Selection and Optimization of Anomaly Detection Models: 4 Tips to Ensure Your Model Is Smarter
发布时间: 2024-09-15 11:48:09 阅读量: 10 订阅数: 17 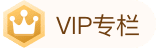
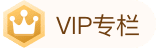
# 1. Overview of Anomaly Detection Models
## 1.1 Introduction to Anomaly Detection
Anomaly detection is a significant part of data science that primarily aims to identify anomalies—data points that deviate from expected patterns or behaviors—from vast amounts of data. These anomalies might represent errors, fraud, system failures, or other conditions that warrant special attention.
## 1.2 Application Scenarios
Anomaly detection technology is applied in various fields, such as credit card fraud detection, network security intrusion detection, and the identification of rare diseases in medical diagnoses. It aids businesses in discovering potential risks in a timely manner and responding accordingly.
## 1.3 Basic Workflow of the Model
The basic workflow of anomaly detection models typically includes data collection, preprocessing, feature extraction, model selection, training and evaluation, and the final model deployment and monitoring. Each step is designed to enhance the accuracy and efficiency of the model in real-world scenarios.
# 2. Theoretical Foundation of Model Selection
## 2.1 Types of Anomaly Detection Models
### 2.1.1 Statistical Methods
Statistical methods form the foundation of anomaly detection, ***mon approaches are parametric and non-parametric methods.
**Parametric methods** assume that data follows a specific distribution, such as the Gaussian distribution, and use model parameters to describe this distribution. For instance, if we assume data follows a Gaussian distribution, we can calculate the mean and variance, and set thresholds based on these parameters. Any data points beyond these thresholds may be considered anomalous. This method performs well when the data distribution is known and stable.
```python
import numpy as np
from scipy import stats
# Assuming we have normally distributed data
data = np.random.randn(1000)
# Calculate mean and standard deviation
mean, std = data.mean(), data.std()
# Set a threshold: typically, a range of standard deviations
threshold = 3 * std
# Find outliers
outliers = data[(np.abs(data - mean) > threshold)]
print("Number of outliers:", len(outliers))
```
**Non-parametric methods** do not rely on data's parameter models but directly analyze the data. For example, the k-nearest neighbors (k-NN) method can detect anomalies based on the assumption that data points in high-density areas are normal, whereas those in low-density areas may be anomalous. The algorithm calculates the distance from a point to its k nearest neighbors and considers the point to be anomalous if this distance exceeds a certain threshold.
```python
from sklearn.neighbors import NearestNeighbors
# Using k-NN to detect anomalies
model = NearestNeighbors(n_neighbors=5)
model.fit(data.reshape(-1, 1))
distances, indices = model.kneighbors(data.reshape(-1, 1))
# Find outliers: those beyond twice the average distance may be anomalous
mean_dist = distances.mean(axis=1)
outliers = data[mean_dist > 2 * mean_dist.mean()]
print("Number of outliers:", len(outliers))
```
### 2.1.2 Machine Learning Methods
Compared to statistical methods, machine learning methods do not req***mon machine learning methods include Support Vector Machines (SVM), Isolation Forest, and neural network-based methods.
**Support Vector Machines (SVM)** can be used for anomaly detection by constructing a hyperplane that separates normal data from anomalies. SVM builds this hyperplane by maximizing the margin between normal and anomalous data. After training, any point on the other side of the hyperplane can be considered an anomaly.
```python
from sklearn.svm import OneClassSVM
# Using One-Class SVM for anomaly detection
svm = OneClassSVM(kernel="rbf", nu=0.05)
svm.fit(data.reshape(-1, 1))
# Predict anomalies
outliers = svm.predict(data.reshape(-1, 1)) == -1
print("Number of outliers:", sum(outliers))
```
**Isolation Forest** is a tree-based algorithm that randomly selects features and randomly chooses split values to "isolate" sample points. Since anomalies are sparse and differ significantly from other data points, they are typically isolated earlier in the decision tree.
```python
from sklearn.ensemble import IsolationForest
# Using Isolation Forest for anomaly detection
iso_forest = IsolationForest(contamination=0.05)
outliers = iso_forest.fit_predict(data.reshape(-1, 1))
# Find outliers
print("Number of outliers:", sum(outliers == -1))
```
## 2.2 Model Evaluation Criteria
### 2.2.1 Accuracy Me***
***mon accuracy metrics include Precision, Recall, and the F1 score.
- **Precision** refers to the proportion of actual anomalies among the data points predicted as anomalous by the model. It indicates the model's ability to accurately predict anomalies in marked abnormal data.
- **Recall** refers to the proportion of all actual anomalies that the model successfully identifies. It reflects the model's ability to detect anomalies.
- The **F1 score** is the harmonic mean of Precision and Recall, serving as a measure of the overall model performance.
