图书管理系统(代码生成)C语言
时间: 2023-11-23 09:56:08 浏览: 248
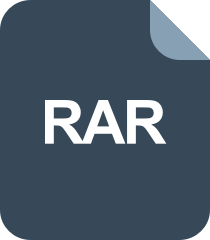
图书管理系统C语言
以下是一个简单的图书管理系统的C语言代码示例,包括添加新书、修改书籍信息、删除书籍和图书查询功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100 // 最大书籍数量
// 书籍结构体
typedef struct {
char title[50]; // 书名
char author[50]; // 作者
char publisher[50]; // 出版社
char isbn[20]; // ISBN号
int stock; // 库存数量
} Book;
// 全局变量
Book books[MAX_BOOKS]; // 存储所有书籍的数组
int num_books = 0; // 当前书籍数量
// 添加新书
void add_book() {
if (num_books >= MAX_BOOKS) {
printf("Error: too many books.\n");
return;
}
Book new_book;
printf("Enter book title: ");
scanf("%s", new_book.title);
printf("Enter book author: ");
scanf("%s", new_book.author);
printf("Enter book publisher: ");
scanf("%s", new_book.publisher);
printf("Enter book ISBN: ");
scanf("%s", new_book.isbn);
printf("Enter book stock: ");
scanf("%d", &new_book.stock);
books[num_books] = new_book;
num_books++;
printf("Book added successfully.\n");
}
// 修改书籍信息
void modify_book() {
char isbn[20];
printf("Enter book ISBN: ");
scanf("%s", isbn);
int i;
for (i = 0; i < num_books; i++) {
if (strcmp(books[i].isbn, isbn) == 0) {
printf("Enter new stock: ");
scanf("%d", &books[i].stock);
printf("Book modified successfully.\n");
return;
}
}
printf("Error: book not found.\n");
}
// 删除书籍
void delete_book() {
char isbn[20];
printf("Enter book ISBN: ");
scanf("%s", isbn);
int i;
for (i = 0; i < num_books; i++) {
if (strcmp(books[i].isbn, isbn) == 0) {
int j;
for (j = i; j < num_books - 1; j++) {
books[j] = books[j + 1];
}
num_books--;
printf("Book deleted successfully.\n");
return;
}
}
printf("Error: book not found.\n");
}
// 图书查询功能
void search_books() {
char keyword[50];
printf("Enter search keyword: ");
scanf("%s", keyword);
int i;
for (i = 0; i < num_books; i++) {
if (strstr(books[i].title, keyword) != NULL ||
strstr(books[i].author, keyword) != NULL ||
strstr(books[i].publisher, keyword) != NULL ||
strstr(books[i].isbn, keyword) != NULL) {
printf("Title: %s\n", books[i].title);
printf("Author: %s\n", books[i].author);
printf("Publisher: %s\n", books[i].publisher);
printf("ISBN: %s\n", books[i].isbn);
printf("Stock: %d\n", books[i].stock);
printf("\n");
}
}
}
int main() {
int choice;
while (1) {
printf("1. Add book\n");
printf("2. Modify book\n");
printf("3. Delete book\n");
printf("4. Search books\n");
printf("5. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book();
break;
case 2:
modify_book();
break;
case 3:
delete_book();
break;
case 4:
search_books();
break;
case 5:
exit(0);
default:
printf("Invalid choice.\n");
}
}
return 0;
}
```
阅读全文
相关推荐
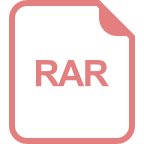
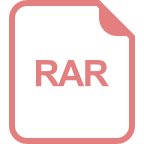
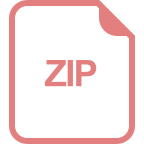
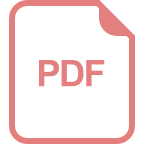
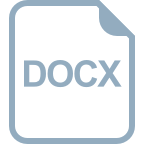
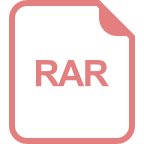
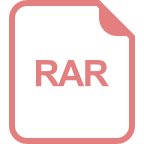
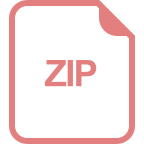
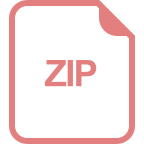
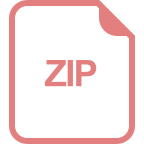
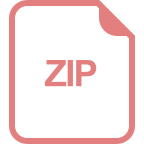
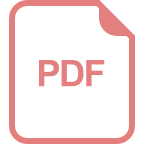
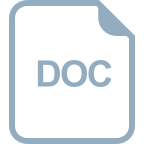



