/** @brief CRC 16 * @param *message : the data that we wanna calculate * @param len : the length of datas * @retval None */ unsigned short Crc_16(unsigned char *luc_Message, unsigned char luc_Len) { unsigned char i, j; unsigned short lus_CrcReg = 0; unsigned short lus_Current; for (i = 0; i < luc_Len; i++){ lus_Current = luc_Message[i] << 8; for (j = 0; j < 8; j++){ if ((short)(lus_CrcReg ^ lus_Current) < 0) lus_CrcReg = (lus_CrcReg << 1) ^ 0x1021; // X^16+X^12+X^5+X^1 else lus_CrcReg <<= 1; lus_Current <<= 1; } } return lus_CrcReg; } 将这串代码装换成python的
时间: 2024-02-14 11:31:55 浏览: 73
以下是将给定的C代码转换为Python代码的等效版本:
def Crc_16(message, length):
crc_reg = 0
for i in range(length):
current = message[i] << 8
for j in range(8):
if (crc_reg ^ current) < 0:
crc_reg = (crc_reg << 1) ^ 0x1021
else:
crc_reg <<= 1
current <<= 1
return crc_reg
# 示例用法
message = [0x12, 0x34, 0x56, 0x78]
length = len(message)
crc_result = Crc_16(message, length)
print(hex(crc_result))
注意:在Python中,不需要指定数据类型,因此将unsigned char替换为整数类型。另外,需要注意Python中的位操作符和C语言中的位操作符略有不同。
相关问题
/** * @brief XOR caculate * @param data * @param len */
XOR计算是一种位运算,它对两个二进制数的对应位进行异或操作。如果两个对应位的值相同,则结果为0;如果两个对应位的值不同,则结果为1。XOR计算常用于数据加密、校验和计算等领域。
在你提供的代码注释中,这段代码是一个XOR计算的函数。它接受两个参数:data和len。data是一个指向二进制数据的指针,len表示数据的长度。该函数的作用是对data指向的数据进行XOR计算。
如果你有任何关于XOR计算或者其他相关问题,请随时提问。
分析以下程序:namespace nav_core { /** * @class BaseGlobalPlanner * @brief Provides an interface for global planners used in navigation. All global planners written as plugins for the navigation stack must adhere to this interface. */ class BaseGlobalPlanner{ public: /** * @brief Given a goal pose in the world, compute a plan * @param start The start pose * @param goal The goal pose * @param plan The plan... filled by the planner * @return True if a valid plan was found, false otherwise */ virtual bool makePlan(const geometry_msgs::PoseStamped& start, const geometry_msgs::PoseStamped& goal, std::vector<geometry_msgs::PoseStamped>& plan) = 0; /** * @brief Given a goal pose in the world, compute a plan * @param start The start pose * @param goal The goal pose * @param plan The plan... filled by the planner * @param cost The plans calculated cost * @return True if a valid plan was found, false otherwise */ virtual bool makePlan(const geometry_msgs::PoseStamped& start, const geometry_msgs::PoseStamped& goal, std::vector<geometry_msgs::PoseStamped>& plan, double& cost) { cost = 0; return makePlan(start, goal, plan); } /** * @brief Initialization function for the BaseGlobalPlanner * @param name The name of this planner * @param costmap_ros A pointer to the ROS wrapper of the costmap to use for planning */ virtual void initialize(std::string name, costmap_2d::Costmap2DROS* costmap_ros) = 0; /** * @brief Virtual destructor for the interface */ virtual ~BaseGlobalPlanner(){} protected: BaseGlobalPlanner(){} }; }; // namespace nav_core #endif // NAV_CORE_BASE_GLOBAL_PLANNER_H
这段代码定义了一个名为 `BaseGlobalPlanner` 的 C++ 类,该类是导航全局规划器的接口,所有为导航堆栈编写的全局规划器插件都必须遵循该接口。该类有三个函数:
1. `makePlan` 函数:给定一个起点和终点的姿态信息,计算出一条连接两点的路径,并将路径填充到一个 `std::vector` 类型的变量中。该函数返回一个布尔值,表示是否成功生成路径。
2. `makePlan` 函数(重载):与第一个函数相似,但是多了一个参数 `cost`,表示计算出的路径的代价。
3. `initialize` 函数:初始化导航全局规划器,其中 `name` 表示规划器的名称, `costmap_ros` 是一个指向 ROS 中表示代价地图的 `Costmap2DROS` 类对象的指针。
此外,该类还有一个虚析构函数。最后,该类被定义在命名空间 `nav_core` 中,且定义了一个宏 `NAV_CORE_BASE_GLOBAL_PLANNER_H`,用于防止头文件重复包含。
阅读全文
相关推荐
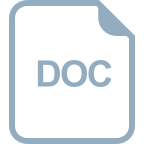
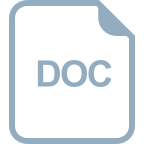
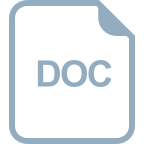





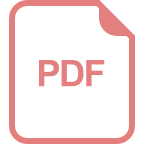
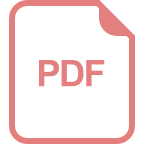
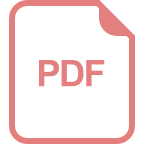
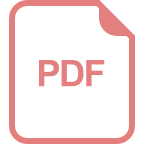
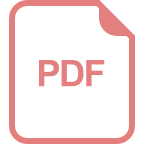
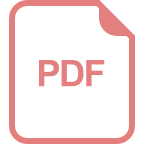


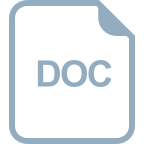