【Basic】Signal Encoding and Decoding in MATLAB: Implementing PCM, DPCM, and ADPCM Coding
发布时间: 2024-09-14 05:42:53 阅读量: 69 订阅数: 71 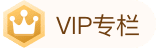
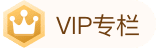
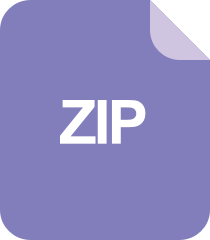
Arithimatic Encoding And Decoding: Arithimatic Encoding And Decoding with block code length-matlab开发
# 1. An Overview of Signal Encoding and Decoding
Signal encoding and decoding are fundamental techniques in digital signal processing, used to convert analog signals into digital signals for easier storage, transmission, and processing. The encoding process involves discretizing continuous analog signals into digital values, while the decoding process restores these digital values back into analog signals.
Signal encoding and decoding technologies are widely used in various fields such as communications, audio, video, and image processing. Through encoding, we can effectively reduce the bandwidth required for signal transmission, thereby increasing transmission efficiency. Moreover, the digital signals after encoding can be conveniently stored and processed, laying the foundation for various digital signal processing algorithms.
# 2. Pulse Code Modulation (PCM)
### 2.1 The Basic Principles of PCM
Pulse code modulation (PCM) is a digital technique for converting analog signals into digital signals. The fundamental principle involves sampling and quantizing the continuous analog signal, and then encoding the quantized samples into a binary bitstream.
Sampling refers to taking samples of the analog signal at a certain frequency to obtain signal values at discrete time points. Quantization involves discretizing the sampled values by mapping them to a finite set of discrete values. Encoding refers to converting the quantized values into a binary bitstream.
### 2.2 Quantization and Encoding in PCM
**Quantization**
***mon quantization methods include:
- **Uniform Quantization:** Dividing the analog signal values uniformly into equally spaced quantization levels and mapping each sample value to the nearest quantization level.
- **Non-uniform Quantization:** Dividing the analog signal values into unequally spaced quantization levels based on the distribution characteristics of the analog signal, to improve quantization accuracy.
**Encoding**
***mon encoding methods include:
- **Linear Encoding:** Directly converting the quantized values into a binary bitstream.
- **Non-linear Encoding:** Using non-linear encoding algorithms, such as logarithmic or exponential encoding, to improve encoding efficiency.
### 2.3 Decoding and Restoration in PCM
**Decoding**
The bitstream encoded with PCM needs to be decoded to restore the original analog signal. The decoding process is the reverse of the encoding process, converting the binary bitstream back into quantized values.
**Restoration**
The quantized values need to be restored into a continuous analog signal. The restoration process uses the same algorithm as quantization, mapping the quantized values back to continuous analog signal values.
```python
import numpy as np
# Analog signal
analog_signal = np.sin(2 * np.pi * 1000 * np.linspace(0, 1, 1000))
# Sampling frequency
fs = 8000
# Sampling
sampled_signal = analog_signal[::int(fs / 1000)]
# Quantization
quantized_signal = np.round(sampled_signal * 100) / 100
# Encoding
encoded_signal = np.array([bin(int(x))[2:] for x in quantized_signal])
# Decoding
decoded_signal = np.array([int(x, 2) for x in encoded_signal])
# Restoration
restored_signal = decoded_signal / 100
# Plot the original signal and the restored signal
import matplotlib.pyplot as plt
plt.plot(analog_signal, label="Original Signal")
plt.plot(restored_signal, label="Restored Signal")
plt.legend()
plt.show()
```
**Code Logic Analysis:**
* Sampling: The analog signal is sampled at a frequency of 8000 Hz using `sampled_signal = analog_signal[::int(fs / 1000)]`.
* Quantization: The sampled values are quantized into 100 discrete values using `quantized_signal = np.round(sampled_signal * 100) / 100`.
* Encoding: The quantized values are encoded into a binary bitstream using `encoded_signal = np.array([bin(int(x))[2:] for x in quantized_signal])`.
* Decoding: The binary bitstream is decoded back in
0
0
相关推荐
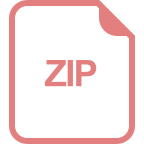
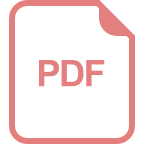
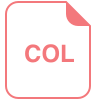
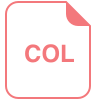
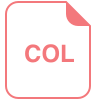
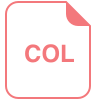
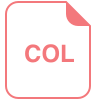
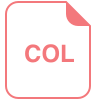