数字图像处理车牌识别源码
时间: 2023-09-08 18:07:15 浏览: 73
您好!对于数字图像处理中的车牌识别,以下是一个简单的示例代码:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 加载图像
image = cv2.imread('car_plate.jpg')
# 将图像转换为灰度图
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 进行图像平滑处理
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# 应用Canny边缘检测算法
edges = cv2.Canny(blur, 50, 150)
# 对图像进行膨胀操作,以填充车牌区域内的空洞
dilated = cv2.dilate(edges, None, iterations=2)
# 查找并筛选车牌区域的轮廓
contours, _ = cv2.findContours(dilated.copy(), cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
contours = sorted(contours, key=cv2.contourArea, reverse=True)[:10]
plate = None
for contour in contours:
# 计算轮廓的周长
perimeter = cv2.arcLength(contour, True)
# 近似轮廓
approx = cv2.approxPolyDP(contour, 0.02 * perimeter, True)
# 如果近似轮廓有四个顶点,则认为是车牌区域
if len(approx) == 4:
plate = approx
break
# 提取车牌区域并进行透视变换
if plate is not None:
mask = np.zeros(gray.shape, np.uint8)
cv2.drawContours(mask, [plate], 0, 255, -1)
masked = cv2.bitwise_and(image, image, mask=mask)
(x, y) = np.where(mask == 255)
(top_x, top_y) = (np.min(x), np.min(y))
(bottom_x, bottom_y) = (np.max(x), np.max(y))
cropped = gray[top_x:bottom_x+1, top_y:bottom_y+1]
# 显示结果
plt.imshow(cv2.cvtColor(cropped, cv2.COLOR_BGR2RGB))
plt.axis('off')
plt.show()
```
请注意,这只是一个简单的示例代码,仅用于说明基本的车牌识别过程。实际应用中可能需要更复杂的算法和步骤来提高准确性和稳定性。此外,您需要将代码中的'car_plate.jpg'替换为您自己的车牌图像路径。
希望对您有所帮助!如有任何其他问题,请随时提问。
相关推荐
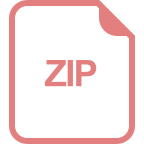
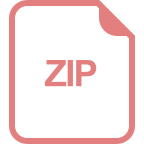
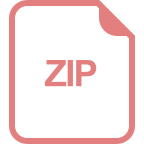














