c++ string insert
时间: 2023-08-17 17:07:34 浏览: 76
在C++中,可以使用`insert()`函数向字符串中插入字符或子字符串。`insert()`函数的原型如下:
```cpp
string insert (size_t pos, const string& str);
```
其中,`pos`表示要插入的位置,`str`表示要插入的字符串。
下面是一个示例代码,演示如何使用`insert()`函数向字符串中插入字符或子字符串:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello World!";
cout << "原始字符串:" << str << endl;
// 在位置5处插入字符','
str.insert(5, ",");
cout << "插入逗号后的字符串:" << str << endl;
// 在末尾插入子字符串" Welcome"
str.insert(str.length(), " Welcome");
cout << "插入欢迎语后的字符串:" << str << endl;
return 0;
}
```
输出结果为:
```
原始字符串:Hello World!
插入逗号后的字符串:Hello, World!
插入欢迎语后的字符串:Hello, World! Welcome
```
希望能帮到你!如有任何问题,请随时提问。
相关问题
c++string insert、
C++ 中的 `string` 类型提供了 `insert` 函数用于在指定位置插入内容。该函数的语法如下:
```cpp
string& insert(size_t pos, const string& str);
string& insert(size_t pos, const char* s);
string& insert(size_t pos, const char* s, size_t n);
string& insert(size_t pos, size_t n, char c);
iterator insert(const_iterator p, size_t n, char c);
iterator insert(const_iterator p, char c);
template<class InputIterator>
void insert(iterator p, InputIterator first, InputIterator last);
```
其中:
- `pos` 表示要插入的位置,从 0 开始计数;
- `str` 表示要插入的字符串;
- `s` 表示要插入的 C 风格字符串;
- `n` 表示要插入的 C 风格字符串的长度;
- `c` 表示要插入的字符;
- `first` 和 `last` 表示要插入的字符范围;
- `p` 表示要插入的位置的迭代器。
下面是一些使用示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello world";
str.insert(5, "my"); // 在第 5 个字符后面插入 "my"
cout << str << endl; // 输出 "hello my world"
str.insert(0, "hi, "); // 在开头插入 "hi, "
cout << str << endl; // 输出 "hi, hello my world"
str.insert(14, 2, '!'); // 在第 14 个字符后面插入 2 个感叹号
cout << str << endl; // 输出 "hi, hello my world!!"
str.insert(str.begin() + 3, ' '); // 在第 3 个字符前面插入一个空格
cout << str << endl; // 输出 "hi, hello my world!!"
str.insert(str.begin() + 6, str.begin() + 10, str.end()); // 将第 6 个字符前面插入从第 10 个字符到结尾的字符
cout << str << endl; // 输出 "hi, my world!!hello my world!!"
return 0;
}
```
c++ string insert函数用法
C++中的string类提供了insert函数,用于在指定位置插入字符串或字符。其用法如下:
```cpp
string insert (size_t pos, const string& str);
string insert (size_t pos, const char* s);
string insert (size_t pos, const char* s, size_t n);
string insert (size_t pos, size_t n, char c);
```
其中,pos表示插入的位置,str表示要插入的字符串,s表示要插入的字符数组,n表示要插入的字符个数,c表示要插入的字符。
示例代码如下:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::cout << "原始字符串:" << str << std::endl;
// 在位置5插入字符串" C++"
str.insert(5, " C++");
std::cout << "插入后的字符串:" << str << std::endl;
// 在位置0插入字符数组"Hi"
str.insert(0, "Hi");
std::cout << "再次插入后的字符串:" << str << std::endl;
// 在位置13插入3个字符'!'
str.insert(13, 3, '!');
std::cout << "最终插入后的字符串:" << str << std::endl;
return 0;
}
```
输出结果为:
```
原始字符串:Hello, world!
插入后的字符串:Hello C++, world!
再次插入后的字符串:HiHello C++, world!
最终插入后的字符串:HiHello C++, world!!!
```
相关推荐
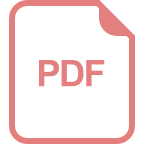
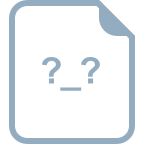











