【Basic】Fast Fourier Transform (FFT) Principles and MATLAB DSP Simulation Implementation
发布时间: 2024-09-14 05:39:14 阅读量: 97 订阅数: 71 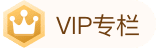
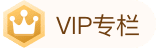
# 1. Fast Fourier Transform (FFT) Basics
The Fast Fourier Transform (FFT) is an efficient algorithm used to compute the Discrete Fourier Transform (DFT). The DFT converts a time-domain signal into a frequency-domain signal, revealing the frequency components of the signal. By exploiting the special structure of the DFT, the FFT dramatically reduces the computational workload, making it widely applicable in signal processing, image processing, and other fields.
# 2. FFT Algorithm Theory
### 2.1 Discrete Fourier Transform (DFT)
#### 2.1.1 Definition and Properties of DFT
The Discrete Fourier Transform (DFT) is a mathematical transformation that converts a time-domain signal into its frequency-domain representation. For a discrete signal x[n] of length N, the DFT is defined as:
```
X[k] = Σ_{n=0}^{N-1} x[n]e^(-j2πkn/N)
```
Where:
* X[k] is the k-th frequency component in the frequency domain
* x[n] is the n-th sampled value of the time-domain signal
* N is the length of the signal
* j is the imaginary unit
The DFT has the following properties:
* Linearity: The DFT is linear, meaning for any two signals x[n] and y[n], and any constants a and b:
```
DFT(ax[n] + by[n]) = aDFT(x[n]) + bDFT(y[n])
```
* Periodicity: The DFT is a periodic function with a period of N.
* Symmetry: The real and imaginary parts of the DFT exhibit symmetry, i.e.:
```
Re(X[k]) = Re(X[N-k])
Im(X[k]) = -Im(X[N-k])
```
#### 2.1.2 Computation of DFT
The complexity of directly computing the DFT is O(N^2), which is computationally intensive for large-scale signals. Therefore, the Fast Fourier Transform (FFT) algorithm is commonly used to compute the DFT.
### 2.2 FFT Algorithm Principles
#### 2.2.1 Derivation of FFT Algorithm
The FFT algorithm is based on the recursive decomposition of the DFT, splitting an N-point DFT into two N/2-point DFTs. The derivation process is as follows:
For a signal of length N, x[n], split it into even and odd parts:
```
x[2n] = x[0], x[2], ..., x[N-2]
x[2n+1] = x[1], x[3], ..., x[N-1]
```
The DFT can then be expressed as:
```
X[k] = Σ_{n=0}^{N/2-1} x[2n]e^(-j2πkn/N) + Σ_{n=0}^{N/2-1} x[2n+1]e^(-j2πkn/N)
```
Using Euler's formula, the exponential terms can be simplified to:
```
X[k] = Σ_{n=0}^{N/2-1} x[2n]cos(2πkn/N) - jΣ_{n=0}^{N/2-1} x[2n]sin(2πkn/N) + Σ_{n=0}^{N/2-1} x[2n+1]cos(2πkn/N) - jΣ_{n=0}^{N/2-1} x[2n+1]sin(2πkn/N)
```
Let the DFTs of the even and odd parts be X_e[k] and X_o[k], respectively. Then we have:
```
X[k] = X_e[k] + e^(-j2πk/N)X_o[k]
```
Thus, an N-point DFT is decomposed into two N/2-point DFTs.
#### 2.2.2 Implementation of FFT Algorithm
The implementation of the FFT algorithm typically employs the divide and conquer strategy, recursively breaking down the DFT into smaller DFTs. The specific steps are as follows:
1. If the signal length is 1, compute the DFT directly.
2. Otherwise, ***
***pute the final DFT using the DFTs of the even and odd parts.
The complexity of the FFT algorithm is O(NlogN), which is a significant reduction from the complexity O(N^2) of direct DFT computation.
# 3.1 Introduction to MATLAB DSP Toolbox
#### 3.1.1 Functions and Applications of DSP Toolbox
The MATLAB DSP Toolbox is a powerful set of tools for digital signal processing (DSP) tasks. It provides a range of functions and tools for signal analysis, filtering, spectral analysis, and image processing.
