python Qprocess示例
时间: 2023-10-26 14:04:53 浏览: 164
当使用Python中的QProcess类时,可以使用以下示例作为参考:
```python
from PyQt5.QtCore import QProcess
# 创建QProcess对象
process = QProcess()
# 设置要执行的命令和参数
command = 'ls'
arguments = ['-l', '/']
process.start(command, arguments)
# 连接信号槽以处理进程输出
process.readyReadStandardOutput.connect(lambda: print(process.readAllStandardOutput().data().decode()))
# 启动进程并等待其完成
process.waitForFinished()
# 获取进程退出代码
exitCode = process.exitCode()
print(f"进程退出代码: {exitCode}")
```
在这个示例中,我们创建了一个QProcess对象,并设置要执行的命令和参数。然后,我们连接了readyReadStandardOutput信号槽,以便在进程有输出时打印它们。接着,我们启动进程并使用waitForFinished方法等待它完成。最后,我们获取进程的退出代码并打印出来。
请注意,这个示例使用了PyQt5库,因此需要确保已经安装了该库。如果没有安装,可以使用以下命令进行安装:
```
pip install PyQt5
```
希望这个示例对你有帮助!如果有任何问题,请随时提问。
相关问题
QProcess如何用命令参数调用python脚本
使用QProcess调用Python脚本需要通过命令行参数传递Python脚本文件的路径和参数。可以使用QProcess::start方法启动Python解释器并传递命令行参数。
以下是一个示例代码:
```c++
#include <QCoreApplication>
#include <QProcess>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// Python脚本路径
QString pythonScript = "path/to/python/script.py";
// Python脚本参数
QStringList pythonArgs;
pythonArgs << "arg1" << "arg2" << "arg3";
// 创建QProcess对象
QProcess process;
// 设置Python解释器路径
process.setProgram("python");
// 添加Python脚本路径和参数
QStringList arguments;
arguments << pythonScript << pythonArgs;
process.setArguments(arguments);
// 启动进程
process.start();
return a.exec();
}
```
在上面的代码中,pythonScript变量包含Python脚本的路径,pythonArgs变量包含Python脚本的参数。使用QProcess::setProgram方法设置Python解释器的路径,使用QProcess::setArguments方法将Python脚本路径和参数添加到QProcess对象中。最后,使用QProcess::start方法启动进程。
以上是一个简单的示例,可以根据需要进行修改。
使用pyqt5中的QProcess执行另一个py文件,报错:QProcess: Destory while process ("python") is still running
这个错误通常发生在你销毁了 QProcess 对象但是子进程仍然在运行的时候。为了避免这个错误,你可以在销毁 QProcess 对象之前,先调用其 `kill()` 方法来终止子进程。
以下是一个示例代码,演示如何使用 QProcess 执行另一个 Python 文件,并在程序退出时终止子进程:
```python
import sys
from PyQt5.QtCore import QProcess
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.process = None
button = QPushButton('Run', self)
button.clicked.connect(self.start_process)
def start_process(self):
if self.process is not None and self.process.state() != QProcess.NotRunning:
# 如果进程正在运行,不要启动一个新的进程
return
self.process = QProcess()
self.process.finished.connect(self.process_finished)
self.process.start('python', ['other_script.py'])
def process_finished(self):
print('Process finished with exit code', self.process.exitCode())
self.process = None
def closeEvent(self, event):
if self.process is not None and self.process.state() != QProcess.NotRunning:
# 在程序退出之前,终止子进程
self.process.kill()
self.process.waitForFinished()
event.accept()
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在这个示例中,我们创建了一个 `MainWindow` 类,其中包含一个按钮,点击按钮时会启动另一个 Python 脚本 `other_script.py`。我们定义了 `start_process()` 方法来启动 QProcess,`process_finished()` 方法来处理子进程结束的信号,以及 `closeEvent()` 方法来在程序退出时终止子进程。
在 `start_process()` 方法中,我们首先检查之前是否已经启动了一个进程(即 `self.process` 不为 `None`),如果是,则直接返回。否则,我们创建一个新的 QProcess 对象,并调用其 `start()` 方法来启动 Python 解释器,并传入要执行的脚本文件路径。在 `process_finished()` 方法中,我们打印子进程的退出代码,并将 `self.process` 设置为 `None`,以便我们可以在下次点击按钮时启动新的进程。
在 `closeEvent()` 方法中,我们检查当前是否有进程正在运行,如果是,则调用 `kill()` 方法和 `waitForFinished()` 方法来终止子进程。最后,我们接受关闭事件并退出程序。
阅读全文
相关推荐
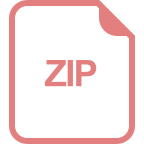
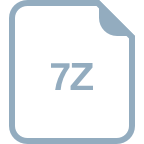
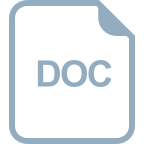
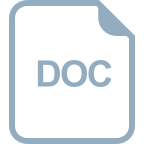









