线程创建。下面是一个简单的多线程示例,编译运行该程序,并观察运行结果,理解程序功能。 /* ex1.c*/
时间: 2024-11-18 17:18:34 浏览: 16
### 线程创建示例
#### 代码
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <unistd.h>
void* thread(void* arg) {
int i;
for(i = 0; i < 3; i++) {
printf(" ------------------This is a pthread.\n");
}
return NULL;
}
int main(void) {
pthread_t id;
int i, ret;
ret = pthread_create(&id, NULL, thread, NULL);
if (ret != 0) {
printf("Create pthread error!\n");
exit(1);
}
for(i = 0; i < 3; i++) {
printf("This is the main pthread.\n");
}
pthread_join(id, NULL);
return 0;
}
```
#### 编译命令
因为 `pthread` 库不是 Linux 系统的标准库,所以需要在编译时链接 `pthread` 库。编译命令如下:
```sh
gcc ex1.c -lpthread -o ex1.o
```
#### 运行命令
```sh
./ex1.o
```
#### 运行结果
运行程序后,可能会看到类似以下的输出:
```
This is the main pthread.
This is the main pthread.
This is the main pthread.
------------------This is a pthread.
------------------This is a pthread.
------------------This is a pthread.
```
#### 解释
- **主函数** (`main`) 创建了一个新的线程 `id`,并将 `thread` 函数作为新线程的入口点。
- **新线程** (`thread`) 执行一个循环,打印三次 "------------------This is a pthread."。
- **主函数** 在创建新线程后,继续执行自己的循环,打印三次 "This is the main pthread."。
- **线程同步** 使用 `pthread_join` 确保主线程等待新线程结束后再退出。
#### 思考题
1. **程序运行后,进程 `thread` 中有几个线程存在?**
- 进程 `thread` 中有两个线程:一个是主线程,另一个是由 `pthread_create` 创建的新线程。
2. **将代码中两处 `for(i=0;i<3;i++)` 改为 `while(1)`,由循环3次变成无穷多次。观察程序运行结果,为什么前后多次运行结果不一样?**
- 修改后的代码会导致两个线程无限循环,主线程和新线程会不断交替执行。由于操作系统的调度机制,每次运行的结果可能会有所不同,具体表现为输出顺序的不同。
3. **试在本程序中再添加一个或多个其他线程,观测运行结果,充分理解多线程的含义。**
- 可以在 `main` 函数中再创建一个或多个线程,每个线程执行不同的任务,观察它们之间的交互和调度情况。这有助于理解多线程环境下的并发执行和资源竞争。
阅读全文
相关推荐
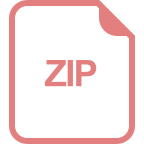
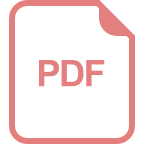
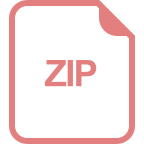
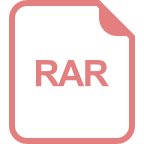
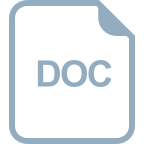
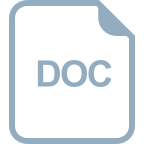
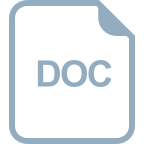
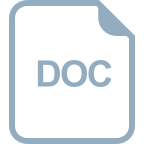
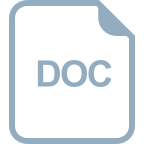
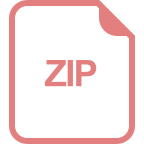
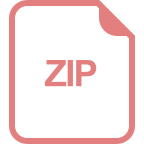
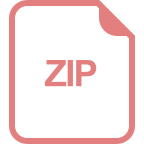
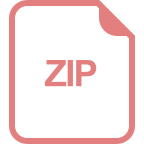