java定义一个 Student 数组,其中保存学生的基本信息,包括姓名、学号、性别, 还分别原存三门课程的成绩及三门课程对应的学分。试编程计算这三门课程的学分积,并按学分移的降幂进行排序,输出排序后的结果
时间: 2024-11-10 18:26:11 浏览: 38
在Java中,你可以定义一个`Student`类来存储学生的个人信息以及成绩和学分,然后创建一个`Student`数组来存储多个学生的信息。首先,我们需要定义`Student`类:
```java
public class Student {
private String name;
private int studentID;
private char gender;
private double[] grades; // 成绩数组,假设每门课都是1分制,例如0-100
private int[] credits; // 学分数组
// 构造函数、getter和setter方法省略...
// 计算学分积的方法
public double calculateTotalCreditProduct() {
double totalProduct = 1.0;
for (int i = 0; i < grades.length; i++) {
totalProduct *= grades[i] * credits[i];
}
return totalProduct;
}
// 按学分降序排列的方法
public void sortStudentsByDescendingCredit() {
Arrays.sort(this, Comparator.comparingDouble(Student::calculateTotalCreditProduct).reversed());
}
// 输出排序后的结果
@Override
public String toString() {
return "Name: " + name + ", ID: " + studentID + ", Gender: " + gender +
", Grades: [" + Arrays.toString(grades) + "], Credits: [" + Arrays.toString(credits) + "]";
}
}
```
接下来,你可以创建一个`Student`数组并进行操作:
```java
public static void main(String[] args) {
Student[] students = new Student[3]; // 创建3个学生实例
students[0] = new Student("张三", 1001, 'M', new double[]{85, 90, 95}, new int[]{4, 3, 2});
students[1] = new Student("李四", 1002, 'F', new double[]{75, 80, 88}, new int[]{3, 2, 4});
students[2] = new Student("王五", 1003, 'M', new double[]{90, 92, 86}, new int[]{2, 3, 3});
for (Student student : students) {
System.out.println("Before sorting: " + student);
}
for (Student student : students) {
student.sortStudentsByDescendingCredit();
}
System.out.println("\nAfter sorting by descending credit:");
for (Student student : students) {
System.out.println(student);
}
}
```
这个程序首先会打印未排序的学生信息,然后对每个学生按照学分积降序进行排序,最后再次打印排序后的结果。
阅读全文
相关推荐
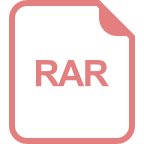
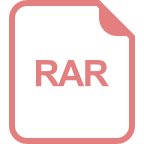
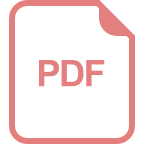















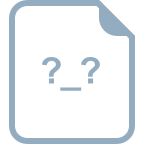
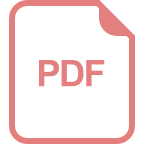