定义一个Student 数组,其中保存学生的基本信息,包括姓名、学号、性别,还分别保存三门课程的成绩及三门课程对应的学分。试编程计算这三门课程的学分积,并按学分积的降幂进行排序,输出排序后的结果。
时间: 2024-10-12 22:08:46 浏览: 29
首先,我们可以定义一个`Student`类,包含姓名、学号、性别、三门课程的成绩和学分。然后,我们可以创建一个`Student`数组,接着编写一个方法来计算学分积,再使用`Collections.sort()`方法按照学分积进行降序排序。以下是相关的Java代码示例:
```java
import java.util.Arrays;
import java.util.Collections;
import java.util.Comparator;
class Student {
String name;
int studentId;
String gender;
double[] scores; // 课程成绩,例如[语文成绩, 数学成绩, 英语成绩]
int[] credits; // 对应课程学分
// 构造函数和其他方法省略...
// 计算学分积
double calculateCreditProduct() {
double product = 1;
for (int i = 0; i < scores.length; i++) {
product *= scores[i] * credits[i];
}
return product;
}
}
public class Main {
public static void main(String[] args) {
// 创建Student对象数组
Student[] students = new Student[3];
students[0] = new Student("张三", 1001, "男", new double[]{85, 90, 92}, new int[]{4, 3, 3});
students[1] = new Student("李四", 1002, "女", new double[]{90, 95, 96}, new int[]{3, 3, 4});
students[2] = new Student("王五", 1003, "男", new double[]{88, 93, 94}, new int[]{3, 4, 3});
// 计算学分积并排序
Arrays.sort(students, Comparator.comparing(Student::calculateCreditProduct).reversed());
// 输出排序后的结果
for (Student s : students) {
System.out.println("Name: " + s.name + ", Total Credit Product: " + s.calculateCreditProduct());
}
}
}
```
阅读全文
相关推荐
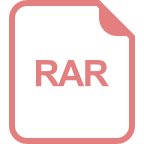
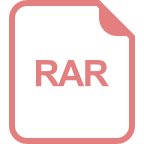
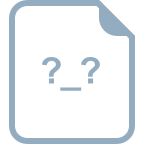















