java 以 post请求方式 在请求Header中添加app_key为xxx ,timestamps为xxx ,random为xxx,sign为xxx,body中参数为grant_type为xxx,client_id为xxx参数以form形
时间: 2024-05-17 17:15:50 浏览: 19
式提交,该如何实现?
你可以使用 Java 的 HttpUrlConnection 类来发送 POST 请求,并设置请求头和请求体参数。具体实现步骤如下:
1. 创建一个 URL 对象,设置请求的 URL 地址。
2. 通过 URL.openConnection() 方法获取一个 HttpURLConnection 对象。
3. 设置请求方法为 POST 。
4. 设置请求头,包括 app_key、timestamps、random 和 sign。
5. 设置请求体参数,包括 grant_type 和 client_id。
6. 发送请求并获取服务器响应结果。
示例代码如下:
```java
import java.net.HttpURLConnection;
import java.net.URL;
import java.io.OutputStream;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.util.Date;
import java.util.Random;
import java.security.MessageDigest;
public class PostRequestExample {
public static void main(String[] args) throws Exception {
String appKey = "xxx";
String clientId = "xxx";
String grantType = "xxx";
String secretKey = "xxx";
// 设置请求 URL
URL url = new URL("http://example.com/api/token");
// 创建 HttpURLConnection 对象
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 设置请求方法为 POST
connection.setRequestMethod("POST");
// 设置请求头
String timestamps = String.valueOf(new Date().getTime());
String random = String.valueOf(new Random().nextInt(100000));
String sign = getSign(appKey, secretKey, timestamps, random);
connection.setRequestProperty("app_key", appKey);
connection.setRequestProperty("timestamps", timestamps);
connection.setRequestProperty("random", random);
connection.setRequestProperty("sign", sign);
// 设置请求体参数
String requestBody = "grant_type=" + grantType + "&client_id=" + clientId;
connection.setDoOutput(true);
OutputStream outputStream = connection.getOutputStream();
outputStream.write(requestBody.getBytes());
outputStream.flush();
outputStream.close();
// 发送请求并获取服务器响应结果
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
System.out.println(response.toString());
}
private static String getSign(String appKey, String secretKey, String timestamps, String random) throws Exception {
String toSign = secretKey + "app_key" + appKey + "random" + random + "timestamps" + timestamps + secretKey;
MessageDigest md = MessageDigest.getInstance("SHA-256");
byte[] bytes = md.digest(toSign.getBytes());
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
}
```
以上代码仅供参考,请根据实际情况进行修改。
相关推荐
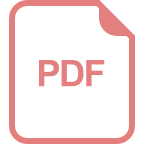
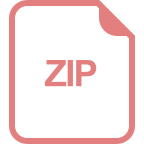
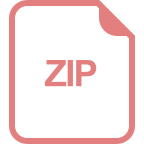














