【Advanced】Signal Beamforming and Spatial Filtering in MATLAB
发布时间: 2024-09-14 06:17:59 阅读量: 70 订阅数: 83 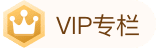
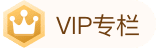
# Theoretical Foundations of Beamforming
Beamforming is a technique that enhances signals from a specific direction by coherently processing signals received from multiple sensors, while suppressing interference and noise from other directions. Its fundamental principle is based on the phenomenon of wave interference.
When multiple sensors receive the same signal, due to the differences in signal propagation distances, the signal phase received at the sensors will vary. By adjusting these phases, signals from a specific direction can be made to be in phase, thus achieving signal enhancement. Signals from other directions, due to different phases, will cancel each other out, thus achieving interference suppression.
# Beamforming Implementation in MATLAB
### 2.1 Basic Beamforming Algorithm
The basic beamforming algorithm is the simplest and most commonly used method in beamforming. It forms a beam by summing up or phase-aligning signals from all sensors in the array.
#### 2.1.1 Delay-and-sum Beamforming
Delay-and-sum beamforming forms a beam by adding up signals from all sensors in the array. This method can effectively suppress interference signals from the array's sides, but it cannot distinguish signals from different directions.
**Code block:**
```
% Delay-and-sum beamforming
N = 10; % Number of sensors
theta = 0:0.1:360; % Range of angles
delay = zeros(N, length(theta));
for i = 1:N
delay(i, :) = (i-1) * d * sin(theta * pi / 180) / c;
end
signal = exp(1j * 2 * pi * f * delay);
beamformed_signal = sum(signal, 1);
% Plotting the beam pattern
figure;
polarplot(theta, abs(beamformed_signal));
title('Delay-and-sum Beam Pattern');
```
**Logical Analysis:**
* The `delay` matrix stores the delay values for each sensor signal to compensate for the differences in signal propagation time.
* The `signal` matrix contains signals from all sensors.
* The `beamformed_signal` is the signal after beamforming.
* The `polarplot` function plots the beam pattern, showing the gain at different angles.
#### 2.1.2 Phase-sum Beamforming
Phase-sum beamforming forms a beam by phase-aligning signals from all sensors in the array. This method can effectively suppress interference signals from the array's sides and distinguish signals from different directions.
**Code block:**
```
% Phase-sum beamforming
N = 10; % Number of sensors
theta = 0:0.1:360; % Range of angles
phase_shift = zeros(N, length(theta));
for i = 1:N
phase_shift(i, :) = (i-1) * d * sin(theta * pi / 180) * 2 * pi / lambda;
end
signal = exp(1j * phase_shift);
beamformed_signal = sum(signal, 1);
% Plotting the beam pattern
figure;
polarplot(theta, abs(beamformed_signal));
title('Phase-sum Beam Pattern');
```
**Logical Analysis:**
* The `phase_shift` matrix stores the phase shift values for each sensor signal to compensate for the phase differences in signal propagation.
* The `signal` matrix contains signals from all sensors.
* The `beamformed_signal` is the signal after beamforming.
* The `polarplot` function plots the beam pattern, showing the gain at different angles.
### 2.2 Adaptive Beamforming Algorithms
Adaptive beamforming algorithms can dynamically adjust the beam shape according to the signal environment to suppress interference signals and enhance target signals.
#### 2.2.1 Minimum Mean Square Error (MSE) Algorithm
The minimum mean square error (MSE) algorithm adjusts the beam weights by minimizing the mean square error of the beamforming output signal. This algorithm can effectively suppress interference signals from the array's sides and distinguish signals from different directions.
**Code block:**
```
% MSE-based beamforming
N = 10; % Number of sensors
theta = 0:0.1:360; % Range of angles
R = cov(signal); % Signal covariance matrix
P = inv(R); % Inverse of the covariance matrix
w = P * steering_vector(theta); % Beam weights
beamformed_signal = w' * signal;
% Plotting the beam pattern
figure;
polarplot(theta, abs(beamformed_signal));
title('MSE-based Beam Pattern');
```
**Logical Analysis:**
* The `R` matrix is the signal covariance matrix.
* The `P` matrix is the inverse of the covariance matrix.
* The `steering_vector` function returns the steering vector for a given angle.
* The `w` vector is the beam weight.
* The `beamformed_signal` is the signal af
0
0
相关推荐







