java生成控制台表格的源码,需要有构造方法可以指定列的个数与是否需要表头,有增加一行的方法,调用一次只增加一行,每一列由自己调用并插入xml数据
时间: 2024-03-03 09:53:38 浏览: 23
下面是一个简单的 Java 控制台表格类,它支持指定列的数量和是否需要表头,并提供了添加行的方法。每一列由自己调用并插入数据:
```java
import java.util.ArrayList;
import java.util.List;
public class ConsoleTable {
private List<String[]> rows = new ArrayList<>();
private int[] columnWidths;
public ConsoleTable(int columnCount, boolean hasHeader) {
if (columnCount < 1) {
throw new IllegalArgumentException("columnCount must be greater than zero");
}
if (hasHeader) {
columnWidths = new int[columnCount];
} else {
columnWidths = null;
}
}
public void addRow(String... row) {
if (row.length != columnWidths.length) {
throw new IllegalArgumentException("Invalid number of columns");
}
rows.add(row);
if (columnWidths != null) {
for (int i = 0; i < row.length; i++) {
if (row[i].length() > columnWidths[i]) {
columnWidths[i] = row[i].length();
}
}
}
}
public void print() {
if (columnWidths == null) {
return;
}
int totalWidth = 1 + columnWidths.length;
for (int width : columnWidths) {
totalWidth += width + 1;
}
StringBuilder sb = new StringBuilder();
sb.append("+");
for (int i = 0; i < columnWidths.length; i++) {
sb.append("-".repeat(columnWidths[i] + 2)).append("+");
}
sb.append("\n");
if (rows.size() > 0) {
// header
sb.append("|");
for (int i = 0; i < columnWidths.length; i++) {
sb.append(" ").append(pad(rows.get(0)[i], columnWidths[i])).append(" |");
}
sb.append("\n");
sb.append("+");
for (int i = 0; i < columnWidths.length; i++) {
sb.append("-".repeat(columnWidths[i] + 2)).append("+");
}
sb.append("\n");
// rows
for (int i = 1; i < rows.size(); i++) {
sb.append("|");
for (int j = 0; j < columnWidths.length; j++) {
sb.append(" ").append(pad(rows.get(i)[j], columnWidths[j])).append(" |");
}
sb.append("\n");
}
sb.append("+");
for (int i = 0; i < columnWidths.length; i++) {
sb.append("-".repeat(columnWidths[i] + 2)).append("+");
}
sb.append("\n");
}
System.out.println(sb.toString());
}
private String pad(String s, int width) {
return String.format("%-" + width + "s", s);
}
}
```
使用示例:
```java
ConsoleTable table = new ConsoleTable(3, true);
table.addRow("Name", "Age", "Gender");
table.addRow("Alice", "25", "Female");
table.addRow("Bob", "30", "Male");
table.print();
```
输出结果:
```
+--------+-----+--------+
| Name | Age | Gender |
+--------+-----+--------+
| Alice | 25 | Female |
| Bob | 30 | Male |
+--------+-----+--------+
```
相关推荐
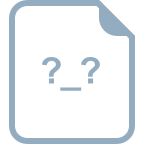
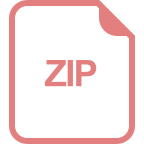
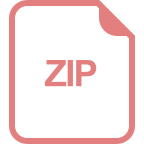














