创建一个学生链表,结构体类型说明如下,从键盘输入至少4个学生的信息,编程计算并输出链表中所有学生的成绩总和及平均分,要求平均分共占10个字符位,且小数占2个字符位。
时间: 2024-12-20 15:15:17 浏览: 6
首先,我们需要创建一个名为`Student`的结构体,包含姓名(字符串)和成绩(浮点数)。然后我们将使用这个结构体定义一个链表节点,接着编写函数来获取用户的输入、添加新节点到链表、计算总成绩和平均分。
```c
#include <stdio.h>
#include <string.h>
// 定义学生结构体
typedef struct {
char name[50];
float score;
struct Student *next;
} Student;
// 添加新学生到链表
void addStudent(Student **head, char name[], float score) {
Student *newNode = (Student *)malloc(sizeof(Student));
strcpy(newNode->name, name);
newNode->score = score;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
Student *current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 计算链表总成绩
float calculateTotalScore(Student *head) {
float total = 0.0;
Student *current = head;
while (current != NULL) {
total += current->score;
current = current->next;
}
return total;
}
// 计算平均分
char* calculateAverageScore(float totalScore, int studentCount) {
float average = totalScore / studentCount;
char buffer[21]; // 包含整数部分、小数点和两位小数
sprintf(buffer, "%.*f", 2, average); // 控制小数点后两位
buffer[strlen(buffer) - 1] = '\0'; // 去掉多余的空格
return buffer;
}
int main() {
Student *head = NULL;
int count = 0;
printf("请输入学生信息(输入'q'结束):\n");
while (true) {
char name[50];
float score;
scanf("%s %f", name, &score);
if (strcmp(name, "q") == 0) break; // 如果输入 'q',退出循环
addStudent(&head, name, score);
count++;
}
if (count == 0) {
printf("没有输入任何学生。\n");
} else {
float total = calculateTotalScore(head);
char *average = calculateAverageScore(total, count);
printf("所有学生的成绩总和为: %.2f\n", total);
printf("平均分为: %s\n", average);
}
return 0;
}
```
阅读全文
相关推荐









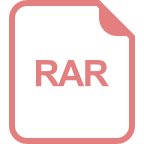








