用C语言指针写一个max函数求一个二维数组所有元素的最大值
时间: 2024-09-19 13:06:54 浏览: 208
在C语言中,你可以使用指针来遍历二维数组并找到最大值。这里是一个简单的示例,假设我们有一个二维整数数组`arr`,其行数由`rows`指定,列数由`cols`指定:
```c
#include <stdio.h>
// 定义一个函数接受二维数组的指针、行数和列数
int findMax(int (*arr)[cols], int rows, int cols) {
// 初始化最大值为数组的第一个元素
int max = arr[0][0];
// 使用指针遍历数组
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
// 如果当前元素大于已知的最大值,更新最大值
if (arr[i][j] > max) {
max = arr[i][j];
}
}
}
return max;
}
int main() {
// 示例二维数组
const int rows = 3;
const int cols = 4;
int arr[rows][cols] = {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}};
// 找到并打印最大值
int max_value = findMax(arr, rows, cols);
printf("二维数组中的最大值是:%d\n", max_value);
return 0;
}
```
在这个例子中,`findMax`函数接收一个指向二维数组首元素的指针,并通过嵌套循环遍历整个数组。当遇到更大的元素时,它会更新`max`的值。
阅读全文
相关推荐
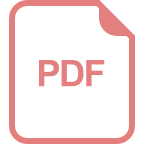
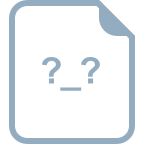
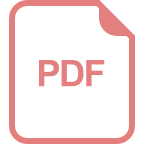















