c++中string的insert
时间: 2023-10-11 12:04:29 浏览: 34
在C语言中,没有原生的string类型,但可以使用字符数组来模拟字符串。要在字符数组中插入字符串,可以使用strcpy和strcat函数。
例如,假设有一个字符数组str,需要在第3个位置插入字符串"hello",可以按照以下步骤进行:
1. 将第3个位置后的字符向后移动,让出空间给插入的字符串。
```c
memmove(str+3+strlen("hello"), str+3, strlen(str)-3+1);
```
2. 将字符串"hello"复制到第3个位置。
```c
strcpy(str+3, "hello");
```
完整代码如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[20] = "world";
printf("before insert: %s\n", str);
memmove(str+3+strlen("hello"), str+3, strlen(str)-3+1);
strcpy(str+3, "hello");
printf("after insert: %s\n", str);
return 0;
}
```
输出:
```
before insert: world
after insert: worhellold
```
相关问题
c++中string的insert用法
在C++中,可以通过string的insert方法来插入字符或子字符串。insert方法接受两个参数:第一个参数是插入位置的迭代器,第二个参数是要插入的字符或子字符串。
例如,将一个字符插入到string对象的第三个位置:
```
string str = "hello";
str.insert(str.begin()+2, 'l');
```
将一个子字符串插入到string对象的第五个位置:
```
string str = "hello";
string sub = "world";
str.insert(4, sub);
```
c++ string insert
在C++中,可以使用`insert()`函数向字符串中插入字符或子字符串。`insert()`函数的原型如下:
```cpp
string insert (size_t pos, const string& str);
```
其中,`pos`表示要插入的位置,`str`表示要插入的字符串。
下面是一个示例代码,演示如何使用`insert()`函数向字符串中插入字符或子字符串:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello World!";
cout << "原始字符串:" << str << endl;
// 在位置5处插入字符','
str.insert(5, ",");
cout << "插入逗号后的字符串:" << str << endl;
// 在末尾插入子字符串" Welcome"
str.insert(str.length(), " Welcome");
cout << "插入欢迎语后的字符串:" << str << endl;
return 0;
}
```
输出结果为:
```
原始字符串:Hello World!
插入逗号后的字符串:Hello, World!
插入欢迎语后的字符串:Hello, World! Welcome
```
希望能帮到你!如有任何问题,请随时提问。
相关推荐
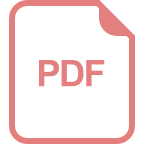
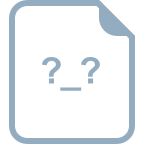
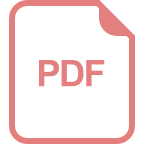












