c# UDPServer
时间: 2023-12-13 18:34:10 浏览: 77
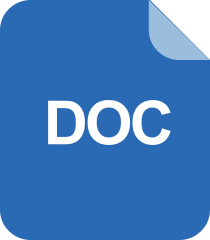
UDP Server
以下是C#实现的UDP服务器端代码示例:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
using System.Text;
public class UDPServer
{
private const int listenPort = 11000;
public static void Main()
{
UdpClient listener = new UdpClient(listenPort);
try
{
while (true)
{
Console.WriteLine("Waiting for broadcast");
IPEndPoint groupEP = new IPEndPoint(IPAddress.Any, listenPort);
byte[] bytes = listener.Receive(ref groupEP);
Console.WriteLine("Received broadcast from {0} :\n {1}\n",
groupEP.ToString(),
Encoding.ASCII.GetString(bytes, 0, bytes.Length));
}
}
catch (SocketException e)
{
Console.WriteLine(e);
}
finally
{
listener.Close();
}
}
}
```
以上代码创建了一个UDP服务器,监听指定端口(11000),并在控制台输出接收到的消息。你可以根据需要修改端口号和输出方式。
以下是C#实现的UDP客户端代码示例:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
using System.Text;
public class UDPClient
{
private const int listenPort = 11000;
public static void Main(string[] args)
{
UdpClient client = new UdpClient();
try
{
IPAddress broadcast = IPAddress.Parse("192.168.1.255");
byte[] sendBytes = Encoding.ASCII.GetBytes("Hello from UDPClient");
client.EnableBroadcast = true;
client.Send(sendBytes, sendBytes.Length, new IPEndPoint(broadcast, listenPort));
}
catch (Exception e)
{
Console.WriteLine(e.ToString());
}
finally
{
client.Close();
}
}
}
```
以上代码创建了一个UDP客户端,向指定IP地址(192.168.1.255)发送消息,并在控制台输出发送结果。你可以根据需要修改IP地址和消息内容。
阅读全文
相关推荐

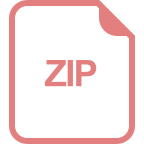
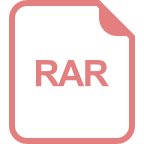
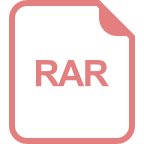
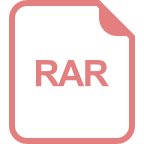
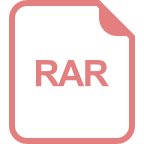
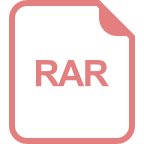
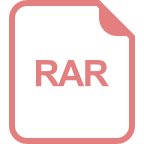
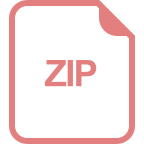
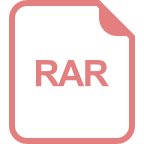
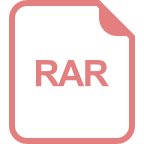
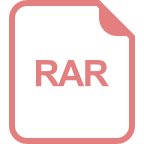


