springboot调用方法前处理
时间: 2023-07-07 10:24:05 浏览: 47
在 Spring Boot 中,我们可以使用 AOP(面向切面编程)技术来实现调用方法前的处理。具体步骤如下:
1.定义一个切面类,使用 @Aspect 注解标注。
2.在切面类中定义一个前置通知方法,使用 @Before 注解标注。
3.在前置通知方法中编写需要执行的逻辑。
4.在需要调用的方法上添加切点注解,如 @Pointcut。
5.在需要调用的方法上添加切面注解,如 @Around。
6.在应用启动类上添加 @EnableAspectJAutoProxy 注解开启自动代理。
举个例子,如果我们需要在调用一个方法前打印一条日志,可以按照以下步骤实现:
1.定义一个切面类,使用 @Aspect 注解标注。
```java
@Aspect
@Component
public class LogAspect {
@Before("execution(* com.example.demo.service.*.*(..))")
public void before(JoinPoint joinPoint) {
String methodName = joinPoint.getSignature().getName();
String className = joinPoint.getTarget().getClass().getName();
System.out.println("调用 " + className + " 类的 " + methodName + " 方法");
}
}
```
2.在需要调用的方法上添加切点注解,如 @Pointcut。
```java
@Pointcut("execution(* com.example.demo.service.UserService.*(..))")
public void userServicePointcut() {}
```
3.在需要调用的方法上添加切面注解,如 @Around。
```java
@Around("userServicePointcut()")
public Object around(ProceedingJoinPoint proceedingJoinPoint) throws Throwable {
// 执行前置逻辑
before(proceedingJoinPoint);
// 执行原方法
Object result = proceedingJoinPoint.proceed();
// 执行后置逻辑
after(proceedingJoinPoint);
return result;
}
```
4.在应用启动类上添加 @EnableAspectJAutoProxy 注解开启自动代理。
```java
@SpringBootApplication
@EnableAspectJAutoProxy
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
这样,在调用 UserService 类的任何方法前都会打印一条日志。
相关推荐
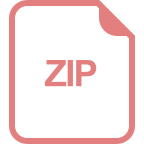
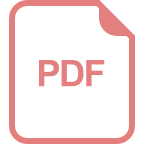
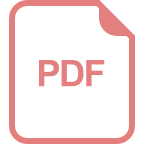














