');
xlabel('Time (s)');
ylabel('Amplitude');
```
**Logical Analysis:**
* `carrier_frequency`: Carrier frequency
* `frequency_deviation`: Frequency deviation
* `bit_rate`: Bit rate
* `data`: Digital signal
* `t`: Time vector
* `modulated_signal`: FSK modulated signal
#### 2.2.3 PSK Modulation
PSK modulation represents digital signals by varying the phase of the carrier.
```matlab
% PSK modulation
carrier_frequency = 1000; % Carrier frequency
phase_shift = pi/2; % Phase shift
bit_rate = 100; % Bit rate
data = [***]; % Digital signal
% Generate PSK modulated signal
t = 0:1/carrier_frequency:length(data)/bit_rate;
modulated_signal = zeros(1, length(t));
for i = 1:length(data)
if data(i) == 0
modulated_signal(i) = cos(2*pi*carrier_frequency*t(i));
else
modulated_signal(i) = cos(2*pi*carrier_frequency*t(i) + phase_shift);
end
end
% Plot the modulated signal
figure;
plot(t, modulated_signal);
title('PSK Modulated Signal');
xlabel('Time (s)');
ylabel('Amplitude');
```
**Logical Analysis:**
* `carrier_frequency`: Carrier frequency
* `phase_shift`: Phase shift
* `bit_rate`: Bit rate
* `data`: Digital signal
* `t`: Time vector
* `modulated_signal`: PSK modulated signal
### 2.3 Performance Analysis of Digital Modulation Techniques
#### 2.3.1 Bandwidth and Bit Rate
The bandwidth of modulation techniques is closely related to the bit rate. Bandwidth refers to the range of spectrum occupied by the modulated signal, while bit rate refers to the number of bits transmitted per second. Generally, the higher the bit rate, the greater the bandwidth required.
#### 2.3.2 Noise Resistance Performance
The noise resistance performance of modulation techniques refers to their ability to transmit signals in the presence of noise. Different modulation techniques have varying sensitivities to noise. For example, PSK modulation has a lower sensitivity to noise, whereas ASK modulation has a higher sensitivity.
# 3.1 Demodulation Principles and Basic Concepts
#### 3.1.1 Demodulation and Modulation
Demodulation is the reverse process of restoring the original information from the modulated signal. Like modulation, demodulation involves the processing of the carrier signal and the modulated signal. Demodulators receive the modulated signal and extract the original information using various techniques.
#### 3.1.2 Types of Demodulation
There are various types of demodulation techniques, each suited for different modulation techniques. The most common types include:
- **Coherent Demodulation:** Uses a reference carrier signal to demodulate the modulated signal.
0
0
相关推荐
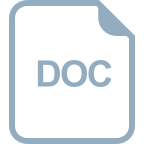
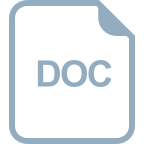
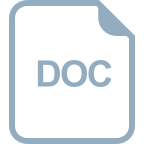
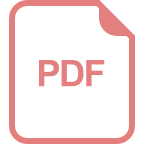
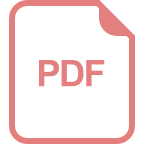
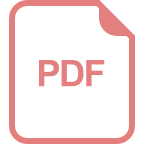
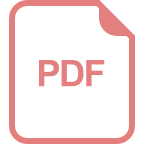
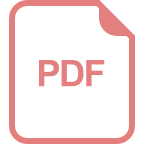
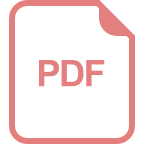