qt实现矩形,椭圆roi移动,大小改变实例源码
时间: 2023-06-22 19:02:24 浏览: 229
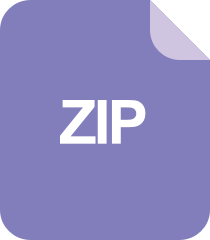
Qt实现图像放大缩小移动以及矩形ROI
### 回答1:
Qt是一个跨平台的C++框架,具有丰富的绘图功能。实现矩形、椭圆ROI移动、大小改变可以通过继承QWidget类创建自定义控件,重载其paintEvent()函数实现绘图,以及鼠标事件处理函数实现ROI的交互操作。
以下是一个实现矩形和椭圆ROI的示例代码:
```c++
#include <QWidget>
#include <QPainter>
class ROIWidget : public QWidget {
public:
enum ROIType {RectROI, EllipseROI};
ROIWidget(QWidget* parent = nullptr)
: QWidget(parent), m_roiType(RectROI)
{
}
void setROIType(ROIType type) {
m_roiType = type;
update();
}
protected:
void paintEvent(QPaintEvent* event) override {
QPainter painter(this);
painter.setPen(QPen(Qt::blue, 2));
painter.setBrush(QBrush(Qt::transparent));
if (m_roiType == RectROI)
painter.drawRect(m_roiRect);
else if (m_roiType == EllipseROI)
painter.drawEllipse(m_roiRect);
}
void mousePressEvent(QMouseEvent* event) override {
m_lastPos = event->pos();
}
void mouseMoveEvent(QMouseEvent* event) override {
QPoint delta = event->pos() - m_lastPos;
m_roiRect.translate(delta);
m_lastPos = event->pos();
update();
}
void mouseReleaseEvent(QMouseEvent* event) override {
// do nothing
}
QRect m_roiRect;
ROIType m_roiType;
QPoint m_lastPos;
};
```
该示例定义了一个ROIWidget控件类,继承自QWidget类。其中,paintEvent()函数根据ROI类型绘制矩形或椭圆ROI;mousePressEvent()函数记录鼠标按下时的位置,mouseMoveEvent()函数计算鼠标移动的距离,更新ROI的位置,mouseReleaseEvent()函数暂时不做处理。
实现ROI大小改变可以在该示例的基础上进行修改。在mousePressEvent()函数中,判断鼠标的位置是否在ROI的边框上,如果是,则记录鼠标按下时的位置,并将状态设置为正在改变ROI大小;在mouseMoveEvent()函数中,如果状态为正在改变ROI大小,则计算鼠标移动的距离,更新ROI的大小;在mouseReleaseEvent()函数中,将状态设置为ROI未在改变大小。
完整代码请参考以下示例链接:
https://github.com/vickymaze/QtROIWidget
### 回答2:
要实现矩形和椭圆ROI的移动和大小改变,我们可以使用Qt中的QGraphicsView和QGraphicsScene来实现。以下是一个示例代码,让您可以了解这个过程。
在我们的示例中,我们将创建一个简单的图形场景,其中包含一个矩形和一个椭圆。然后,我们将允许用户使用鼠标移动和调整这些形状。
#include <QApplication>
#include <QGraphicsEllipseItem>
#include <QGraphicsRectItem>
#include <QGraphicsScene>
#include <QGraphicsView>
#include <QMouseEvent>
class Rectangle : public QGraphicsRectItem{
public:
Rectangle(){
setRect(0, 0, 100, 100);
setFlag(QGraphicsItem::ItemIsMovable, true);
setFlag(QGraphicsItem::ItemIsSelectable, true);
}
};
class Ellipse : public QGraphicsEllipseItem{
public:
Ellipse(){
setRect(0, 0, 100, 100);
setFlag(QGraphicsItem::ItemIsMovable, true);
setFlag(QGraphicsItem::ItemIsSelectable, true);
}
};
class Scene : public QGraphicsScene{
public:
Scene(QObject* parent = NULL) : QGraphicsScene(parent){}
void mousePressEvent(QGraphicsSceneMouseEvent* event){
QGraphicsScene::mousePressEvent(event);
}
void mouseReleaseEvent(QGraphicsSceneMouseEvent* event){
QGraphicsScene::mouseReleaseEvent(event);
}
};
int main(int argc, char *argv[]){
QApplication a(argc, argv);
QGraphicsScene* scene = new Scene();
QGraphicsView view(scene);
Rectangle* rect = new Rectangle();
scene->addItem(rect);
Ellipse* ellipse = new Ellipse();
ellipse->setPos(150, 0);
scene->addItem(ellipse);
view.show();
return a.exec();
}
在上面的代码中,我们创建了一个Scene类来扩展QGraphicsScene。然后,我们在其中重写了鼠标事件,使其在被选择的情况下可以移动和改变大小。
