The Absolute Importance of Model Validation: How to Ensure Your Model Isn't a House of Cards
发布时间: 2024-09-15 11:10:29 阅读量: 27 订阅数: 32 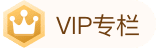
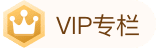
# The Absolute Importance of Model Validation: Ensuring Your Model Isn't a Hollow Skyscraper
Model validation is a core step in the field of data science to ensure the quality of a model. It is crucial for improving the predictive accuracy of models and guaranteeing their effectiveness and reliability in real-world applications. The validation process helps us identify and correct model biases, assess the generalization ability of models, and provide data support for model selection. Therefore, whether in academic research or actual business applications, model validation plays an indispensable role.
Next, we will delve into the theoretical framework of model validation, including its basic concepts, methodological validation, and the decomposition and analysis of model errors. These contents provide us with the necessary theoretical basis for in-depth understanding and implementation of model validation.
# The Theoretical Framework of Model Validation
## Basic Concepts of Model Validation
### Definition and Objectives
Model validation is a core link in the field of data analysis and machine learning, ensuring the reliability and effectiveness of a model in practical applications. In definition, model validation refers to the process of evaluating the predictive accuracy of a model, ensuring that the model's performance on unknown data meets expectations. The goal is to identify and minimize prediction errors, including bias and variance.
The practical objectives of model validation are multifaceted:
1. **Accuracy assessment**: Determine whether the model's predictive performance meets business or research standards.
2. **Robustness testing**: Test whether the model's performance is stable across different datasets.
3. **Bias analysis**: Identify and reduce systematic errors introduced during data collection, processing, or model training.
To achieve these goals, model validation needs to consider a variety of evaluation methods and techniques, including but not limited to cross-validation, bootstrapping, and error analysis.
### Importance of Validation
The importance of model validation cannot be underestimated, especially in areas requiring highly accurate predictions, such as finance, healthcare, and security. The validation process provides a guarantee for the reliability and applicability of the model in the following ways:
1. **Improving predictive accuracy**: By evaluating model performance on an independent test dataset, we can identify whether the model is overfitting the training dataset, thereby enhancing the model's generalization ability.
2. **Ensuring the credibility of results**: Users or decision-makers typically need to establish trust in the model's predictions through model validation.
3. **Identifying problems and directions for improvement**: The validation process reveals potential issues with the model, such as overfitting or underfitting, and through error analysis, it points out directions for improvement.
Model validation is an indispensable part of the model development process for data scientists and machine learning engineers. It helps optimize model performance and provides a solid foundation for model deployment and application.
## Methodology of Validation
### Statistical Hypothesis Testing
Statistical hypothesis testing is a fundamental tool in model validation, involving statistical inference on model performance. In statistics, a hypothesis test usually includes the following steps:
1. **Define hypotheses**: Clearly state the null hypothesis (H0) and the alternative hypothesis (H1). For example, in model validation, the null hypothesis might be that the model has no predictive error.
2. **Choose a test statistic**: Select an appropriate statistic based on the nature of the data and the hypothesis, such as the t-statistic or the chi-squared statistic.
3. **Determine the significance level**: Set a threshold (α), usually 0.05 or 0.01, to determine whether to reject or accept the null hypothesis.
4. **Calculate the test statistic value**: Use statistical methods and data to calculate the observed value of the test statistic.
5. **Draw conclusions**: Based on the comparison of the observed value with the threshold, decide whether to reject the null hypothesis.
Through hypothesis testing, the statistical significance of model prediction errors can be quantified, thus deciding whether to accept the model's predictive performance.
### Cross-Validation and Bootstrapping
Cross-validation and bootstrapping are two commonly used techniques for estimating model performance and reducing the risk of overfitting:
1. **Cross-validation**: The most commonly used cross-validation technique is k-fold cross-validation. In k-fold cross-validation, the dataset is divided into k equal-sized subsets. The model is trained on k-1 subsets and validated on the remaining one subset. This process is repeated k times, each time using a different validation subset. The final performance evaluation is based on the average performance of the k validations. An example code is as follows:
```python
from sklearn.model_selection import cross_val_score
from sklearn.linear_model import LinearRegression
from sklearn.datasets import make_regression
# Create a regression dataset
X, y = make_regression(n_samples=100, n_features=20, noise=0.1)
# Perform 10-fold cross-validation with a linear regression model
linreg = LinearRegression()
scores = cross_val_score(linreg, X, y, cv=10)
print(f"Mean accuracy: {scores.mean()}")
```
2. **Bootstrapping**: Bootstrapping is a sampling method with replacement, used to generate multiple alternative samples from the original dataset. The model is trained on each alternative sample and then evaluated on an independent test set. This method can provide a stable estimate of model performance and help estimate the model's predictive uncertainty.
