c# udpclient
时间: 2023-07-31 22:05:29 浏览: 76
C#中使用UDP协议通信可以使用UDPClient类。下面是一个简单的UDPClient示例:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
using System.Text;
class Program
{
static void Main(string[] args)
{
// 设置服务器IP和端口号
IPAddress serverIP = IPAddress.Parse("127.0.0.1");
int serverPort = 8080;
// 创建UDPClient实例
UdpClient udpClient = new UdpClient();
try
{
// 发送数据到服务器
byte[] sendBytes = Encoding.ASCII.GetBytes("Hello, server!");
udpClient.Send(sendBytes, sendBytes.Length, new IPEndPoint(serverIP, serverPort));
// 接收服务器返回的数据
IPEndPoint remoteEP = null;
byte[] receiveBytes = udpClient.Receive(ref remoteEP);
string receiveString = Encoding.ASCII.GetString(receiveBytes);
Console.WriteLine("Received from {0}: {1}", remoteEP.ToString(), receiveString);
}
catch (Exception e)
{
Console.WriteLine(e.ToString());
}
finally
{
// 关闭UDPClient
udpClient.Close();
}
Console.ReadLine();
}
}
```
在这个示例中,我们创建了一个UDPClient实例,并使用Send方法发送数据到服务器。然后使用Receive方法接收服务器返回的数据。注意,我们在接收数据之前需要先指定一个远程终结点,用于指定接收数据的来源。最后,我们关闭了UDPClient实例。
相关推荐
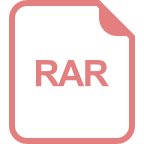














