C# FileStream读取8BIM psd取psd文件大小
时间: 2023-07-05 09:23:53 浏览: 64
如果你需要读取PSD文件的大小信息,可以使用C#的FileStream来实现。具体来说,你需要读取PSD文件的第二个段落,也就是从文件头部之后到第一个图层数据之前的部分。这个段落包含了PSD文件的各种元数据信息,其中也包括了文件的大小信息。
以下是一个示例代码,可以读取PSD文件的大小信息:
```csharp
using System.IO;
public static void ReadPsdFileSize(string filePath)
{
using (FileStream stream = new FileStream(filePath, FileMode.Open, FileAccess.Read))
using (BinaryReader reader = new BinaryReader(stream))
{
// 读取文件标识符
byte[] identifier = reader.ReadBytes(6);
if (identifier[0] != 'P' || identifier[1] != 'S' || identifier[2] != 'D' || identifier[3] != '\0')
{
throw new InvalidDataException("Not a valid PSD file.");
}
// 读取文件头信息
reader.BaseStream.Seek(10, SeekOrigin.Begin);
ushort channels = reader.ReadUInt16();
int height = reader.ReadInt32();
int width = reader.ReadInt32();
ushort depth = reader.ReadUInt16();
ushort mode = reader.ReadUInt16();
// 跳过色彩数据信息
reader.BaseStream.Seek(4, SeekOrigin.Current);
// 读取元数据信息
long metadataStart = reader.BaseStream.Position;
while (metadataStart < reader.BaseStream.Length)
{
// 读取段落类型,段落长度和段落内容
byte[] sectionType = reader.ReadBytes(4);
int sectionLength = reader.ReadInt32();
byte[] sectionContent = reader.ReadBytes(sectionLength);
// 如果是文件尺寸段落,输出文件大小信息
if (sectionType[0] == '8' && sectionType[1] == 'B' && sectionType[2] == 'I' && sectionType[3] == 'M')
{
MemoryStream memoryStream = new MemoryStream(sectionContent);
BinaryReader sectionReader = new BinaryReader(memoryStream);
byte[] signature = sectionReader.ReadBytes(4);
int sectionSize = sectionReader.ReadInt32();
byte[] sectionData = sectionReader.ReadBytes(sectionSize);
if (signature[0] == '8' && signature[1] == 'B' && signature[2] == 'I' && signature[3] == 'M')
{
int fileSize = BitConverter.ToInt32(sectionData, 0);
Console.WriteLine("File size: {0} bytes", fileSize);
}
}
// 跳过段落填充
if (sectionLength % 2 == 1)
{
reader.ReadByte();
}
// 如果已经到达第一个图层数据之前的位置,退出循环
if (sectionType[0] == 'L' && sectionType[1] == 'A' && sectionType[2] == 'Y' && sectionType[3] == 'R')
{
break;
}
metadataStart += 4 + sectionLength + (sectionLength % 2 == 1 ? 1 : 0);
}
}
}
```
注意,这只是一个示例代码,并不能保证适用于所有PSD文件。在实际应用中,你需要考虑更多的PSD文件格式和异常情况,以确保正确解析PSD文件的大小信息。
相关推荐
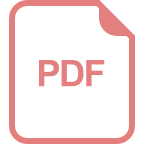
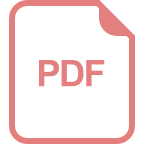
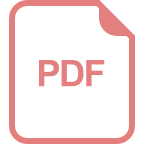