## Decomposition and Analysis of Model Errors
### Sources of Errors
Model errors can usually be divided into two main types: bias and variance. Understanding these two errors is crucial for designing an effective validation strategy.
- **Bias**: Refers to the average difference between the model's predicted values and the true values. High bias usually indicates that the model is too simple and fails to capture the key relationships in the data.
- **Variance**: Refers to the consistency of the model's predicted values across different training sets. High variance indicates that the model is too complex and overly sensitive to random fluctuations in the training data.
### The Trade-off Between Bias and Variance
When designing a model, a trade-off must be made between bias and variance, often referred to as the bias-variance trade-off. Both high bias or high variance can impair the model's predictive performance. In the model selection and adjustment process, a balance must be continuously sought between model complexity and stability.
In the trade-off process, the usual approach is:
1. **Reduce bias**: By increasing model complexity, such as using more features or increasing model parameters.
2. **Reduce variance**: By introducing regularization techniques, such as L1 or L2 penalty terms, or using ensemble methods, such as random forests or gradient boosting trees.
The analysis of bias and variance is instructive for model selection and optimization and is a key link in the model validation process.
In the next chapter, we will delve into the practical operations of model validation, how to apply the above theoretical framework to actual data and models, and challenges and strategies for addressing issues encountered in practical operations.
# Practical Operations of Model Validation
After understanding the theoretical foundations of model validation, applying these theories to practical operations is a crucial step. This chapter will explore in depth the practical methods of model validation, including data preprocessing and feature engineering, model training and selection, and how to handle practical issues during the validation process.
## Data Preprocessing and Feature Engineering
Data is the foundation for building models, and data preprocessing and feature engineering are key steps to ensure the effectiveness of models. In this section, we will delve into how to clean and process data and how to select and reduce dimensions of features to prepare for model training.
### Data Cleaning and Preprocessing Techniques
In machine learning practice, data is often not clean and neat. Data cleaning is the primary step in preprocessing, aimed at identifying and dealing with missing values, outliers, duplicate data, and other issues. Data cleaning techniques include, but are not limited to, filling in missing values, removing or interpolating outliers, and merging duplicate records.
A typical method for handling missing values is mean imputation, as shown in the code example below:
```python
import pandas as pd
from sklearn.impute import SimpleImputer
# Load the dataset
df = pd.read_csv('dataset.csv')
# Simple mean imputation
imputer = SimpleImputer(strategy='mean')
df['feature'] = imputer.fit_transform(df[['feature']])
```
For detecting and processing outliers, the boxplot method can be used to identify outliers, and then decide whether to remove or take other actions based on the specific situation.
Data normalization is also an important technique in preprocessing, ***mon normalization methods include min-max normalization and Z-score standardization.
```python
from sklearn.preprocessing import MinMaxScaler, StandardScaler
# Min-max normalization
min_max_scaler = MinMaxScaler()
df['feature'] = min_max_scaler.fit_transform(df[['feature']])
# Z-score standardization
z_score_scaler = StandardScaler()
df['feature'] = z_score_scaler.fit_transform(df[['feature']])
```
### Feature Selection and Dimensionality Reduction Methods
The purpose of feature selection is to choose the most representative subset of features from the original data to reduce the complexity of the model and avoid overfitting. Feature selection methods can be divided into filter, wrapper, and embedded methods.
Filter methods select features based on statistical relationships between features and the target variable, such as chi-square tests, mutual information methods, etc.
Wrapper methods train models using different subsets of features and score them using performance evaluation metrics.
```python
from sklearn.feature_selection import RFE
from sklearn.ensemble import RandomForestClassifier
# Use random forest as an estimator for recursive feature elimination
selector = RFE(estimator=RandomForestClassifier(), n_features_to_select=5)
selector = selector.fit(df.drop('target', axis=1), df['target'])
selected_columns = df.columns[selector.support_]
```
Embedded methods perform feature selection during the model training process, for example, L1 regularization can force coefficients to zero, thereby achieving feature selection.
