python Harris角点检测
时间: 2023-10-26 15:44:15 浏览: 62
Harris角点检测是一种计算机视觉算法,用于检测图像中的角点。它是由Chris Harris和Mike Stephens在1988年提出的。
Harris角点检测的基本思想是:在图像中的某个点,如果沿着任意方向移动一个小的距离,图像的强度都会发生明显的变化,那么这个点就是角点。Harris角点检测利用图像中像素值的变化率来检测角点。
实现Harris角点检测的基本步骤:
1. 计算图像的梯度:使用Sobel算子或其他的梯度算子计算图像的x和y方向的导数。
2. 计算每个像素点的协方差矩阵:对于每个像素点,计算其周围像素的梯度信息,并计算其协方差矩阵。
3. 计算每个像素点的响应值:利用协方差矩阵计算每个像素点的响应值。
4. 非极大值抑制:对于每个像素点,检查其周围像素的响应值,并将其与周围像素的响应值进行比较,保留响应值最大的像素点。
5. 滞后阈值:对于响应值较小的像素点,如果其周围像素的响应值都比它大,则将其过滤掉。
以下是一个简单的Python实现:
```
import numpy as np
import cv2
def HarrisCornerDetection(image, k=0.04, threshold=0.01):
# 1. 计算图像的梯度
dx = cv2.Sobel(image, cv2.CV_32F, 1, 0, ksize=3)
dy = cv2.Sobel(image, cv2.CV_32F, 0, 1, ksize=3)
# 2. 计算每个像素点的协方差矩阵
Ixx = dx ** 2
Ixy = dx * dy
Iyy = dy ** 2
height, width = image.shape
corner_map = np.zeros((height, width))
# 3. 计算每个像素点的响应值
offset = 1
for y in range(offset, height - offset):
for x in range(offset, width - offset):
Sxx = np.sum(Ixx[y - offset:y + offset + 1, x - offset:x + offset + 1])
Sxy = np.sum(Ixy[y - offset:y + offset + 1, x - offset:x + offset + 1])
Syy = np.sum(Iyy[y - offset:y + offset + 1, x - offset:x + offset + 1])
det = Sxx * Syy - Sxy ** 2
trace = Sxx + Syy
r = det - k * trace ** 2
corner_map[y, x] = r
# 4. 非极大值抑制
corner_map = cv2.dilate(corner_map, None)
corner_map = cv2.erode(corner_map, None)
# 5. 滞后阈值
threshold_value = threshold * corner_map.max()
corner_map[corner_map < threshold_value] = 0
# 返回角点坐标
return np.argwhere(corner_map > 0)
```
使用示例:
```
image = cv2.imread('lena.png', cv2.IMREAD_GRAYSCALE)
keypoints = HarrisCornerDetection(image)
for point in keypoints:
cv2.circle(image, tuple(point[::-1]), 3, (0, 255, 0), -1)
cv2.imshow('Harris Corner Detection', image)
cv2.waitKey()
cv2.destroyAllWindows()
```
其中lena.png是一个灰度图像。运行结果如下图所示:

阅读全文
相关推荐
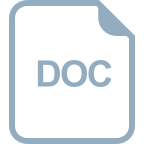
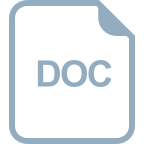
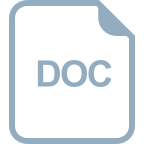















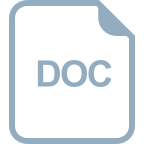