C++编程用贪心策略方法求解 01 背包问题
时间: 2023-08-04 10:32:57 浏览: 100
好的,下面是用C++编写的贪心算法求解01背包问题的代码:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
const int maxn = 1000; // 背包最大容量
struct Good {
int w, v; // w表示物品重量,v表示物品价值
double s; // s表示物品单位价值
} g[maxn];
bool cmp(const Good& g1, const Good& g2) { // 按单位价值从大到小排序
return g1.s > g2.s;
}
int main() {
int n; // 物品数量
int c; // 背包容量
cin >> n >> c;
for (int i = 0; i < n; ++i) {
cin >> g[i].w >> g[i].v;
g[i].s = (double)g[i].v / g[i].w;
}
sort(g, g + n, cmp); // 按单位价值从大到小排序
int ans = 0; // 最大价值
for (int i = 0; i < n; ++i) {
if (c >= g[i].w) { // 当前物品可以放入背包
c -= g[i].w;
ans += g[i].v;
}
}
cout << ans << endl;
return 0;
}
```
这个程序首先输入物品的数量和背包的容量,然后按照物品的单位价值从大到小排序,依次将单位价值最大的物品放入背包中,直到背包不能再放入物品为止。最后输出背包能够装下的最大价值。
阅读全文
相关推荐
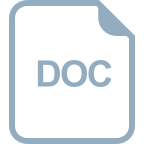
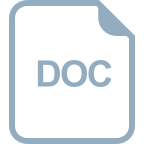
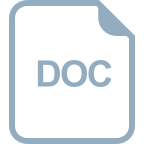
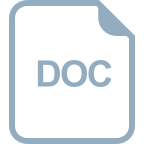
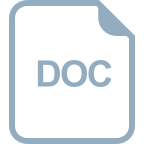






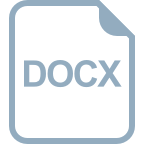
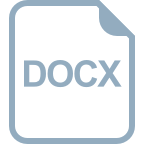




