【Practical Exercise】Implementation of a Basic Voice Signal Recognition System in MATLAB
发布时间: 2024-09-14 06:53:45 阅读量: 52 订阅数: 71 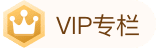
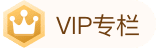
# 1. Basic Principles of MATLAB Speech Signal Processing
Speech signal processing is a technology that uses computer technology to analyze, process, and recognize speech signals. MATLAB, as a powerful scientific computing software, offers a wealth of toolboxes for speech signal processing, which can efficiently accomplish various tasks related to speech signals. This chapter will introduce the fundamental knowledge of MATLAB speech signal processing, including the sampling, quantization, noise reduction, feature extraction, and classification of speech signals.
# 2. Speech Signal Preprocessing
Speech signal preprocessing is a crucial step in speech signal processing, aimed at removing noise, enhancing signals, and preparing for subsequent feature extraction and classification.
### 2.1 Sampling and Quantization of Speech Signals
#### 2.1.1 Sampling Theorem and Sampling Frequency
The sampling theorem states that to avoid aliasing, the sampling frequency of the speech signal must be at least twice the highest frequency of the signal. The sampling frequency determines the spectral resolution and temporal resolution of the signal.
#### 2.1.2 Quantization and Quantization Error
Quantization is the process of converting a continuous analog signal into a discrete digital signal. Quantization error is the difference between the quantized signal and the original signal, which affects the quality of the signal.
```matlab
% A speech signal with a sampling frequency of 8000 Hz
fs = 8000;
% Sampling signal
speech_signal = audioread('speech.wav');
% Quantization bits of 8
num_bits = 8;
% Quantized signal
quantized_signal = quantize(speech_signal, num_bits);
% Calculate quantization error
quantization_error = speech_signal - quantized_signal;
% Plot original signal, quantized signal, and quantization error
figure;
subplot(3,1,1);
plot(speech_signal);
title('Original Signal');
subplot(3,1,2);
plot(quantized_signal);
title('Quantized Signal');
subplot(3,1,3);
plot(quantization_error);
title('Quantization Error');
```
### 2.2 Denoising of Speech Signals
#### 2.2.1 Time-domain Filtering and Frequency-domain Filtering
Time-domain filtering processes the signal's time series directly, such as moving average filters and median filters. Frequency-domain filtering converts the signal into the frequency domain and then filters specific frequency ranges, such as low-pass filters and high-pass filters.
#### 2.2.2 Adaptive Filtering and Wiener Filtering
Adaptive filters can automatically adjust filter parameters based on the statistical characteristics of the input signal to suppress noise. The Wiener filter is a type of adaptive filter that minimizes the mean square error between the signal and noise.
```matlab
% Original speech signal
speech_signal = audioread('speech.wav');
% Adding noise
noise_signal = randn(size(speech_signal));
noisy_signal = speech_signal + noise_signal;
% Time-domain filtering: Moving average filter
window_size = 101;
filtered_signal_time = movmean(noisy_signal, window_size);
% Frequency-domain filtering: Low-pass filter
cutoff_frequency = 3000;
order = 6;
filtered_signal_freq = lowpass(noisy_signal, cutoff_frequency, fs, order);
% Adaptive filtering: LMS algorithm
step_size = 0.001;
num_iterations = 1000;
[~, filtered_signal_lms] = lms(noisy_signal, speech_signal, step_size, num_iterations);
% Wiener filtering
filtered_signal_wiener = wiener(noisy_signal, speech_signal);
% Plot original signal, noise signal, and filtered signals
figure;
subplot(5,1,1);
plot(speech_signal);
title('Original Signal');
subplot(5,1,2);
plot(noisy_signal);
title('Noisy Signal');
subplot(5,1,3);
plot(filtered_signal_time);
title('Time-domain Filtering');
subplot(5,1,4);
plot(filtered_signal_freq);
title('Frequency-domain Filtering');
subplot(5,1,5);
plot(filtered_signal_lms);
title('Adaptive Filtering');
subplot(5,1,6);
plot(filtered_signal_wiener);
title('Wiener Filtering');
```
# 3. Feature Extraction of Speech Signals
Feature extraction of speech signals
0
0
相关推荐
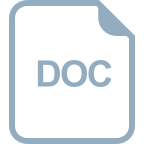
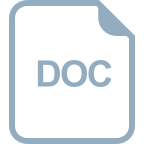
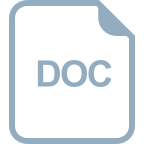
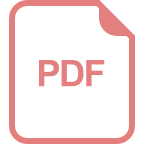
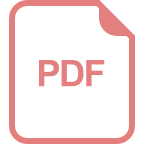
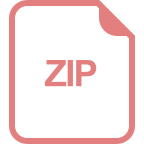
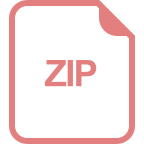
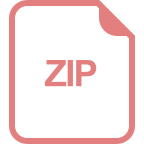