C 语言代码,能够根据用户输入的可执行程序名称,创建一个线程来运行该程序。
时间: 2024-09-30 20:04:37 浏览: 39
在C语言中,你可以使用POSIX线程库(pthreads)来实现这个功能。首先,你需要获取用户的输入,然后创建一个新的线程来执行指定的程序。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
// 定义一个函数作为新线程的目标
void* run_program(void* arg) {
char* program_name = (char*)arg;
FILE* child_process = popen(program_name, "r"); // 打开进程管道
if (!child_process) {
perror("Error running program");
return NULL;
}
// 读取并处理子进程的输出
char buffer[1024];
while (fgets(buffer, sizeof(buffer), child_process)) {
printf("%s", buffer);
}
pclose(child_process); // 关闭子进程
return NULL;
}
int main() {
pthread_t thread_id; // 线程标识符
// 用户输入的程序名称
char* program_name = malloc(sizeof(char) * 100);
printf("Enter the name of the executable program: ");
fgets(program_name, 100, stdin);
program_name[strcspn(program_name, "\n")] = '\0'; // 移除换行符
// 创建新的线程
if (pthread_create(&thread_id, NULL, run_program, program_name) != 0) {
perror("Error creating thread");
exit(EXIT_FAILURE);
}
// 主线程继续执行,而子线程会在run_program函数中启动指定的程序
pthread_join(thread_id, NULL); // 等待线程完成
free(program_name); // 释放内存
return 0;
}
```
阅读全文
相关推荐


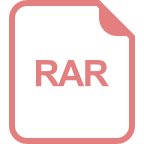















