[Practical Exercise] Signal Denoising Technique Implementation Using MATLAB
发布时间: 2024-09-14 07:00:38 阅读量: 21 订阅数: 42 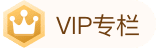
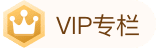
# 1. Fundamental Theory of Signal Denoising
Signal denoising is a fundamental task in digital signal processing aimed at removing unnecessary noise components from signals to enhance their quality and intelligibility. Noise can originate from a variety of sources, such as electronic devices, environmental interference, or data transmission errors.
The theory of signal denoising is built upon statistical signal processing and linear system theory. It involves using mathematical models to characterize noise and signals, and developing algorithms to effectively separate them. Signal denoising algorithms are typically divided into two main categories: time-domain methods and frequency-domain methods.
# 2. MATLAB Signal Denoising Methods
### 2.1 Time-domain based denoising methods
#### 2.1.1 Moving Average Filtering
**Principle:**
Moving average filtering is a time-domain filtering technique that smooths signals by calculating the average of the surrounding data points for each data point in the signal.
**Code Implementation:**
```matlab
% Define the filter window size
window_size = 5;
% Apply moving average filtering to the signal
filtered_signal = movmean(signal, window_size);
```
**Logical Analysis:**
* The `movmean` function calculates the average of the `window_size` number of data points around each data point in the signal.
* The `window_size` parameter specifies the size of the filter window. A larger window size produces a smoother signal but may result in loss of detail.
#### 2.1.2 Median Filtering
**Principle:**
Median filtering is another time-domain filtering technique that smooths signals by calculating the median of the surrounding data points for each data point in the signal.
**Code Implementation:**
```matlab
% Define the filter window size
window_size = 5;
% Apply median filtering to the signal
filtered_signal = medfilt1(signal, window_size);
```
**Logical Analysis:**
* The `medfilt1` function calculates the median of the `window_size` number of data points around each data point in the signal.
* Median filtering is effective at removing non-Gaussian noise such as impulse noise, but it may cause blurring at the edges of the signal.
### 2.2 Frequency-domain based denoising methods
#### 2.2.1 Fourier Transform Denoising
**Principle:**
Fourier transform denoising is a frequency-domain filtering technique that smooths signals by transforming the signal into the frequency domain, removing noise components, and then transforming the signal back into the time domain.
**Code Implementation:**
```matlab
% Perform Fourier transform on the signal
fft_signal = fft(signal);
% Set noise threshold
noise_threshold = 0.05;
% Filter noise components
filtered_fft_signal = fft_signal;
filtered_fft_signal(abs(fft_signal) < noise_threshold) = 0;
% Convert the signal back to the time domain
filtered_signal = ifft(filtered_fft_signal);
```
**Logical Analysis:**
* The `fft` function converts the signal into the frequency domain.
* The `noise_threshold` parameter specifies the noise threshold. Frequencies below this threshold are considered noise and are removed.
* The `ifft` function converts the signal back from the frequency domain to the time domain.
#### 2.2.2 Wavelet Transform Denoising
**Principle:**
Wavelet transform denoising is a multi-scale filtering technique that smooths signals by decomposing the signal into wavelet coefficients at different scales, removing noise components, and then reconstructing the signal.
**Code Implementation:**
```matlab
% Define wavelet name
wavelet_name = 'db4';
% Define the number of decomposition levels
decomposition_level = 5;
% Apply wavelet transform denoising to the signal
[cA, cD] = dwt(signal, wavelet_name, decomposition_level);
% Set noise threshold
noise_threshold = 0.05;
% Filter noise components
filtered_cD = wthresh(cD, noise_threshold, 's');
% Reconstruct the signal
filtered_signal = idwt(cA, filtered_cD, wavelet_name);
``
```
0
0
相关推荐
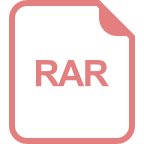
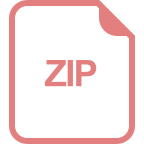
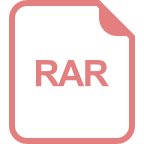





