Advanced Feature Engineering Techniques: 10 Methods to Power Up Your Models
发布时间: 2024-09-15 11:26:11 阅读量: 27 订阅数: 32 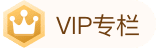
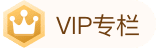
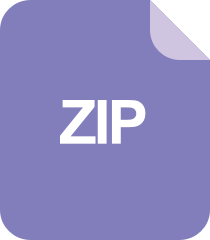
House Prices: Advanced Regression Techniques-数据集
# Advanced Feature Engineering Techniques: 10 Methods to Power Up Your Model
In the realm of machine learning and data analysis, feature engineering is the process of transforming raw data into features that can be used to train efficient learning models. It is a critical step in improving model predictive performance, involving the understanding, transformation, selection, and optimization of data. Effective feature engineering can extract key information, simplify problem complexity, and enhance the efficiency and accuracy of algorithms. This chapter will introduce the basic concepts and core elements of feature engineering, laying the foundation for an in-depth exploration of advanced feature engineering techniques for different types of data in subsequent chapters.
## 1.1 The Importance of Feature Engineering
In practical applications, raw data often cannot be directly used for machine learning models. Data may contain noise, missing values, or inconsistent formats. The primary task of feature engineering is data cleaning and preprocessing to ensure data quality and consistency. In addition, selecting the most explanatory features for the problem can effectively improve model training efficiency and predictive accuracy. For instance, in image recognition tasks, extracting advanced features such as edges and textures from pixel data can better assist classifiers in understanding image content.
## 1.2 The Main Steps of Feature Engineering
Feature engineering typically includes the following core steps:
- Data preprocessing: including data cleaning, normalization, encoding, etc.
- Feature selection: selecting features that help improve model performance from many features.
- Feature construction: creating new features by combining or transforming existing ones.
- Feature extraction: using statistical and mathematical methods to extract information-rich new feature sets from the data.
- Feature evaluation: evaluating the effectiveness and importance of features, providing a basis for feature selection.
Through these steps, we can transform raw data into a high-quality feature set, laying a solid foundation for subsequent model training and testing. Next, we will delve into advanced methods of feature extraction, further revealing the technical details and application scenarios behind feature engineering.
# 2. Advanced Methods of Feature Extraction
Feature extraction is one of the core links in feature engineering, which includes extracting useful information from the original data to form a feature set that can characterize the data properties. This process usually requires the use of statistical methods, model evaluation techniques, and creatively constructing new features.
### 2.1 Statistical-Based Feature Extraction
Statistics provide powerful tools to identify patterns in data, among which entropy and information gain, as well as Principal Component Analysis (PCA), are two commonly used methods.
#### 2.1.1 Applications of Entropy and Information Gain
Entropy is a statistical measure of the disorder of data. In information theory, entropy is used to measure the uncertainty of data. In feature extraction, we usually use information gain to select features. The greater the information gain, the closer the relationship between the feature and the label, and the more helpful it is to extract the feature for classification tasks.
```python
from sklearn.feature_selection import mutual_info_classif
# Assuming X is the feature matrix and y is the label vector
# Use mutual information method to calculate feature selection scores
mi_scores = mutual_info_classif(X, y)
```
The above code uses the scikit-learn library to calculate the mutual information of features, which helps to evaluate the mutual dependence between features and labels. Mutual information is a measure of the interrelation between variables, which is very effective for classification problems. During feature selection, features with higher mutual information values can be chosen.
#### 2.1.2 In-depth Understanding of Principal Component Analysis (PCA)
Principal Component Analysis (PCA) is another powerful feature extraction method. It transforms possibly correlated variables into a set of linearly uncorrelated variables through an orthogonal transformation, known as the principal components. The key to PCA is that it can reduce the dimensionality of data while preserving the most important information, with minimal loss.
```python
from sklearn.decomposition import PCA
import numpy as np
# Assuming X is the normalized feature matrix
pca = PCA(n_components=2) # Retain two principal components
X_pca = pca.fit_transform(X)
```
In the above code, PCA is used for dimensionality reduction. By setting the `n_components` parameter, you can specify the number of principal components to retain. In practical applications, the number of principal components to retain needs to be decided based on the percentage of explained variance. Generally, the principal components that contribute to more than 80% or 90% of the cumulative contribution rate are selected as the feature set after dimensionality reduction.
### 2.2 Model-Based Feature Selection
Model evaluation metrics are directly related to feature selection methods because they provide standards for evaluating the importance of features.
#### 2.2.1 Model Evaluation Metrics and Feature Selection
Model evaluation metrics such as accuracy, recall, F1 score, etc., provide methods for measuring model performance. During the feature selection phase, we can use the scores of these metrics to determine which features are more helpful in improving model performance.
```python
from sklearn.model_selection import cross_val_score
from sklearn.ensemble import RandomForestClassifier
# Assuming X is the feature matrix and y is the label vector
rf = RandomForestClassifier()
scores = cross_val_score(rf, X, y, cv=5)
# Output the average cross-validation score
print("Average cross-validation score:", np.mean(scores))
```
Here, the Random Forest classifier and cross-validation are used to evaluate the feature set. By comparing the performance of models containing different feature sets, we can determine which features are beneficial for model prediction.