Dimensionality reduction is another feature engineering method used to reduce high-dimensional data to a lower dimensional space for easier model learning. Principal Component Analysis (PCA) is one of the most commonly used dimensionality reduction techniques.
```python
from sklearn.decomposition import PCA
# Use PCA for data dimensionality reduction
pca = PCA(n_components=2)
df_reduced = pca.fit_transform(df.drop('target', axis=1))
```
Through the above preprocessing and feature engineering steps, we can improve the training efficiency and accuracy of the model. Next, we will discuss how to perform model training and selection, and potential practical issues encountered during the validation process.
## Model Training and Selection
Training models on prepared datasets is a core part of the machine learning process. This section will discuss how to choose appropriate evaluation metrics and strategies and methods for model selection.
### Choosing Appropriate Evaluation Metrics
Choosing evaluation metrics is one of the key decisions in the model training and validation process, depending on the specific type of problem. For classification problems, common evaluation metrics include accuracy, precision, recall, and F1 score. For regression problems, common evaluation metrics include Mean Squared Error (MSE), Root Mean Squared Error (RMSE), and R-squared (R²).
```python
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score, mean_squared_error, r2_score
# Evaluation metrics for classification problems
accuracy = accuracy_score(y_true, y_pred)
precision = precision_score(y_true, y_pred)
recall = recall_score(y_true, y_pred)
f1 = f1_score(y_true, y_pred)
# Evaluation metrics for regression problems
mse = mean_squared_error(y_true, y_pred)
r2 = r2_score(y_true, y_pred)
```
### Strategies and Methods for Model Selection
Model selection usually involves comparing the performance of different models to find the one that best suits the current problem. Cross-validation is an important strategy for model selection, which can prevent overfitting and provide a more stable performance evaluation.
```python
from sklearn.model_selection import cross_val_score
# Use cross-validation to evaluate model performance
cross_val_scores = cross_val_score(model, X, y, cv=5)
```
Model selection methods can be rule-based, such as selecting the model with the highest accuracy, or machine learning-based, such as grid search (GridSearchCV).
```python
from sklearn.model_selection import GridSearchCV
# Set model parameters
param_grid = {'n_estimators': [10, 50, 100], 'max_depth': [2, 4, 6]}
# Use grid search for model selection
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X, y)
best_model = grid_search.best_estimator_
```
By carefully choosing evaluation metrics and model selection strategies, we can ensure that the selected model best meets the problem requirements. During the model validation process, we will encounter some practical issues, such as overfitting and underfitting, and testing the model's generalization ability. We will discuss these issues in more detail in the next section.
## Practical Issues in the Validation Process
The validation process will encounter various practical issues, among which overfitting and underfitting are the most common. This subsection will discuss the causes, diagnosis, and solutions of these problems, as well as how to test the generalization ability of the model.
### Diagnosis of Overfitting and Underfitting
Overfitting and underfitting are common problems encountered during model training. Overfitting occurs when the model performs well on the training data but poorly on validation or test data; underfitting is when the model performs poorly on all data.
Diagnosis methods include:
- Using learning curves to observe how training and validation errors change as the number of training samples increases.
- Comparing the performance of the model on training data and validation data.
A simple example of a learning curve is as follows:
```python
from sklearn.model_selection import learning_curve
import matplotlib.pyplot as plt
train_sizes, train_scores, val_scores = learning_curve(
estimator=model,
X=X,
y=y,
train_sizes=np.linspace(0.1, 1.0, 10),
cv=5,
scoring='accuracy'
)
# Calculate the average error of training and validation
train_mean = np.mean(train_scores, axis=1)
val_mean = np.mean(val_scores, axis=1)
# Draw the learning curve
plt.plot(train_sizes, train_mean, label='Training score')
plt.plot(train_sizes, val_mean, label='Cross-validation score')
plt.xlabel('Training examples')
plt.ylabel('Score')
plt.legend(loc='best')
plt.show()
```
### Testing the Generalization Ability of the Model
The generalization ability of a model refers to its ability to handle unseen data. A common method for testing model generalization ability is to split the dataset into training sets, validation sets, and test sets. After the model training and validation phases, the test set is used to evaluate the model's final generalization ability.