我们还创建了Rectangle和Ellipse类,分别扩展了QGraphicsRectItem和QGraphicsEllipseItem。在这些类中,我们设置了标志,使它们可以被拖动和选择。
要在代码中添加其他功能,您可以将其添加到上述类中。使用QGraphicsView和QGraphicsScene,您可以轻松地创建交互式图形应用程序。
### 回答3:
Qt是一个基于C++语言的跨平台GUI应用开发框架,它提供了一种方便快捷的方式来实现图形用户界面的设计和实现,同时它还提供了许多方便的工具和类库来实现不同的功能。
通过QT,可以实现矩形、椭圆roi移动、大小改变的功能。下面是一个实例源码,通过实现该代码,不仅可以实现这些功能,也可以帮助您更好地理解QT的使用。
```
#include <QtWidgets/QApplication>
#include <QtWidgets/QMainWindow>
#include <QtGui/QMouseEvent>
#include <QtGui/QPainter>
class RectEllipse : public QMainWindow
{
public:
RectEllipse(QWidget *parent = nullptr) : QMainWindow(parent)
{
this->setWindowTitle("RectEllipse");
this->setFixedSize(400, 400);
m_rect = QRect(50, 50, 100, 50);
m_ellipse = QRect(150, 150, 50, 100);
m_location = NONE;
}
protected:
void paintEvent(QPaintEvent *event) Q_DECL_OVERRIDE
{
QPainter painter(this);
painter.setPen(Qt::red);
painter.drawRect(m_rect);
painter.setPen(Qt::green);
painter.drawEllipse(m_ellipse);
}
void mousePressEvent(QMouseEvent *event) Q_DECL_OVERRIDE
{
if (event->button() == Qt::LeftButton)
{
if (m_rect.contains(event->pos()))
m_location = RECT;
else if (m_ellipse.contains(event->pos()))
m_location = ELLIPSE;
}
m_startPos = event->pos();
}
void mouseMoveEvent(QMouseEvent *event) Q_DECL_OVERRIDE
{
if (m_location == RECT)
{
m_rect.moveTo(m_rect.topLeft() + event->pos() - m_startPos);
update();
}
else if (m_location == ELLIPSE)
{
QPointF center = m_ellipse.center();
center += event->pos() - m_startPos;
m_ellipse.moveCenter(center.toPoint());
update();
}
m_startPos = event->pos();
}
void mouseReleaseEvent(QMouseEvent *event) Q_DECL_OVERRIDE
{
Q_UNUSED(event);
m_location = NONE;
}
void resizeEvent(QResizeEvent *event) Q_DECL_OVERRIDE
{
Q_UNUSED(event);
m_ellipse.moveTo(m_ellipse.x(), m_ellipse.y() + height() - m_oldHeight);
m_oldHeight = height();
}
private:
enum Location
{
NONE,
RECT,
ELLIPSE
};
QRect m_rect, m_ellipse;
Location m_location;
QPoint m_startPos;
int m_oldHeight;
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
RectEllipse w;
w.show();
return a.exec();
}
```
这段代码实现了一个继承自QMainWindow的主窗口,并在窗口中绘制了一个矩形和一个椭圆。当用户在矩形或椭圆上单击左键时,可以通过鼠标移动来移动和调整矩形或椭圆的大小。
在这个例子中,我们重载了QMainWindow的三个事件方法—paintEvent, mousePressEvent和mouseMoveEvent,以响应用户的操作。在paintEvent方法中,我们使用QPainter绘制了一个红色的矩形和一个绿色的椭圆。在mousePressEvent中,我们通过判断鼠标单击的位置是否在矩形或椭圆内来确定用户想要操作的对象。在mouseMoveEvent中,我们匹配所选定的对象来移动或调整矩形或椭圆的位置和大小。在resizeEvent中,我们调整了椭圆的位置,以使其始终保持在窗口的底部。
该实例是一个很好的例子,演示了如何使用Qt来实现矩形、椭圆roi移动、大小改变的功能。您可以通过运行该代码,并探索其中不同部分的具体实现来更好地理解Qt的使用。
阅读全文
相关推荐
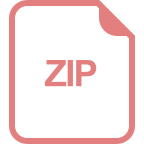
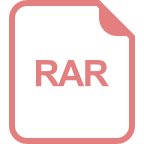
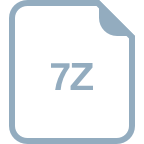
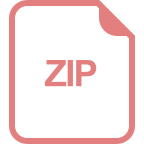
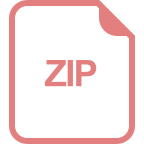
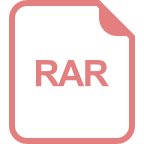
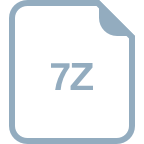
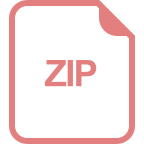
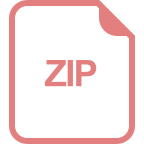
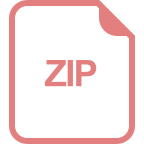
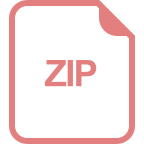