python按列写入xlxs
时间: 2023-11-15 13:02:51 浏览: 80
在Python中,我们可以使用openpyxl库来按列写入xlsx文件。具体步骤如下:
1. 导入openpyxl库
2. 创建一个Workbook对象
3. 获取或创建一个Worksheet对象
4. 使用Worksheet对象的cell方法来写入数据,指定行和列的位置
5. 使用Workbook对象的save方法保存文件
下面是一个示例代码,演示如何按列写入xlsx文件:
```
import openpyxl
# 创建Workbook对象
workbook = openpyxl.Workbook()
# 获取或创建Worksheet对象
sheet = workbook.active
# 写入数据
data = ['apple', 'banana', 'orange', 'pear']
for i in range(len(data)):
sheet.cell(row=i+1, column=1, value=data[i])
# 保存文件
workbook.save(filename='test.xlsx')
```
在上面的示例代码中,我们创建了一个Workbook对象,然后获取或创建了一个Worksheet对象。接着,我们使用Worksheet对象的cell方法来按列写入数据,指定行和列的位置。最后,我们使用Workbook对象的save方法保存文件。
相关问题
python xlxs
回答: Python中处理xlsx文件可以使用openpyxl库进行读写操作。如果文件后缀是xlsx,可以使用openpyxl库进行读写操作。你可以使用openpyxl库的相关函数来读取和写入xlsx文件。具体的使用方法可以参考openpyxl的官方文档\[3\]。另外,如果你想使用pandas生成xlsx文件,可以使用pandas库的to_excel函数来实现\[2\]。
#### 引用[.reference_title]
- *1* [python--xlsx文件的读写](https://blog.csdn.net/liuyingying0418/article/details/101066630)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [python生成excel文件的三种方式](https://blog.csdn.net/cainiao_python/article/details/131179392)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [【Python】使用Python操作XLSX数据表](https://blog.csdn.net/weixin_52058417/article/details/123266853)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python 多个print 到 xlxs文件
你可以使用 Python 中的 openpyxl 库来将多个 print 输出到 Excel 文件中。下面是一个简单的示例代码:
```python
from openpyxl import Workbook
# 创建一个工作簿
wb = Workbook()
# 选择活动工作表
ws = wb.active
# 输出多个字符串
strings = ['Hello', 'World', 'Python']
for i, s in enumerate(strings):
# 将字符串写入工作表中的单元格
ws.cell(row=i+1, column=1).value = s
# 保存工作簿
wb.save('output.xlsx')
```
在这个例子中,我们首先创建一个工作簿,然后选择活动工作表。然后我们使用一个 for 循环来迭代多个字符串,并将它们写入工作表中的单元格。最后,我们保存工作簿到一个名为 "output.xlsx" 的 Excel 文件中。
你可以根据需要修改这个代码,以满足你的实际需求。
相关推荐
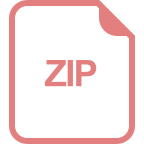
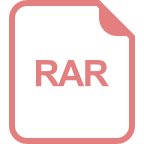







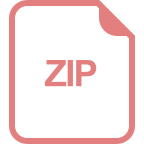
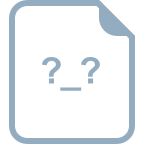
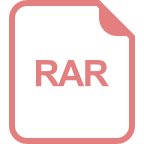
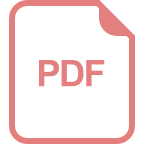
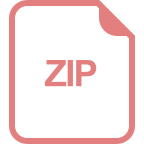