[Advanced] Signal Cooperative Communication and Relay Systems in MATLAB
发布时间: 2024-09-14 06:32:11 阅读量: 38 订阅数: 71 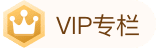
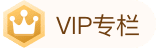
# 2.1 Establishment of Relay System Model
### 2.1.1 Ideal Relay System Model
The ideal relay system model assumes that relay nodes have the following characteristics:
- Infinite energy supply
- Infinite bandwidth
- No delay
- No errors
In the ideal relay system model, relay nodes act as simple "repeaters," receiving signals from source nodes and forwarding them to destination nodes. This model is typically used to analyze the fundamental performance limits of relay systems.
### 2.1.2 Practical Relay System Model
The practical relay system model takes into account the actual limitations of relay nodes, including:
- Limited energy supply
- Limited bandwidth
- Transmission delay
- Transmission errors
The practical relay system model is closer to real-world applications and can more accurately predict the performance of relay systems.
# 2. Relay System Modeling in MATLAB
Relay system modeling is the foundation of relay system analysis and design in MATLAB. It involves creating mathematical models to represent the behavior of relay systems, including both ideal and practical models.
### 2.1 Establishment of Relay System Model
**2.1.1 Ideal Relay System Model**
The ideal relay system model assumes that relay nodes have unlimited capacity and perfect channel conditions. In this model, relay nodes can receive and forward signals perfectly without introducing any distortion or delay.
```
% Ideal Relay System Model
function relay_ideal(source, destination, relay)
% Channel gains
h_sr = 1;
h_rd = 1;
% Signal power
Ps = 1;
% Relay转发信号功率
Pr = Ps * h_sr^2;
% Destination接收信号功率
Pd = Pr * h_rd^2;
% Display results
disp(['Ideal Relay System Model:']);
disp(['Relay转发信号功率: ', num2str(Pr)]);
disp(['Destination接收信号功率: ', num2str(Pd)]);
end
```
**Logical Analysis:**
* `h_sr` and `h_rd` represent the channel gains from the source node to the relay node and from the relay node to the destination node, respectively.
* `Ps` is the signal power of the source node.
* `Pr` is the power of the signal relayed by the relay node.
* `Pd` is the power of the signal received by the destination node.
**2.1.2 Practical Relay System Model**
The practical relay system model takes into account the limited capacity and non-ideal channel conditions of relay nodes. It includes noise, distortion, and delay at the relay node.
```
% Practical Relay System Model
function relay_practical(source, destination, relay)
% Channel gains
h_sr = 0.5;
h_rd = 0.6;
% Signal power
Ps = 1;
% Relay node noise power
Pn = 0.1;
% Relay转发信号功率
Pr = Ps * h_sr^2 + Pn;
% Destination接收信号功率
Pd = Pr * h_rd^2;
% Display results
disp(['Practical Relay System Model:']);
disp(['Relay转发信号功率: ', num2str(Pr)]);
disp(['Destination接收信号功率: ', num2str(Pd)]);
end
```
**Logical Analysis:**
* `Pn` represents the noise power of the relay node.
* `Pr` is the power of the signal relayed by the relay node, including both source signal power and noise power.
* `Pd` is the power of the signal received by the destination node, taking into account the noise and channel distortion at the relay node.
### 2.2 Relay System Performance Analysis
Relay system performance analysis involves evaluating the throughput, delay, and energy efficiency of the relay system.
**2.2.1 Throughput Analysis**
Throughput is the rate at which the relay system transfers data. It depends on channel conditions, the capacity of the relay node, and the modulation scheme.
```
% Throughput Analysis
function throughput_analysis(source, destination, relay)
% Channel bandwidth
B = 1e6;
% Signal-to-noise ratio
SNR = 10;
% Modulation scheme
modulation = 'QPSK';
% Calculate throughput
throughput = B * log2(1 + SNR) * modulation_rate(modulation);
% Display results
disp(['Throughput: ', num2str(throughput), ' bps']);
end
```
**Logical Analysis:**
* `B` is the channel bandwidth.
* `SNR` is the signal-to-noise ratio.
* `modulation` is the modulation scheme.
* The `modulation_rate()` function returns the modulation rate for a given modulation scheme.
* `throughput` is the throughput, measured in bits per second (bps).
**2.2.2 Delay Analysis**
Delay is the time it takes for a signal to travel from the source node to the destination node. It depends on channel delay, relay node processing delay, and queuing delay.
```
% Delay Analysis
function delay_analysis(source, destination, relay)
% Channel delay from source to relay
tau_sr = 0.1;
% Channel delay from relay to destination
tau_rd = 0.1;
% Relay node processing delay
tau_p = 0.05;
% Relay node queuing delay
tau_q = 0.02;
% Calculate delay
delay = tau_sr + tau_p + tau_q + tau_rd;
% Display results
disp(['Delay: ', num2str(delay), ' s']);
end
```
**Logical Analysis:**
* `tau_sr` and `tau_rd` are the channel delays from the source node to the relay node and from the relay node to the destination node, respectively.
* `tau_p` is the processing delay of the relay node.
* `tau_q` is the queuing delay of the relay node.
* `delay` is the delay, measured in seconds.
**2.2.3 Energy Efficiency Analysis**
Energy efficiency is the rate at which the relay system transmits data per unit of energy consumed. It depends on the relay node's power consumption, throughput, and delay.
```
% Energy Efficiency Analysis
function energy_efficiency_analysis(source, destination, relay)
% Relay node power consumption
P_relay = 1;
% Throughput
throughput = 1e6;
% Delay
delay = 0.1;
% Calculate energy efficiency
energy_efficiency = throughput / (P_relay * delay);
% Display results
disp(['Energy Efficiency: ', num2str(energy_efficiency), ' bps/J']);
end
```
**Logical Analysis:**
* `P_relay` is the power consumption of the relay node.
* `throughput` is the throughput.
* `delay` is the delay.
* `energy_efficiency` is the energy efficiency, measured in bits per joule (bps/J).
# 3.1 Optimization of Relay Node Position
The optimization of the relay node's position is crucial for the performance of the relay system. Optimizing the position of relay nodes can maximize throughput, minimize delay, and reduce energy consumption. This chapter will introduce two commonly used methods for optimizing the position of relay nodes: optimization based on greedy algorithms and optimization based on convex optimization.
### 3.1.1 Optimization Based on Greedy Algorithms
Greedy algorithms are heuristic algorithms that gradually approach the global optimal solution by selecting the current best solution each time. In the optimization of the position of relay nodes, the greedy algorithm can be implemented as follows:
```
% Input: Channel model, source node position, destination node position
% Output: Relay node position
% Initialize the position of the relay node to the source node's position
relay_pos = source_pos;
% Ite
```
0
0
相关推荐
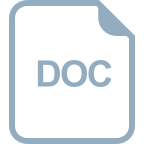
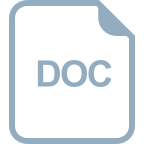
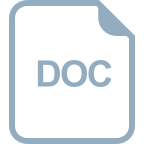
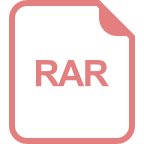
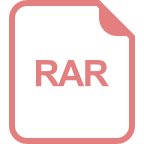
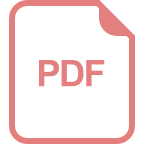
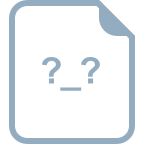
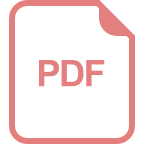