The main functions of the DSP toolbox include:
- **Signal Generation and Processing:** Create and manipulate various types of signals, including sine waves, square waves, and noise.
- **Filtering:** Design and implement various types of filters, including low-pass, high-pass, and band-pass filters.
- **Spectral Analysis:** Calculate the amplitude spectrum and phase spectrum of signals to analyze their frequency components.
- **Image Processing:** Process and analyze images, including image enhancement, compression, and segmentation.
#### 3.1.2 Installation and Use of DSP Toolbox
The MATLAB DSP Toolbox can be installed as an add-on to MATLAB. The installation process is as follows:
1. Open MATLAB and go to the "Add-Ons" tab.
2. In the "Add-Ons Manager," search for "DSP Toolbox."
3. Click the "Install" button.
Once installed, the DSP Toolbox can be loaded into MATLAB by typing the following command in the MATLAB command window:
```matlab
addpath(genpath('path/to/DSP_toolbox_folder'))
```
### 3.2 FFT Simulation Implementation
#### 3.2.1 MATLAB Implementation of FFT Algorithm
MATLAB provides the `fft` function to perform the FFT algorithm. The syntax is as follows:
```matlab
Y = fft(x)
```
Where:
- `x` is the input signal (time-domain signal).
- `Y` is the output signal (frequency-domain signal).
The following code example demonstrates how to use the `fft` function to execute an FFT:
```matlab
x = [1, 2, 3, 4, 5, 6, 7, 8];
Y = fft(x);
% Calculate the magnitude spectrum
magnitude_spectrum = abs(Y);
% Calculate the phase spectrum
phase_spectrum = angle(Y);
% Plot the magnitude spectrum and phase spectrum
figure;
subplot(2, 1, 1);
plot(magnitude_spectrum);
title('Magnitude Spectrum');
xlabel('Frequency');
ylabel('Magnitude');
subplot(2, 1, 2);
plot(phase_spectrum);
title('Phase Spectrum');
xlabel('Frequency');
ylabel('Phase');
```
#### 3.2.2 Analysis of FFT Simulation Results
The results of the FFT simulation include the magnitude spectrum and phase spectrum. The magnitude spectrum represents the amplitude of the signal at different frequencies, while the phase spectrum represents the phase at different frequencies.
The magnitude spectrum can be used to identify the frequency components in the signal. In the example above, the magnitude spectrum shows two main frequency components in the signal: one at a low frequency and another at a high frequency.
The phase spectrum can be used to analyze the phase relationship of the signal. In the example above, the phase spectrum shows that the signal's phase is zero at low frequencies and π at high frequencies.
# 4. FFT Application Examples
### 4.1 Signal Processing
FFT has a wide range of applications in the field of signal processing, including spectral analysis and filtering.
#### 4.1.1 Spectral Analysis
Spectral analysis is the process of decomposing a signal into its constituent frequency components. FFT can compute the spectrum of a signal quickly and efficiently, aiding in the analysis of the signal's frequency characteristics.
For instance, in speech signal processing, FFT can be used to analyze the frequency components of speech signals to identify the vocal characteristics of the speaker.
#### 4.1.2 Filtering
Filtering is the process of removing unwanted frequency components from a signal. FFT can be used to design filters, allowing for the filtering operation of signals.
For example, in image processing, FFT can be used to design high-pass filters to remove noise from images.
### 4.2 Image Processing
FFT also plays a significant role in image processing, including image enhancement and compression.
#### 4.2.1 Image Enhancement
Image enhancement is the process of improving image quality. FFT can be used to enhance contrast, brightness, and clarity of images.
For instance, FFT can be used to design sharpening filters to increase image clarity.
#### 4.2.2 Image Compression
Image compression is the process of reducing the size of image files. FFT can be used to design image compression algorithms for lossless or lossy compression of images.
For example, the JPEG image compression algorithm is based on FFT.
