new GeoJSON().readFeatures Cannot read properties of undefined (reading '0')
时间: 2024-11-29 22:15:09 浏览: 24
这个错误提示 "Cannot read properties of undefined (reading '0')" 意味着你在尝试访问 `GeoJSON()` 对象的一个不存在的属性或方法,而这个属性或方法应该是指向某个数据的第一个元素(索引为0)。`readFeatures` 通常是在处理GeoJSON数据时用于读取特征集合的方法,如果 `GeoJSON()` 对象还没有正确初始化,或者 `features` 数组为空,就可能导致这样的错误。
要解决这个问题,你需要检查以下几个步骤:
1. 确保你已经成功创建了一个 GeoJSON 对象实例,并且它的构造函数已经被正确地调用。
2. 检查 `readFeatures` 执行之前,`GeoJSON` 对象是否包含了预期的地理数据,特别是 `features` 属性。
3. 如果是从服务器获取的数据,确保数据已加载并解析完成后再调用 `readFeatures`。
修复代码示例(假设 `geoData` 是你从服务器获取的数据):
```javascript
const geojson = new GeoJSON();
if (geoData && geoData.features) {
const features = geojson.readFeatures(geoData);
// 现在可以安全地操作 features
} else {
console.error('GeoJSON data is not defined or empty.');
}
```
相关问题
new GeoJSON().readFeatures(json) Cannot read properties of undefined (reading ‘0’)
当你看到这样的错误 `new GeoJSON().readFeatures(json) Cannot read properties of undefined (reading '0')`,这通常发生在使用JavaScript的geojson-js库处理GeoJSON数据时。`readFeatures()` 是这个库中用于解析GeoJSON数组并返回FeatureCollection对象的方法。
这个错误意味着你在尝试访问 `json` 对象的一个不存在的属性,也就是 `json[0]` 或者 json 的第一个元素。可能的原因有:
1. `json` 变量可能是 null、undefined 或者不是有效的GeoJSON格式。确保你在调用 `readFeatures()` 之前,`json` 已经被正确地解析并且是一个包含GeoJSON特征的数组。
2. 如果 `json` 是一个对象而不是数组,那么你不能直接索引它。你需要先检查 `json.features` 是否存在,再调用 `readFeatures()`。
修复这个问题,你可以添加一些错误检查和类型判断:
```javascript
if (typeof json === 'object' && Array.isArray(json)) {
const features = json.features || [];
if (features.length > 0) {
const geoJson = new GeoJSON();
const parsedFeatures = geoJson.readFeatures(features);
// ... 使用parsedFeatures
} else {
console.error('Invalid GeoJSON format or no features found.');
}
} else {
console.error('json is not a valid GeoJSON array.');
}
```
Cannot read properties of undefined (reading geoJson )
This error typically occurs when you are trying to access a property of an object that is undefined. In this case, it seems like you are trying to access the `geoJson` property of an object, but the object itself is undefined.
To fix this issue, you should ensure that the object containing the `geoJson` property is properly initialized before accessing it. You can use conditional statements or null/undefined checks to avoid accessing properties on undefined objects.
Here's an example of how you can handle this situation:
```javascript
if (myObject && myObject.geoJson) {
// Access the geoJson property here
console.log(myObject.geoJson);
} else {
// Handle the case when the object or geoJson property is undefined
console.log("Object or geoJson property is undefined.");
}
```
By performing these checks, you can prevent the "Cannot read properties of undefined" error from occurring.
阅读全文
相关推荐
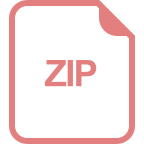
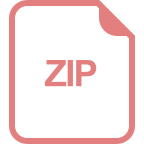










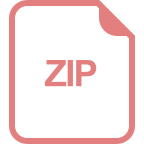
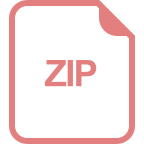
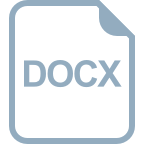