【Fundamentals】Voice Signal Synthesis in MATLAB: Understanding Speech Synthesis Technologies and TTS Systems
发布时间: 2024-09-14 06:06:44 阅读量: 59 订阅数: 83 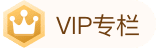
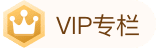
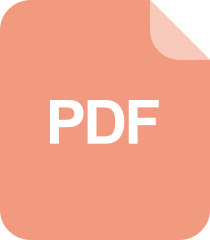
Color in Computer Vision:Fundamentals and Applications
# 2.1 Text-to-Speech (TTS) Engine Synthesis
## 2.1.1 Principles and Selection of TTS Engines
A Text-to-Speech (TTS) engine is a software capable of transforming textual input into speech output. The working principle of a TTS engine involves breaking down text into a sequence of phonemes and then employing speech synthesis algorithms to convert these phoneme sequences into speech waveforms.
When selecting a TTS engine, consider the following factors:
- **Speech Quality:** The naturalness and intelligibility of speech generated by the TTS engine.
- **Supported Languages:** The quantity and quality of languages supported by the TTS engine.
- **Customization Capabilities:** Whether the TTS engine allows users to customize speech output, such as speaking rate, pitch, and tone.
- **Availability:** Whether the TTS engine is free or commercial, and its ease of integration into MATLAB.
## 2.1.2 Usage of TTS Engines in MATLAB
MATLAB offers several built-in TTS engines, including:
- **text2speech:** A simple TTS engine that supports basic text-to-speech conversion.
- **webvoices:** A more advanced TTS engine that supports multiple languages and customization options.
To use TTS engines in MATLAB, follow these steps:
1. Create a text2speech or webvoices object.
2. Set engine properties, such as language, speaking rate, and pitch.
3. Use the speak() method to convert text into speech.
For example, the following code uses the text2speech engine to transform the text "Hello, world!" into speech:
```matlab
engine = text2speech;
engine.Rate = 1.2;
engine.Pitch = 1.1;
speak(engine, 'Hello, world!');
```
# 2. Speech Synthesis Methods in MATLAB
MATLAB provides a variety of speech synthesis methods to cater to different needs and application scenarios. This chapter will introduce two main speech synthesis methods: text-to-speech (TTS) engine-based synthesis and parameter-based synthesis methods.
## 2.1 Text-to-Speech (TTS) Engine-Based Synthesis
### 2.1.1 Principles and Selection of TTS Engines
A TTS engine is a software component that converts textual input into speech output. Its principles are as follows:
- Text preprocessing: Segmentation of text input, punctuation processing, and phoneme conversion.
- Speech synthesis: Generation of speech waveforms using pre-trained speech models based on preprocessed text.
MATLAB supports various TTS engines, including:
- **TextToSpeechSystem:** MATLAB's built-in TTS engine, providing basic speech synthesis capabilities.
- **Google Text-to-Speech:** A TTS engine provided by Google, offering high-quality speech synthesis effects.
- **Amazon Polly:** A TTS engine provided by Amazon, supporting multiple languages and speech styles.
When choosing a TTS engine, consider the following factors:
- **Speech Quality:** The quality of speech generated by different engines may vary, so choose according to actual needs.
- **Supported Languages:** The number and types of languages supported by the TTS engine.
- **Customization Capabilities:** Some engines allow users to customize speech parameters, such as speaking rate, pitch, and volume.
- **Cost:** Commercial TTS engines typically require payment for use.
### 2.1.2 Using TTS Engines in MATLAB
To perform speech synthesis using TTS engines in MATLAB, follow these steps:
1. Create a TextToSpeechSystem object:
```
tts = textToSpeechSystem;
```
2. Set engine parameters:
```
tts.Voice = 'Google US English'; % Set the voice engine and language
tts.Rate = 1.2; % Set the speaking rate
```
3. Synthesize speech:
```
audio = synthesize(tts, 'Hello world'); % Synthesize speech and store in the audio variable
```
4. Play speech:
```
sound(audio); % Play the synthesized speech
```
### 2.2 Parameter-Based Synthesis Methods
#### 2.2.1 Extraction and Modeling of Speech Parameters
Parameter-based synthesis methods generate speech by extracting and modeling speech parameters. Speech parameters include:
- **Pitch (F0):** The frequency of the sound.
- **Loudness (A):** The volume of the sound.
- **Formants:** Frequency peaks of harmonics in the sound.
Extraction and modeling of speech parameters can use the following techniques:
- **Linear Predictive Coding (LPC):** A widely used method for extracting speech parameters, estimating parameters by predicting future values of the speech signal.
- **Mel-Frequency Cepstral Coefficients (MFCC):** A speech parameter extraction method based on the human auditory system, converting speech signals into the Mel frequency domain.
- **Hidden Markov Models (HMM):** A statistical model used for speech parameter modeling and sequence prediction.
#### 2.2.2 Implementation of Parameter Synthesis Algo
0
0
相关推荐







