Bellman-Ford算法怎样通过追溯前驱节点来找到负权环的具体路径C++
时间: 2024-04-07 12:33:31 浏览: 12
要通过追溯前驱节点来找到负权环的具体路径,可以在Bellman-Ford算法的基础上进行一些修改。下面是一个用C++实现的示例代码:
```cpp
#include <iostream>
#include <vector>
using namespace std;
struct Edge {
int src, dest, weight;
};
vector<int> findNegativeCycle(vector<Edge>& edges, int V, int E, int source) {
vector<int> dist(V, INT_MAX);
vector<int> parent(V, -1);
dist[source] = 0;
int lastUpdatedVertex;
// Relax all edges V-1 times
for (int i = 1; i <= V - 1; i++) {
lastUpdatedVertex = -1;
for (int j = 0; j < E; j++) {
int u = edges[j].src;
int v = edges[j].dest;
int weight = edges[j].weight;
if (dist[u] != INT_MAX && dist[u] + weight < dist[v]) {
dist[v] = dist[u] + weight;
parent[v] = u;
lastUpdatedVertex = v;
}
}
}
// If there is no update in the last iteration, then there is no negative cycle
if (lastUpdatedVertex == -1)
return {};
// Trace back the negative cycle using the parent array
vector<int> cycle;
int startVertex = lastUpdatedVertex;
for (int i = 0; i < V; i++)
startVertex = parent[startVertex];
int currVertex = startVertex;
cycle.push_back(currVertex);
do {
currVertex = parent[currVertex];
cycle.push_back(currVertex);
} while (currVertex != startVertex);
return cycle;
}
int main() {
int V, E;
cout << "Enter the number of vertices and edges: ";
cin >> V >> E;
vector<Edge> edges(E);
cout << "Enter the source, destination, and weight of each edge:\n";
for (int i = 0; i < E; i++)
cin >> edges[i].src >> edges[i].dest >> edges[i].weight;
int source;
cout << "Enter the source vertex: ";
cin >> source;
vector<int> cycle = findNegativeCycle(edges, V, E, source);
if (cycle.empty()) {
cout << "No negative cycle found.\n";
} else {
cout << "Negative cycle found: ";
for (int vertex : cycle)
cout << vertex << " ";
cout << endl;
}
return 0;
}
```
在代码中,我们使用了`dist`数组来存储从起点到每个顶点的最短路径距离,使用`parent`数组来记录每个顶点的前驱节点。在算法的最后,我们通过追溯`parent`数组来找到负权环的具体路径。
请注意,这只是一个示例代码,并未进行详尽的输入验证和错误处理。在实际应用中,需要根据具体情况进行适当的修改和完善。
相关推荐
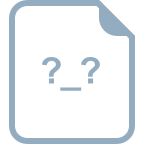