#### 2.2.2 Evaluation of Feature Importance Based on Tree Models
Tree models such as decision trees and random forests can provide a measure of feature importance. These models can be used to evaluate the contribution of each feature to the prediction result, thereby achieving model-based feature selection.
```python
importances = rf.feature_importances_
indices = np.argsort(importances)[::-1]
# Print feature importance
for f in range(X.shape[1]):
print("%d. feature %d (%f)" % (f + 1, indices[f], importances[indices[f]]))
```
In the above code snippet, we use the `feature_importances_` attribute of the Random Forest model to view the importance of each feature. Features are sorted by importance, which is very useful for selectively retaining or discarding certain features.
### 2.3 Generation and Application of Combined Features
New features can be generated by combining existing features, capturing the interaction between data.
#### 2.3.1 The Role of Polynomial Features and Cross Features
Polynomial features and cross features are created through the product and power combination of original features. This can increase the model's ability to express complex relationships.
```python
from sklearn.preprocessing import PolynomialFeatures
# Assuming X is the feature matrix
poly = PolynomialFeatures(degree=2, include_bias=False)
X_poly = poly.fit_transform(X)
```
In this code, polynomial features are generated using the `PolynomialFeatures` class, which can create quadratic polynomial combinations of the original features, including the squared terms of individual features. This feature generation method is often used in scenarios where data relationships are believed to be nonlinear.
#### 2.3.2 New Feature Generation Based on Feature Construction
Based on domain knowledge, new features can sometimes be constructed, and such features often significantly improve performance. For example, for time series data, statistical measures of sliding windows can be constructed as features; for text data, features can be constructed through word frequency, sentence length, etc.
```python
# Assuming X is the feature matrix and X_new is the newly constructed feature matrix
X_new = np.hstack([X, X_poly]) # Combine polynomial features with original features
```
By merging the original features with polynomial features, we obtain a richer feature set, which can provide more information in machine learning models, helping to improve the predictive power of the model.
In this chapter, we introduced statistical-based feature extraction methods and how to select features using model evaluation metrics and tree-based methods. We also explored the generation of combined features, including polynomial features and the construction of new features. In the process of feature extraction, mastering and applying these methods can greatly enhance the expressive power of data and lay a solid foundation for subsequent model training.
# 3. Feature Transformation and Normalization Techniques
In the practice of machine learning and data science, feature transformation and normalization are crucial steps. This helps ensure that the model can learn the structure of the data better, while avoiding numerical problems, such as gradient vanishing or gradient explosion. This chapter will delve into nonlinear transformation methods, feature scaling techniques, and feature encoding strategies, putting data in the most suitable state for model learning.
## 3.1 Nonlinear Transformation Methods
### 3.1.1 Power Transform and Box-Cox Transform
In data preprocessing, the power transform is a common method that changes the data distribution by applying a power function, improving the normality of data, thereby enhancing model performance. The formula for the power transform can be expressed as:
\[ Y = X^{\lambda} \]
where, \( \lambda \) is the transformation parameter, which can be estimated by maximizing the log-likelihood function, suitable for continuous variables.
Box-Cox transform is an extension of the power transform, designed to address the situation where there are non-positive numbers in the data. Its transformation formula is as follows:
\[ Y = \begin{cases}
\frac{X^\lambda - 1}{\lambda} & \text{if } \lambda \neq 0 \\
\log(X) & \text{if } \lambda = 0
\end{cases} \]
where, \( \lambda \) is a parameter estimated by maximizing the data's log-likelihood function. If the data contains zeros or negative numbers, the data must first be shifted to make it positive.
### 3.1.2 Applications of Logarithmic and Exponential Transformations
Logarithmic and exponential transformations are special forms of power transforms, particularly useful when data exhibits a skewed distribution, helping to reduce data skewness.
The logarithmic transformation is commonly used to compress larger values and expand smaller ones, helping to balance the data distribution:
\[ Y = \log(X) \]
It is particularly useful when dealing with financial and economic time series data, helping to stabilize data variance.
The exponential transformation is the inverse operation of the logarithmic transformation, used when the data集中 contains negative numbers or zeros:
\[ Y = \exp(X) \]
It is commonly used for inverse power transformations in data, such as in time series forecasting and biostatistics.
## 3.2 Feature Scaling Techniques
### 3.2.1 Min-Max Normalization and Z-score Standardization
The scale of data usually significantly affects model performance, so feature scaling is a necessary step before algorithm training.
Min-Max normalization scales the features to a fixed range, usually the [0,1] interval:
\[ X_{\text{norm}} = \frac{X - X_{\text{min}}}{X_{\text{max}} - X_{\text{min}}} \]
This method is simple and prese
0
0
相关推荐
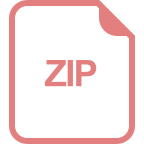
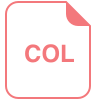
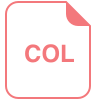
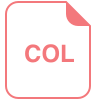
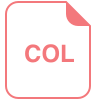
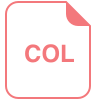
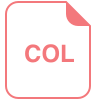
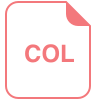