# 5. FFT Optimization Techniques
### 5.1 Algorithm Optimization
#### 5.1.1 Radix-2 FFT Algorithm
The Radix-2 FFT algorithm is a variant of the FFT algorithm that decomposes the computation of the DFT into a series of smaller 2-point DFT computations. This decomposition can significantly reduce the computational load, especially when the input data length is a power of two.
**Algorithm Steps:**
1. Decompose the input data into two sub-sequences with even and odd indices.
2. Perform 2-point DFT computations on each sub-sequence.
3. Merge the DFT results of the two sub-sequences to obtain the final DFT result.
**Code Block:**
```python
def radix2_fft(x):
"""
Radix-2 FFT algorithm
Parameters:
x: Input data sequence
Returns:
X: DFT result
"""
N = len(x)
if N == 1:
return x
# Decompose input data
x_even = x[::2]
x_odd = x[1::2]
# Compute sub-sequence DFTs
X_even = radix2_fft(x_even)
X_odd = radix2_fft(x_odd)
# Merge DFT results
X = np.zeros(N, dtype=***plex128)
for k in range(N // 2):
X[k] = X_even[k] + np.exp(-1j * 2 * np.pi * k / N) * X_odd[k]
X[k + N // 2] = X_even[k] - np.exp(-1j * 2 * np.pi * k / N) * X_odd[k]
return X
```
**Logical Analysis:**
This code implements the Radix-2 FFT algorithm. It first decomposes the input data into two sub-sequences with even and odd indices, then computes the DFTs for each sub-sequence. Finally, it merges the DFT results of the two sub-sequences to obtain the final DFT result.
#### 5.1.2 Radix-4 FFT Algorithm
The Radix-4 FFT algorithm is an extension of the Radix-2 FFT algorithm, decomposing the DFT computation into a series of smaller 4-point DFT computations. This decomposition further reduces the computational load, especially when the input data length is a power of four.
**Algorithm Steps:**
1. Decompose the input data into four sub-sequences, ***
***pute 4-point DFTs for each sub-sequence.
3. Merge the DFT results of the four sub-sequences to obtain the final DFT result.
**Code Block:**
```python
def radix4_fft(x):
"""
Radix-4 FFT algorithm
Parameters:
x: Input data sequence
Returns:
X: DFT result
"""
N = len(x)
if N == 1:
return x
# Decompose input data
x_0 = x[::4]
x_1 = x[1::4]
x_2 = x[2::4]
x_3 = x[3::4]
# Compute sub-sequence DFTs
X_0 = radix4_fft(x_0)
X_1 = radix4_fft(x_1)
X_2 = radix4_fft(x_2)
X_3 = radix4_fft(x_3)
# Merge DFT results
X = np.zeros(N, dtype=***plex128)
for k in range(N // 4):
X[k] = X_0[k] + np.exp(-1j * 2 * np.pi * k / N) * X_1[k] + np.exp(-1j * 4 * np.pi * k / N) * X_2[k] + np.exp(-1j * 6 * np.pi * k / N) * X_3[k]
X[k + N // 4] = X_0[k] - np.exp(-1j * 2 * np.pi * k / N) * X_1[k] + np.exp(-1j * 4 * np.pi * k / N) * X_2[k] - np.exp(-1j * 6 * np.pi * k / N) * X_3[k]
X[k + N // 2] = X_0[k] + np.exp(-1j * 2 * np.pi * k / N) * X_1[k] - np.exp(-1j * 4 * np.pi * k / N) * X_2[k] + np.exp(-1j * 6 * np.pi * k / N) * X_3[k]
X[k + 3 * N // 4] = X_0[k] - np.exp(-1j * 2 * np.pi * k / N) * X_1[k] - np.exp(-1j * 4 * np.pi * k / N) * X_2[k] - np.exp(-1j * 6 * np.pi * k / N) * X_3[k]
return X
```
**Logical Analysis:**
This code implements the Radix-4 FFT algorithm. It first decomposes the input data into four sub-sequences, each containing four adjacent elements. Then it computes 4-point DFTs for each sub-sequence. Finally, it merges the DFT results of the four sub-sequences to obtain the final DFT result.
