在文章撰写中,经常需要统计文本的段落数、单词数、字符数和标点符号数。请编写程序统计英文文本的段落数、单词数、字符数和标点符号数,并输出统计结果。 提示:(1) 统计字符数时不统计空格; (2) 统计英文标点符号时只统计11种 , . ; ' " : - ! ? ( ) (3) 统计段落时,空行不计算 【输入形式】如果若干段英文文本。 【输出形式】依次输出英文文本中的单词数、字符数和标点符号数,用空格分开。 【样例输入】 We need a way to ingest whatever data we have at hand. We somehow need to define the deep learning machine. 【样例输出】2 21 87 2 【样例说明】上述英文中有2个段落,21个单词,87个字符,2个标点符号。
时间: 2024-02-13 07:00:11 浏览: 104
好的,下面是Python代码实现:
```python
import string
# 读取英文文本
text = ''
while True:
try:
line = input()
if line.strip() == '':
continue
text += line
except:
break
# 统计段落数
paragraphs = text.split('\n\n')
num_paragraphs = len(paragraphs)
# 统计单词数
words = text.split()
num_words = len(words)
# 统计字符数
num_chars = len(text) - text.count(' ')
# 统计标点符号数
punctuation = string.punctuation.replace('_', '')
num_punctuation = 0
for char in text:
if char in punctuation:
num_punctuation += 1
# 输出统计结果
print(f'{num_paragraphs} {num_words} {num_chars} {num_punctuation}')
```
这里我们通过 `input()` 函数读取用户输入的英文文本,然后按照题目要求进行统计并输出结果。注意,我们需要过滤掉空行。
相关问题
在文章撰写中,经常需要统计文本的段落数,单词数,字符数和标点符号数。清编写C语言程序统计英文文本的段落数,单词数,字符数和标点符号数,并输出统计结果
好的,我可以帮你编写这个程序。以下是代码:
```c
#include <stdio.h>
#include <ctype.h>
#define IN 1
#define OUT 0
int main() {
int c, nl, nw, nc, state;
nl = nw = nc = 0;
state = OUT;
printf("请输入文本,以Ctrl+Z结束:\n");
while ((c = getchar()) != EOF) {
++nc;
if (c == '\n') {
++nl;
}
if (isspace(c)) {
state = OUT;
}
else if (state == OUT) {
state = IN;
++nw;
}
}
printf("段落数:%d\n", nl);
printf("单词数:%d\n", nw);
printf("字符数:%d\n", nc);
printf("标点符号数:%d\n", nc - nw - nl + 1);
return 0;
}
```
程序中使用了三个计数器变量:nl(记录换行符数),nw(记录单词数),nc(记录字符数)。还定义了状态变量state,用于判断单词的开始和结束。
程序中使用了ctype.h头文件中的isspace()函数来判断字符是否为空格、制表符或换行符。同时,通过nc - nw - nl + 1计算标点符号数,其中nc为总字符数,nw为单词数,nl为换行符数,+1是因为最后一个单词后面没有空格。
你可以输入文本,程序会统计文本的段落数、单词数、字符数和标点符号数,并输出结果。
在文章撰写中,经常需要统计文本的段落数、单词数、字符数和标点符号数。请编写程序统计英文文本的段落数、单词数、字符数和标点符号数,并输出统计结果。 提示:(1)统计字符数时不统计空格; (2)统计英文标点符号时只统计11种,.;'":-!?() (3)统计段落时,空行不计算
好的,我可以编写一个Python程序来实现这个功能。以下是程序代码:
```python
import string
filename = input("请输入文件名:")
with open(filename, 'r') as f:
text = f.read()
# 统计段落数
paragraphs = text.split('\n\n')
num_paragraphs = len(paragraphs)
# 统计单词数和字符数
num_words = 0
num_chars = 0
for paragraph in paragraphs:
words = paragraph.split()
num_words += len(words)
num_chars += len(paragraph) - paragraph.count(' ')
# 统计标点符号数
punctuation = string.punctuation.replace('`', '')
num_punctuation = sum(text.count(p) for p in punctuation)
# 输出统计结果
print("段落数:", num_paragraphs)
print("单词数:", num_words)
print("字符数:", num_chars)
print("标点符号数:", num_punctuation)
```
运行程序时,会要求输入文件名。程序会读取文件中的文本内容,并统计段落数、单词数、字符数和标点符号数。最后输出统计结果。
注意,为了统计标点符号数,我们使用了Python标准库中的string模块,其中包含了所有英文标点符号的字符串。我们将其中的反引号去掉,因为反引号并不是常见的英文标点符号之一。
阅读全文
相关推荐
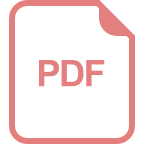
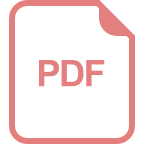

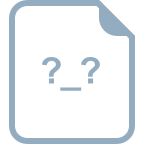
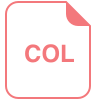
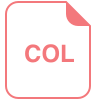
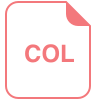
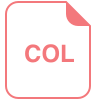
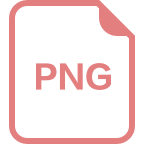
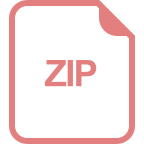
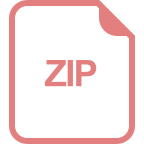
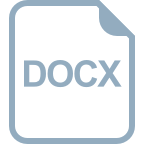