用Java代码实现通过网络提供的api,实现天气的实时查询,输入城市名称,显示城市的天气情况。 建议:采用“和风天气平台”提供的API. 和风天气平台 https://dev.qweather.com/
时间: 2024-12-13 09:18:35 浏览: 4
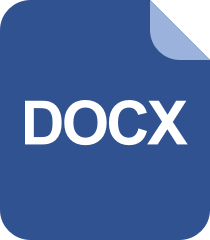
安卓天气预报
在Java中,你可以使用HttpURLConnection或者第三方库如OkHttp或Spring WebClient来访问和风天气平台的API获取天气信息。这里是一个基本的例子,假设你已经有了API密钥:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class WeatherQuery {
private static final String API_KEY = "your_qweather_api_key";
private static final String BASE_URL = "http://wthrcdn.etouch.cn/weather_mini?cityid=";
public static void main(String[] args) {
try {
// 输入的城市名,比如北京
String city = "010";
// 构建完整的URL
URL url = new URL(BASE_URL + city + "&key=" + API_KEY);
// 打开连接并获取HTTP响应码
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
// 读取并解析返回的JSON数据
StringBuilder responseBuilder = new StringBuilder();
String inputLine;
while ((inputLine = in.readLine()) != null) {
responseBuilder.append(inputLine);
}
in.close();
// 示例,处理JSON数据获取温度、描述等信息
// 这里需要根据实际的API文档来解析数据
parseWeatherData(responseBuilder.toString());
} else {
System.out.println("Error: " + responseCode);
}
} catch (Exception e) {
e.printStackTrace();
}
}
private static void parseWeatherData(String response) {
// 使用正则表达式或其他库如Jackson、Gson来解析天气数据
// 例如:
// Map<String, Object> weatherInfo = JSON.parseObject(response, new TypeReference<Map<String, Object>>() {});
// double temperature = Double.parseDouble(weatherInfo.get("temperature").toString());
// String description = weatherInfo.get("description").toString();
// 输出结果
// System.out.println("Temperature: " + temperature + ", Description: " + description);
}
}
```
记得替换`"your_qweather_api_key"`为你从和风天气平台获得的实际API密钥。这个例子只是一个基础示例,实际项目中还需要处理异常、添加错误处理,并可能需要处理更复杂的JSON结构。
阅读全文
相关推荐
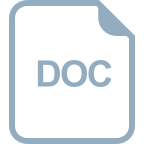
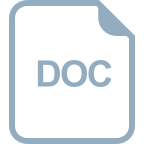

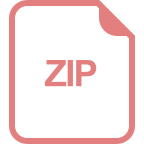
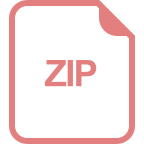
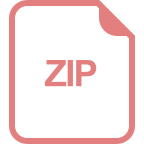
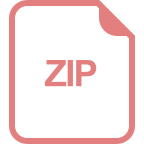
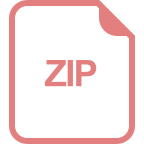
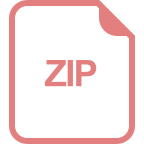
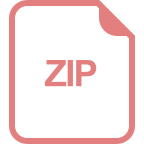
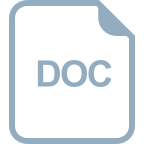
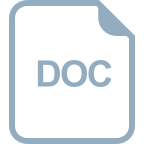
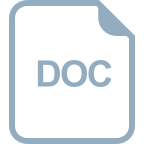
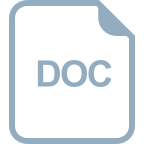
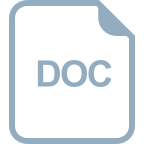
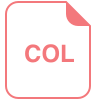
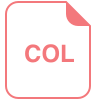