```python
from sklearn.metrics import precision_score, recall_score, f1_score
# Assuming we have actual and predicted values
true_values = np.array([1, 0, 1, 1, 0, 0, 1])
predicted_values = np.array([1, 0, 0, 1, 0, 1, 0])
# Calculate accuracy metrics
precision = precision_score(true_values, predicted_values)
recall = recall_score(true_values, predicted_values)
f1 = f1_score(true_values, predicted_values)
print(f"Precision: {precision}, Recall: {recall}, F1 score: {f1}")
```
### 2.2.2 Predictive Quality Metrics
In addition to accuracy metrics, there are other indicators used to assess the quality of a model's predictions. For example, ROC-AUC (Receiver Operating Characteristic - Area Under Curve) is widely used in classification problems and is particularly suitable for imbalanced datasets.
- **ROC-AUC** is assessed by calculating the area under the ROC curve, which evaluates the model's performance at different thresholds. An ideal model's ROC curve is close to the top left corner, indicating high recall and high precision.
```python
from sklearn.metrics import roc_auc_score
# Assuming we have actual and predicted probabilities
true_values = np.array([1, 0, 1, 1, 0, 0, 1])
predicted_probabilities = np.array([0.9, 0.1, 0.8, 0.65, 0.1, 0.2, 0.3])
# Calculate ROC-AUC
roc_auc = roc_auc_score(true_values, predicted_probabilities)
print(f"ROC-AUC: {roc_auc}")
```
## 2.3 Influencing Factors for Model Selection
### 2.3.1 Data Characteristic Analysis
Before selecting an appropriate anomaly detection model, a thorough analysis of the data is necessary. Data characteristics include the dimensionality, distribution, noise level, and presence of missing values.
- **Data Dimensionality**: High dimensionality may result in sparsity, which can make distance-based methods (like k-NN) less effective. In high-dimensional data, dimensionality reduction techniques like PCA can be considered, or algorithms capable of handling high-dimensional data, such as the Isolation Forest, can be used.
- **Data Distribution**: Some algorithms assume a specific data distribution, such as the Gaussian distribution. If the data does not follow such a distribution, the performance of these algorithms may degrade.
- **Noise Level**: In the presence of significant noise, statistical models may not be suitable as noise can interfere with the model's judgment on anomalies. In such cases, machine learning methods may be needed.
- **Missing Values**: Missing values can be handled in various ways, such as filling (interpolation), ignoring, or using robust model versions.
### 2.3.2 Considerations for Real-world Application Scenarios
In addition to data characteristics, the requirements and constraints of real-world application scenarios are crucial for model selection. These requirements include model real-time performance, interpretability, complexity, and deployment environment.
- **Real-time Performance**: For applications that require real-time or near-real-time detection (such as credit card fraud detection), model selection must consider computational efficiency. It may be necessary to sacrifice some accuracy to ensure detection speed.
- **Interpretability**: In some fields (like medical diagnostics), model interpretability is equally important. Statistical methods and tree-based machine learning methods are typically easier to interpret.
- **Complexity**: Simple models are easier to understand and deploy but may not handle complex data structures. More complex models may offer better performance but increase computational costs and maintenance difficulties.
- **Deployment Environment**: The model deployment environment also influences model selection, such as whether a GPU can be used, or the model needs to run on edge devices.
These factors should be considered comprehensively when selecting an anomaly detection model. In practice, it may be necessary to experiment with various models and use techniques like cross-validation to evaluate model performance and ultimately select the model that best suits the application requirements.
# 3. Model Optimization Techniques in Practice
## 3.1 Feature Engineering
### 3.1.1 Feature Selection Methods
Feature selection is an important step in reducing model complexity, improving model running efficiency, and avoiding overfitting. ***mon feature selection methods include:
- Filter Methods: Select features through statistical tests without considering model performance. Typical methods include the chi-squared test, mutual information, and analysis of variance (ANOVA).
- Wrapper Methods: Use a learner to evaluate the effect of feature subsets, such as Recursive Feature Elimination (RFE).
- Embedded Methods: Perform feature selection during the learner training process, such as Lasso and Ridge Regression.
Each method corresponds to different scenarios and needs. Choosing the appropriate feature selection method can significantly enhance model performance. When dealing with large datasets, Wrapper and Embedded methods may increase computational costs, while Filter methods are more efficient.
**Code Example**: Using Recursive Feature Elimination (RFE) for feature selection.
```python
from sklearn.feature_selection import RFE
from sklearn.ensemble import RandomForestClassifier
```
0
0
相关推荐
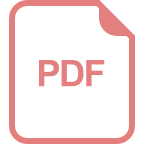
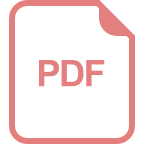
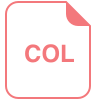
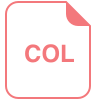
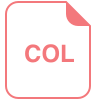
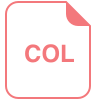


