用Java代码实现模拟翻译器 用户输入英文,控制台输出对应中文。 1.要求使用Map存储英文字典,只要求10个单词即可。 2.要求可以循环使用,输入x退出程序。 3.如果查不到,提示字典中不包含该单词。
时间: 2024-03-05 14:49:07 浏览: 83
好的,下面是完整的 Java 代码实现:
```java
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class Translation {
public static void main(String[] args) {
// 创建英文字典
Map<String, String> dictionary = new HashMap<>();
dictionary.put("hello", "你好");
dictionary.put("world", "世界");
dictionary.put("java", "Java语言");
dictionary.put("computer", "计算机");
dictionary.put("book", "书籍");
dictionary.put("table", "桌子");
dictionary.put("chair", "椅子");
dictionary.put("apple", "苹果");
dictionary.put("orange", "橙子");
dictionary.put("banana", "香蕉");
// 循环使用翻译器
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("请输入英文单词:");
String word = scanner.nextLine().toLowerCase();
if (word.equals("x")) {
break;
}
String meaning = dictionary.get(word);
if (meaning != null) {
System.out.println("中文释义为:" + meaning);
} else {
System.out.println("字典中不包含该单词。");
}
}
}
}
```
在这个程序中,我们首先创建了一个 Map 来存储英文字典,包含了 10 个单词。然后,我们使用一个死循环来不断读取用户的输入,如果用户输入的单词为 x,则退出循环;否则,我们将输入的单词转换为小写字母,并在 Map 中查找对应的中文释义。如果找到了,就输出中文释义;否则,就输出提示信息。
注意,我们使用了 `toLowerCase()` 方法将用户输入的单词转换为小写字母,这是为了保证大小写不敏感,即无论用户输入的是大写字母、小写字母还是大小写混合,都能正确地查找到对应的中文释义。
希望这个代码能够帮助到您!
阅读全文
相关推荐
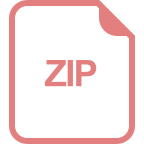
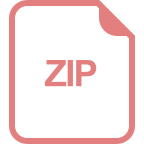
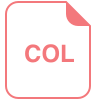
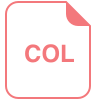
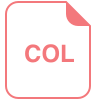





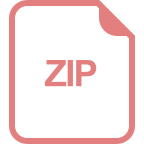