```python
from sklearn.model_selection import train_test_split
# Split the dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train the model
model.fit(X_train, y_train)
# Use the test set to evaluate the model
test_score = model.score(X_test, y_test)
```
The practical operations of model validation are an important step to ensure the effectiveness of the model, including data preprocessing, feature engineering, model training and selection, and diagnostic methods for solving practical problems. Through the discussion in this chapter, we can obtain detailed guidance on applying theory to practice, laying a solid foundation for building efficient and accurate models.
# Advanced Model Validation Techniques
In the field of model validation, deepening and expanding techniques are key to maintaining its adaptability and effectiveness. This chapter will delve into complex scenarios in model validation, interpretability, and the latest advances.
## Complex Scenarios in Model Validation
Model validation techniques require special consideration and methods when dealing with specific types of data, especially time series data and imbalanced data in big data situations.
### Validation of Time Series Data
Time series data, due to its inherent temporal correlation, presents special requirements for validation. Correctly handling this dependency is crucial for ensuring the model's validity.
```python
# Python code example: Splitting and validating time series data
from sklearn.model_selection import TimeSeriesSplit
# Assuming X, y are time series data and target variables
tscv = TimeSeriesSplit(n_splits=5)
for train_index, test_index in tscv.split(X):
X_train, X_test = X[train_index], X[test_index]
y_train, y_test = y[train_index], y[test_index]
# Here, model training and evaluation are performed
```
### Validation of Big Data and Imbalanced Data
In the big data environment, validation work is often limited by computing resources and is often accompanied by imbalanced data problems, requiring special validation strategies.
```python
# Python code example: Using SMOTE for imbalanced data processing
from imblearn.over_sampling import SMOTE
smote = SMOTE()
X_train_sm, y_train_sm = smote.fit_resample(X_train, y_train)
# Using the processed data to train the model
```
## Model Interpretability and Validation
As machine learning models become more complex, understanding the model's decision-making process becomes increasingly important.
### Importance of Interpretability Models
Interpretability models not only help us understand the model's decisions but are also key to building trust in the model.
```python
# Python code example: Using LIME for model explanation
from lime import lime_tabular
explainer = lime_tabular.LimeTabularExplainer(
training_data=np.array(X_train),
feature_names=np.array(feature_names),
class_names=np.array(class_names),
mode="classification"
)
# Generate an explanation for a predicted sample
idx = 10 # Select a sample
exp = explainer.explain_instance(X_test[idx], classifier.predict_proba, num_features=10)
exp.show_in_notebook(show_all=False)
```
### Interpretability Methods and Tools
Currently, there are various tools and techniques to improve the transparency of models, such as LIME (Local Interpretable Model-agnostic Explanations) and SHAP (SHapley Additive exPlanations).
## Latest Advances in Model Validation
In the fields of deep learning and automation technology, model validation techniques are also advancing.
### Model Validation in Deep Learning
The complexity of deep learning makes validation more important and challenging. For example, evaluating the generalization ability of deep learning models requires special strategies.
### Automated Validation Frameworks and Tools
Automated validation frameworks such as Keras Tuner, Ray Tune, etc., have begun to support automated model validation processes.
```python
# Python code example: Using Keras Tuner for hyperparameter optimization
from kerastuner import HyperModel
class SimpleHyperModel(HyperModel):
def __init__(self, input_shape):
self.input_shape = input_shape
def build(self, hp):
model = keras.Sequential()
model.add(keras.layers.Flatten(input_shape=self.input_shape))
model.add(keras.layers.Dense(units=hp.Int('units', min_value=32, max_value=512, step=32), activation='relu'))
model.add(keras.layers.Dense(10, activation='softmax'))
***pile(optimizer=keras.optimizers.Adam(hp.Choice('learning_rate', [1e-2, 1e-3, 1e-4])),
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Define the hyperparameter search space and start the search
hypermodel = SimpleHyperModel(input_shape=(28 * 28,))
tuner = RandomSearch(
hypermodel,
objective='val_accuracy',
max_trials=5,
executions_per_trial=3,
directory='my_dir',
project_name='helloworld'
)
tuner.search(x_train, y_train, epochs=10, validation_data=(x_val, y_val))
```
In this chapter, we discussed techniques for validating models in complex scenarios, introduced tools and methods for model interpretability, and explored the latest advances in automated validation frameworks and tools. These contents constitute some of the most cutting-edge topics in the field of model validation, providing a foundation for further deepening and advancing model validation technologies.
