基于eclipse map reduce hadoop的销售统计代码
时间: 2024-03-09 09:49:09 浏览: 105
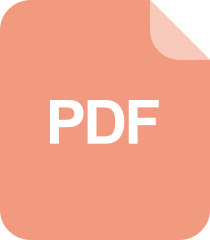
在Eclipse下的Hadoop0.17.0(MapReduce)的统计作业指导书

好的,以下是一个简单的基于Eclipse、MapReduce和Hadoop的销售统计代码示例,供您参考:
1. Mapper类
```java
public class SalesMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
private Text outputKey = new Text();
private IntWritable outputValue = new IntWritable();
@Override
protected void map(LongWritable key, Text value, Context context)
throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split(",");
// 获取商品名称和销售数量
String productName = fields[0];
int sales = Integer.parseInt(fields[1]);
// 将商品名称作为MapReduce的输出key,销售数量作为输出value
outputKey.set(productName);
outputValue.set(sales);
context.write(outputKey, outputValue);
}
}
```
2. Reducer类
```java
public class SalesReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
private IntWritable outputValue = new IntWritable();
@Override
protected void reduce(Text key, Iterable<IntWritable> values, Context context)
throws IOException, InterruptedException {
int sum = 0;
for (IntWritable value : values) {
sum += value.get();
}
// 输出每个商品的总销售数量
outputValue.set(sum);
context.write(key, outputValue);
}
}
```
3. Driver类
```java
public class SalesDriver {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf);
job.setJarByClass(SalesDriver.class);
job.setMapperClass(SalesMapper.class);
job.setReducerClass(SalesReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.setInputPaths(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
boolean result = job.waitForCompletion(true);
System.exit(result ? 0 : 1);
}
}
```
以上是一个简单的示例代码,根据实际情况和需求进行修改和完善。在运行代码前,需要将代码打包成jar包并上传到Hadoop集群进行运行。
阅读全文
相关推荐
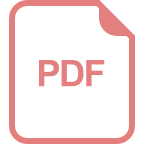
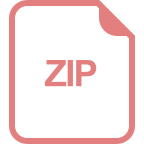

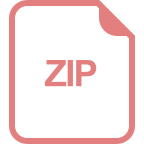
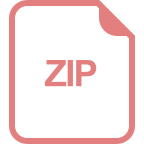

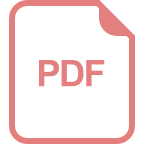
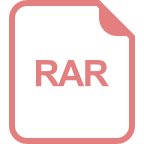
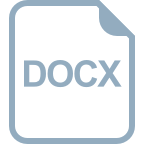
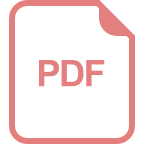
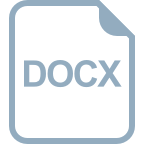
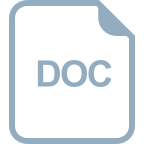
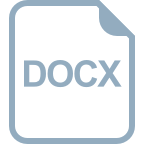
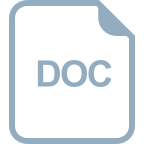
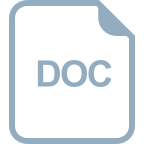
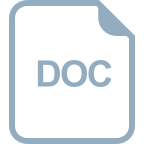
