dht11温湿度传感器stm32f103c8t6 代码
时间: 2023-08-31 08:10:30 浏览: 60
下面是使用STM32F103C8T6和DHT11温湿度传感器的示例代码:
```c
#include "stm32f10x.h"
#include <stdio.h>
#define DHT11_PIN GPIO_Pin_0
#define DHT11_PORT GPIOB
void Delay_us(uint32_t microseconds)
{
microseconds *= (SystemCoreClock / 1000000) / 5;
while (microseconds--)
{
__NOP();
__NOP();
__NOP();
__NOP();
__NOP();
}
}
void DHT11_Start(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
GPIO_SetBits(DHT11_PORT, DHT11_PIN);
Delay_us(1000);
GPIO_ResetBits(DHT11_PORT, DHT11_PIN);
Delay_us(18000);
GPIO_SetBits(DHT11_PORT, DHT11_PIN);
Delay_us(40);
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
}
void DHT11_Check_Response(void)
{
while (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN))
;
while (!GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN))
;
while (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN))
;
}
uint8_t DHT11_Read(void)
{
uint8_t i, j;
uint8_t data = 0;
for (j = 0; j < 8; j++)
{
while (!GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN))
;
Delay_us(40);
if (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN))
{
data |= (1 << (7 - j));
}
while (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN))
;
}
return data;
}
int main(void)
{
uint8_t Humidity, Temperature;
char buffer[20];
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
while (1)
{
DHT11_Start();
DHT11_Check_Response();
Humidity = DHT11_Read();
DHT11_Read();
Temperature = DHT11_Read();
DHT11_Read();
DHT11_Read();
sprintf(buffer, "Humidity: %d.%d %%\n", Humidity / 10, Humidity % 10);
printf(buffer);
sprintf(buffer, "Temperature: %d.%d C\n\n", Temperature / 10, Temperature % 10);
printf(buffer);
Delay_us(2000000);
}
}
```
在这个例子中,使用PB0作为DHT11的数据引脚。代码通过设置GPIO引脚的输入和输出模式来控制DHT11的通信。它使用延迟函数来生成所需的时间间隔,并从DHT11读取温度和湿度数据。最后,将温度和湿度值格式化为字符串并打印到终端上。
请注意,此代码仅供参考,您可能需要根据自己的硬件配置进行修改。
相关推荐
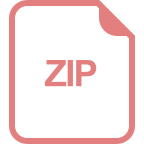
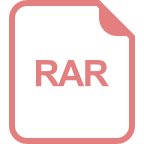














