Signal Detection and Estimation in MATLAB: Understanding Matched Filters and Maximum Likelihood Detection
发布时间: 2024-09-14 05:51:19 阅读量: 37 订阅数: 71 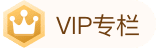
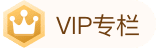
# 2.1 Basic Principles of Matched Filters
### 2.1.1 Correlation and Convolution
Correlation measures the similarity between two signals, defined as the integral of the product of one signal as it is shifted in time relative to the other. Convolution is a special case of correlation where one of the signals is flipped.
### 2.1.2 Design of Matched Filters
A matched filter is a linear filter whose frequency response matches the complex conjugate frequency spectrum of the signal to be detected. It achieves optimal signal detection by maximizing the correlation between the signal and the filter output. The design of a matched filter involves determining the filter's transfer function, which is given by:
```
H(f) = K * S*(f)
```
where:
* H(f) is the transfer function of the matched filter
* K is a normalization constant
* S*(f) is the complex conjugate frequency spectrum of the signal to be detected
# 2. Matched Filter Theory and Practice
### 2.1 Basic Principles of Matched Filters
#### 2.1.1 Correlation and Convolution
**Correlation** measures the similarity between two signals, defined as:
```
R_{xy}(τ) = ∫_{-∞}^{∞} x(t) y(t - τ) dt
```
where:
* `x(t)` and `y(t)` are the two signals
* `τ` is the time shift
**Convolution** is a special case of correlation where one signal is flipped and shifted along the time axis:
```
x(t) * y(t) = ∫_{-∞}^{∞} x(u) y(t - u) du
```
#### 2.1.2 Design of Matched Filters
A matched filter is a linear time-invariant filter whose frequency response matches the complex conjugate time-domain waveform of the signal. The design steps are as follows:
1. **Compute the complex conjugate time-domain waveform of the signal:** `h(t) = x^*(t)`
2. **Take the Fourier transform of the complex conjugate time-domain waveform:** `H(f) = F[h(t)]`
3. **Take the complex conjugate of the Fourier transform result:** `H^*(f)`
### 2.2 Application of Matched Filters in Signal Detection
#### 2.2.1 Signal Detection in Noisy Environments
In noisy environments, matched filters can enhance the contrast between the target signal and the noise. The operating principle is as follows:
1. **Convolve the received signal with the matched filter:** `y(t) = x(t) * h(t)`
2. **Take the absolute value of the convolution result:** `|y(t)|`
3. **Locate the peak of the absolute values:** The peak corresponds to the estimated arrival time of the signal
#### 2.2.2 Performance Evaluation of Matched Filters
The performance of matched filters is typically evaluated using the following metrics:
***Signal-to-Noise Ratio (SNR):** The ratio of signal power to noise power
***Detection Probability:** The probability of correctly detecting the signal
***False Alarm Probability:** The probability of incorrectly detecting noise as signal
**Table 2.1: Performance Metrics for Matched Filters**
| Metric | Formula |
|---|---|
| SNR | SNR = 10 log10(P_s / P_n) |
| Detection Probability | P_d = P(H_1 | H_1) |
| False Alarm Probability | P_fa = P(H_1 | H_0) |
where:
* `P_s` is the signal power
* `P_n` is the noise power
* `H_1` is the hypothesis of signal presence
* `H_0` is the hypothesis of signal absence
**Code Block:**
```python
import numpy as np
from scipy.signal import correlate
# Signal
x = np.array([1, 2, 3, 4, 5])
# Noise
n = np.random.randn(len(x))
# Matched filter
h = np.flip(np.conj(x))
# Convolution
y = correlate(x, h)
# Take absolute value
y_abs = np.abs(y)
# Find the peak
peak_index = np.argmax(y_abs)
# Estimate arrival time
tau = peak_index - len(x) + 1
# Print estimated arrival time
print("Estimated arrival time:", tau)
```
**Code Logic Analysis:**
* The `correlate()` function is used to calculate the convolution of the signal and the matched filter.
* The absolute value of the convolution result is taken to enhance the contrast between the
0
0
相关推荐
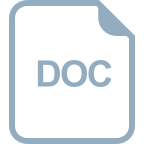
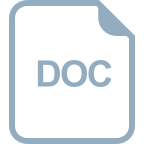
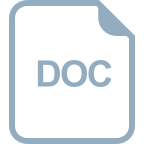
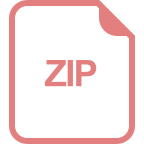
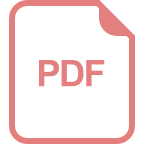
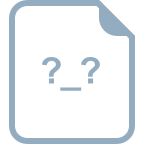
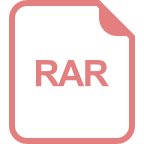
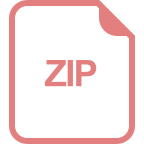