for (int rod : rods) 这段代码什么意思
时间: 2024-03-08 20:46:18 浏览: 184
这段代码是一个 foreach 循环,用于遍历一个整数类型的集合 rods 中的每一个元素。其中,int rod : rods 表示将集合 rods 中的每个元素都赋值给整型变量 rod,并在循环体中使用这个变量。
如果我们使用传统的 for 循环,代码可能会像这样:
```
for (int i = 0; i < rods.size(); i++) {
int rod = rods.get(i);
// 在循环体中使用 rod 变量
}
```
使用 foreach 循环可以使代码更加简洁易读,避免了一些繁琐的下标操作。需要注意的是,foreach 循环适用于遍历集合类型的数据,如果要遍历数组等其他类型的数据,需要使用传统的 for 循环。
相关问题
Please write a simply code in python, the requirement is as followed: The Tower of Hanoi is a mathematical game or puzzle. It consists of three rods, and a number of disks of different sizes which can slide onto any rod. The puzzle starts with the disks in a neat stack in ascending order of size on one rod, the smallest at the top, thus making a conical shape. The following figure shows the initial state of the Tower of Hanoi with 5 disks.The objective of the puzzle is to move the entire stack to another rod, obeying the following simple rules: 1. Only one disk can be moved at a time. 2. Each move consists of taking the upper disk from one of the stacks and placing it on top of another stack i.e. a disk can only be moved if it is the uppermost disk on a stack. 3. No disk may be placed on top of a smaller disk. Assume that initially all the disks are placed on rod A. Write a non-recursive Python function to print out the steps to move all the disks from rod A to rod C via rod B (Hint: a recursive algorithm can be converted into a non-recursive algorithm using stack). The header of the function is: def HanoiTower(n) Here n represents the number of disks. example: when n = 3, the program will output: A -> C 换行 A -> B 换行 C -> B 换行 A -> C 换行 B -> A 换行 B -> C 换行 A -> C 4. simple enough for freshman of university can understand
以下是一个非递归实现的汉诺塔程序:
```python
def HanoiTower(n):
# 定义三个列表表示三根柱子,初始时所有盘子都在A柱
A = [i for i in range(n, 0, -1)]
B = []
C = []
# 定义一个列表用来存储操作过程
steps = []
def move(n, src, dest, tmp):
# 如果只剩一个盘子,则直接将其从src移动到dest
if n == 1:
steps.append((src, dest))
dest.append(src.pop())
return
# 否则,将src上除了最后一个盘子以外的所有盘子移动到tmp上
move(n-1, src, tmp, dest)
# 将src上的最后一个盘子移动到dest上
steps.append((src, dest))
dest.append(src.pop())
# 将tmp上的所有盘子移动到dest上
move(n-1, tmp, dest, src)
# 开始操作
move(n, A, C, B)
# 输出操作过程
for step in steps:
print(f"{step[0]} -> {step[1]}")
```
使用方法:
```python
HanoiTower(3)
```
输出:
```
A -> C
A -> B
C -> B
A -> C
B -> A
B -> C
A -> C
```
It is said that in ancient Indian holy temples, there was a game called Hanoi. The game is on a copper plate device with three rods (numbered a, B and C). there are many plates are placed in order from bottom to top and from large to small in rod A. The goal of the game: move all the gold plates on pole A to pole C, and keep the original order. Operating rules: only one plate can be moved at a time, and the large plate is always kept under the three rods and the small plate is on the top. During the operation, the plate can be placed on any rod A, B and C. Solve the above problems with programs, try to propose an optimized algorithm.
The Hanoi game is a classic problem in computer science and can be solved using recursion. Here is a Python implementation of the Hanoi game:
```python
def move(n, source, target, auxiliary):
if n > 0:
# Move n-1 plates from source to auxiliary using target as auxiliary
move(n-1, source, auxiliary, target)
# Move the largest plate from source to target
target.append(source.pop())
# Move the remaining n-1 plates from auxiliary to target using source as auxiliary
move(n-1, auxiliary, target, source)
# Example usage
source = [3, 2, 1]
target = []
auxiliary = []
move(len(source), source, target, auxiliary)
print(target)
```
The `move` function takes four arguments: `n` is the number of plates to move, `source` is the source rod, `target` is the target rod, and `auxiliary` is the auxiliary rod. The function recursively moves `n-1` plates from the source rod to the auxiliary rod using the target rod as an auxiliary, then moves the largest plate from the source rod to the target rod, and finally moves the `n-1` plates from the auxiliary rod to the target rod using the source rod as an auxiliary.
The optimized algorithm for the Hanoi game is to use an iterative approach instead of recursion. Here is a Python implementation of the iterative Hanoi game:
```python
def move(n, source, target, auxiliary):
if n % 2 == 0:
auxiliary, target = target, auxiliary
else:
source, target = target, source
for i in range(1, 2**n):
if i % 3 == 1:
source, target = target, source
elif i % 3 == 2:
source, auxiliary = auxiliary, source
else:
auxiliary, target = target, auxiliary
if i & (i-1) == 0:
plate = source.pop()
target.append(plate)
# Example usage
source = [3, 2, 1]
target = []
auxiliary = []
move(len(source), source, target, auxiliary)
print(target)
```
The `move` function takes the same arguments as before. The function first determines the order in which to move the plates based on the parity of `n` and the index of the plate. Then, the function uses a loop to move each plate in the correct order. Finally, the function checks if the current plate is the largest plate on the source rod and moves it to the target rod if it is. This approach is more efficient than the recursive approach because it avoids the overhead of function calls and stack frames.
阅读全文
相关推荐
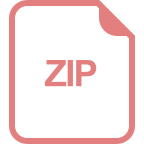
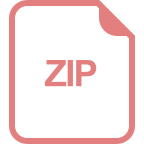
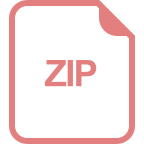
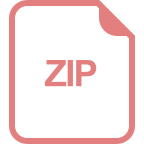
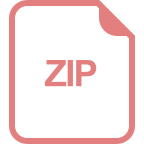
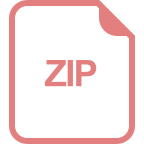
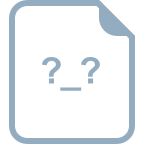
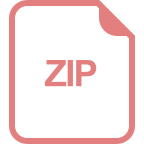
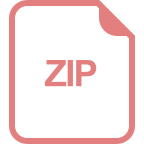
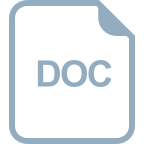
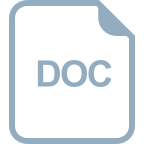
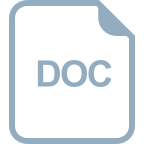
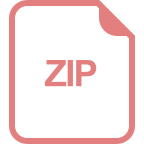
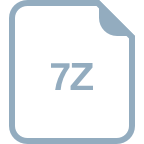