简单的扫雷C++代码。
时间: 2024-09-10 11:11:42 浏览: 86
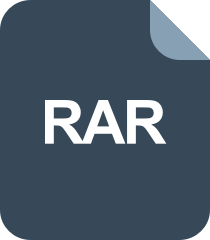
C++扫雷游戏最全源代码

简单的扫雷游戏C++代码实现通常包括以下几个部分:游戏初始化、游戏主循环、雷区生成、玩家操作处理、胜负判定等。下面给出一个简化的扫雷游戏代码框架,以供参考:
```cpp
#include <iostream>
#include <vector>
#include <ctime>
#include <cstdlib>
using namespace std;
// 游戏设置
const int WIDTH = 10; // 雷区宽度
const int HEIGHT = 10; // 雷区高度
const int MINES = 20; // 雷的数量
// 游戏状态
bool gameOver;
vector<vector<char>> board(HEIGHT, vector<char>(WIDTH, ' ')); // 游戏雷区
vector<vector<bool>> revealed(HEIGHT, vector<bool>(WIDTH, false)); // 是否已揭露
// 初始化游戏
void init() {
gameOver = false;
// 随机放置雷
srand(time(nullptr));
int minesPlaced = 0;
while (minesPlaced < MINES) {
int x = rand() % WIDTH;
int y = rand() % HEIGHT;
if (board[y][x] != '*') {
board[y][x] = '*';
minesPlaced++;
}
}
}
// 打印雷区
void printBoard() {
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
if (revealed[y][x]) {
cout << board[y][x] << " ";
} else {
cout << ". ";
}
}
cout << endl;
}
}
// 主函数
int main() {
init();
printBoard();
// 游戏主循环
while (!gameOver) {
// 玩家输入
int x, y;
cout << "Enter X and Y coordinates: ";
cin >> x >> y;
if (x < 0 || x >= WIDTH || y < 0 || y >= HEIGHT) {
cout << "Invalid coordinates! Try again." << endl;
continue;
}
// 检查是否踩雷
if (board[y][x] == '*') {
gameOver = true;
cout << "Game Over! You hit a mine!" << endl;
break;
}
// 揭露当前位置
revealed[y][x] = true;
// 这里可以添加一个揭露周围雷数的逻辑
// 更新雷区显示
printBoard();
}
return 0;
}
```
这段代码是一个非常基础的扫雷游戏的雏形,它只包含了游戏初始化、简单的雷区生成、玩家输入以及胜负判断。在实际的游戏开发中,你还需要添加更多的功能,比如递归揭露空白区域、计算周围雷数、标记疑似雷区等。而且为了提供更好的用户体验,还应该考虑添加字符或图形界面,并对用户输入进行更严谨的检查。
阅读全文
相关推荐
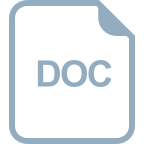

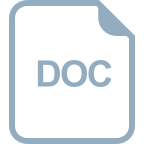
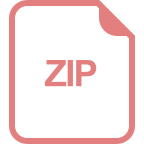
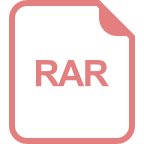
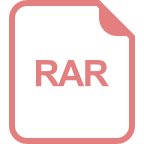
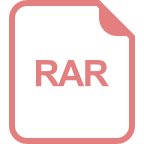
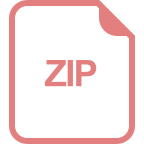
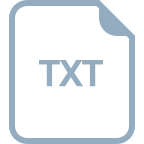