### 5.2 Parallelization Techniques
#### 5.2.1 Multithreading Parallelization
Multithreading parallelization is a technique that improves computational performance by executing tasks simultaneously using multiple threads. It can decompose the FFT algorithm into multiple smaller tasks and assign them to different threads for execution.
**Code Block:**
```python
import threading
def fft_thread(x, start, end):
"""
FFT thread function
Parameters:
x: Input data sequence
start: Thread start index
end: Thread end index
"""
X = np.fft.fft(x[start:end])
return X
def fft_multithread(x, num_threads):
"""
Multithreaded FFT algorithm
Parameters:
x: Input data sequence
num_threads: Number of threads
Returns:
X: DFT result
"""
N = len(x)
threads = []
step = N // num_threads
for i in range(num_threads):
start = i * step
end = (i + 1) * step
thread = threading.Thread(target=fft_thread, args=(x, start, end))
threads.append(thread)
for thread in threads:
thread.start()
for thread in threads:
thread.join()
X = np.concatenate([thread.result for thread in threads])
return X
```
**Logical Analysis:**
This code implements the multithreaded FFT algorithm. It first decomposes the input data into multiple smaller tasks and assigns them to different threads for execution. Then it merges the results of each thread into the final DFT result.
#### 5.2.2 GPU Parallelization
GPU parallelization is a technique that utilizes graphics processing units (GPUs) to improve computational performance. GPUs have a large number of parallel processing units and can perform a vast number of computations simultaneously. It can decompose the FFT algorithm into a large number of smaller tasks and assign them to the GPU for execution.
**Code Block:**
```python
import cupy
def fft_gpu(x):
"""
GPU FFT algorithm
Parameters:
x: Input data sequence
Returns:
X: DFT result
"""
X = cupy.fft.fft(x)
return X.get()
```
**Logical Analysis:**
This code implements the GPU FFT algorithm. It first copies the input data into the GPU memory, then uses the GPU to perform the FFT calculation. Finally, it copies the results back into the CPU memory.
# 6.1 Quantum FFT Algorithm
**6.1.1 Principles of Quantum FFT Algorithm**
The Quantum Fast Fourier Transform (QFFT) ***pared to classical FFT algorithms, the QFFT has the following advantages:
- **Exponential Acceleration:** The complexity of the QFFT algorithm is O(n log n), while the complexity of classical FFT algorithms is O(n^2). For large datasets, the QFFT algorithm can provide significant acceleration.
- **Parallel Computation:** The QFFT algorithm can leverage the parallelism of quantum bits to perform multiple Fourier transforms simultaneously.
The basic principle of the QFFT algorithm is to utilize the superposition and interference properties of quantum states. Specifically, the algorithm represents the input data as a quantum state and then transforms the quantum state through a series of quantum gate operations to ultimately obtain the result of the Fourier transform.
**6.1.2 Applications of Quantum FFT Algorithm**
The QFFT algorithm has a broad application prospect in the following fields:
- **Quantum Computing:** The QFFT algorithm is an important foundational algorithm in quantum computing, applicable for solving various quantum computing problems.
- **Signal Processing:** The QFFT algorithm can accelerate signal processing tasks, such as spectral analysis and filtering.
- **Image Processing:** The QFFT algorithm can be used for image processing tasks, such as image enhancement and compression.
- **Financial Modeling:** The QFFT algorithm can accelerate Fourier transform computations involved in financial modeling.
- **Cryptography:** The QFFT algorithm can be used to crack Fourier transform-based cryptography algorithms.
0
0
相关推荐
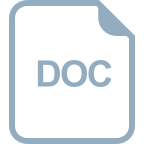
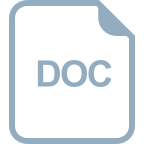
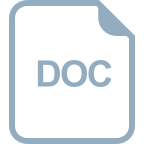
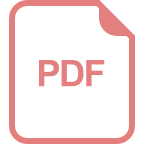
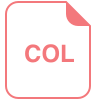
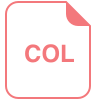
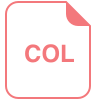
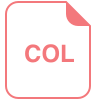
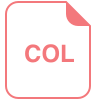