# Case Studies and Future Outlook
## Classic Case Analysis
### Successful Model Validation Cases
In the IT industry, successful cases of model validation undoubtedly set benchmarks for the entire industry. Taking a classic example from the field of machine learning—Google's AlphaGo. AlphaGo made history in the world of Go, successfully defeating world champion Lee Sedol. In this case, model validation played a crucial role.
- **Preparation phase for validation:** During the training phase of AlphaGo, the team used a massive amount of Go game data to train the model. At the same time, they adjusted model parameters through simulated matches to ensure that the model could make correct judgments in the face of complex situations.
- **Validation strategy:** Cross-validation was used to evaluate the model's performance, ensuring the robustness of the results. Moreover, different validation sets were set at different stages to evaluate the model's generalization ability during the learning process.
- **Validation results:** AlphaGo was not only able to make correct predictions on training data but, more importantly, was able to make excellent decisions in situations it had never seen before. Its success proved that the model was not just "overfitting" to existing game data.
From this case, we can see that effective model validation can ensure the performance of AI models in the real world and push the boundaries of technology in various fields such as business and research.
### Lessons from Model Validation Failures
Behind successful cases, model validation failures also provide valuable lessons. A widely discussed example is the predictive analysis model adopted by the US Department of Veterans Affairs (VA) in 2015.
- **Lack of the validation process:** The VA's model attempted to predict the suicide risk of veterans, but in actual use, the model had not been thoroughly validated. Shortly after the model was deployed, it issued too many false alarms, resulting in staff being unable to effectively respond to real crises.
- **Root of the problem:** The model had not undergone appropriate validation to test its accuracy across different populations and environments. Additionally, the VA did not consider the operability and practicality of the model in actual operations.
- **Lesson learned:** This case emphasizes that validation is not only needed during the model development stage but also needs to be continued after the model is deployed. The real-world data and scenarios are much more complex than the idealized test environment.
This case tells us that in the model validation process, we need to focus not only on the technical performance of the model but also on its practical application issues. Ensure the comprehensiveness of the validation process to prevent significant deviations in actual applications.
## Future Trends in Model Validation
### Directions of Model Validation Technology Development
As technology advances, model validation technology is also making progress. Future development trends can be seen from the following directions:
- **Automated validation:** As models become increasingly complex, manual validation becomes increasingly impractical. The development of automated tools and frameworks will allow for rapid and accurate model validation, for example, using automated tests in continuous integration/continuous deployment (CI/CD) pipelines.
- **Interpretability and explainability:** The decision-making process of machine learning models is becoming more transparent. Interpretability tools, such as LIME and SHAP, will become more widespread, allowing users to understand model predictions.
### The Relationship Between Ethics, Law, and Validation
Model validation is not just a technical issue; it also involves ethical and legal considerations. As artificial intelligence technology becomes more widespread, there will be increasing demands for transparency and explainability in its decision-making process.
- **Ethical compliance:** The validation process must ensure that models do not produce discriminatory results due to biases, requiring consideration of ethical issues during data collection and model design.
- **Legal liability:** When model decisions lead to problems, it must be possible to trace and verify the model's decision-making process. This will require a legal framework to define liability boundaries, as well as requiring model validation to provide sufficient evidence support.
In summary, model validation is a key link to ensure the reliability and effectiveness of artificial intelligence applications. With continuous technological development, we need to focus not only on technological progress but also on the impact of the model's application in society on ethics and law. The future model validation will be a comprehensive field involving multiple disciplines, providing a guarantee for the sustainable development of artificial intelligence.
0
0
相关推荐
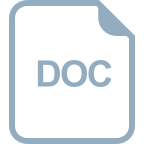
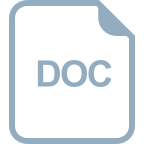
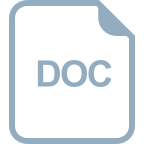
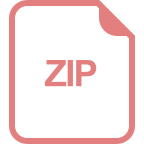
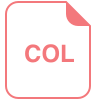
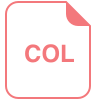
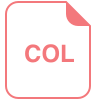
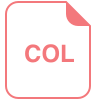
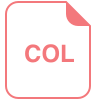