查找数组中所有 两个key对应值其中一个相同活全部相同的数据
时间: 2024-02-23 19:58:46 浏览: 101
好的,让我解释一下你的问题。你想在一个数组中查找所有满足以下条件的数据:
1. 该数据中有两个键对应的值中,至少有一个相同;
2. 或者该数据中有两个键对应的值都相同。
是这样吗?
如果是的话,你可以按照以下步骤进行:
1. 遍历数组中的每个元素;
2. 对于每个元素,再遍历一遍数组中的所有元素,找到所有满足条件的元素,并将它们存储在一个新的数组中;
3. 最后返回这个新的数组。
代码实现可能会像这样:
```python
def find_similar_values(arr):
result = []
for i in range(len(arr)):
for j in range(i+1, len(arr)):
if any(value in arr[j].values() for value in arr[i].values()) or arr[i].items() <= arr[j].items():
result.append(arr[i])
result.append(arr[j])
return result
```
这段代码会返回一个包含所有满足条件的元素的新数组。注意,这个算法的时间复杂度是 O(n^2),如果数组很大的话,可能会比较慢。
相关问题
题目描述 已知一维数组中的10个元素各不相同,但已按升序排列。查找数组中是否存在值为key的数组元素。如果有,输出相应的下标,否则输出not found。你有什么好方法吗? 输入要求 先从键盘输入10个升序排列的整数,然后再输入一个待查找的数据key。 输出要求 输出对应结果。若不存在则输出not found. 输入样例 6 7 9 10 16 18 20 35 141 150 21 输出样例 not found代码
可以使用二分查找的方法来查找数组中是否存在值为key的元素。具体步骤如下:
1. 定义左右两个指针left和right,分别指向数组的起始和末尾位置。
2. 在循环中,每次计算出中间位置mid = (left + right) / 2,然后将中间位置的元素与key进行比较。
3. 如果中间位置的元素等于key,则直接输出mid即为对应下标。
4. 如果中间位置的元素大于key,则说明key可能在左半部分,将right指针移动到mid-1的位置。
5. 如果中间位置的元素小于key,则说明key可能在右半部分,将left指针移动到mid+1的位置。
6. 循环执行以上步骤,直到left>right为止。
7. 如果最终left>right,说明数组中不存在值为key的元素,输出not found。
下面是对应的代码实现:
使用C语言编写程序给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出 和为目标值 target 的那 两个 整数,并返回它们的数组下标。 你可以假设每种输入只会对应一个答案,并且你不能使用两次相同的元素。 你可以按任意顺序返回答案。输入整数N
在C语言中,可以通过创建一个哈希表来存储遍历数组时的元素值和对应的索引,以实现快速查找和为为目标值的两个整数。下面是一种可能的实现方法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义哈希表节点结构体
typedef struct HashNode {
int key;
int value;
struct HashNode *next;
} HashNode;
// 定义哈希表结构体
typedef struct {
HashNode **buckets;
int size;
} HashTable;
// 创建哈希表
HashTable* createHashTable(int size) {
HashTable *hashTable = (HashTable*)malloc(sizeof(HashTable));
hashTable->size = size;
hashTable->buckets = (HashNode**)malloc(sizeof(HashNode*) * size);
for (int i = 0; i < size; i++) {
hashTable->buckets[i] = NULL;
}
return hashTable;
}
// 释放哈希表内存
void freeHashTable(HashTable *hashTable) {
for (int i = 0; i < hashTable->size; i++) {
HashNode *node = hashTable->buckets[i];
while (node) {
HashNode *temp = node;
node = node->next;
free(temp);
}
}
free(hashTable->buckets);
free(hashTable);
}
// 插入键值对到哈希表
void insert(HashTable *hashTable, int key, int value) {
int index = key % hashTable->size;
HashNode *newNode = (HashNode*)malloc(sizeof(HashNode));
newNode->key = key;
newNode->value = value;
newNode->next = hashTable->buckets[index];
hashTable->buckets[index] = newNode;
}
// 在哈希表中查找键对应的值
int search(HashTable *hashTable, int key) {
int index = key % hashTable->size;
HashNode *node = hashTable->buckets[index];
while (node) {
if (node->key == key) {
return node->value;
}
node = node->next;
}
return -1; // 未找到
}
// 返回数组中两个整数的和为target的下标
int* twoSum(int* nums, int numsSize, int target, int* returnSize) {
HashTable *hashTable = createHashTable(numsSize);
int *result = (int*)malloc(sizeof(int) * 2);
for (int i = 0; i < numsSize; ++i) {
int complement = target - nums[i];
int complementIndex = search(hashTable, complement);
if (complementIndex != -1) {
result[0] = complementIndex;
result[1] = i;
*returnSize = 2;
freeHashTable(hashTable);
return result;
}
insert(hashTable, nums[i], i);
}
*returnSize = 0;
freeHashTable(hashTable);
return result;
}
int main() {
int nums[] = {2, 7, 11, 15};
int target = 9;
int returnSize = 0;
int* indices = twoSum(nums, sizeof(nums) / sizeof(nums[0]), target, &returnSize);
if (returnSize == 2) {
printf("找到的两个数的下标为: [%d, %d]\n", indices[0], indices[1]);
} else {
printf("未找到符合条件的两个数\n");
}
free(indices);
return 0;
}
```
这段代码定义了一个哈希表,并提供了创建、插入和查找的功能。`twoSum`函数利用这个哈希表来快速查找是否存在和为`target`的两个整数。如果找到,返回它们的下标;否则,返回没有找到的信息。
阅读全文
相关推荐
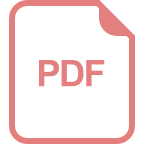
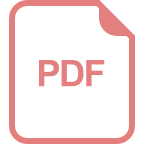
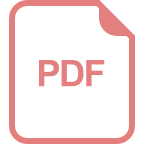
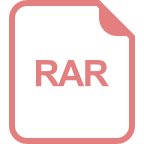
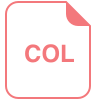








