2.设计一个Circle(圆)类,该类中包括圆心位置为坐标(0,0)、半径0.5、颜色为‘蓝色’等属性,还包括构造方法和计算周长和面积的方法。设计完成后,请测试类的功能。
时间: 2024-12-17 15:19:46 浏览: 3
我可以帮你设计一个简单的Circle类,首先包含所需属性和方法:
```java
public class Circle {
private double centerX;
private double centerY;
private double radius;
private String color; // 默认值设为"蓝色"
public Circle() {
this.centerX = 0;
this.centerY = 0;
this.radius = 0.5;
this.color = "蓝色";
}
public Circle(double centerX, double centerY, double radius) {
this.centerX = centerX;
this.centerY = centerY;
this.radius = radius;
this.color = "蓝色"; // 如果需要自定义颜色,这里可以添加参数接收颜色值
}
// 计算周长的方法
public double getPerimeter() {
return Math.PI * 2 * radius;
}
// 计算面积的方法
public double getArea() {
return Math.PI * Math.pow(radius, 2);
}
// 获取圆的相关信息
@Override
public String toString() {
return "Circle [centerX=" + centerX + ", centerY=" + centerY + ", radius=" + radius + ", color=" + color + "]";
}
}
// 测试Circle类
public static void main(String[] args) {
Circle circle1 = new Circle(); // 使用默认构造函数创建圆
System.out.println("Circle 1: " + circle1);
Circle circle2 = new Circle(3, 4, 1); // 自定义参数创建圆
System.out.println("Circle 2: " + circle2);
System.out.printf("Perimeter of Circle 1: %.2f\n", circle1.getPerimeter());
System.out.printf("Area of Circle 1: %.2f\n", circle1.getArea());
}
```
在这个例子中,我们创建了一个Circle类,包含了圆心位置、半径和颜色属性,以及构造方法和计算周长和面积的方法。在`main`方法中对这个类进行了测试。
阅读全文
相关推荐
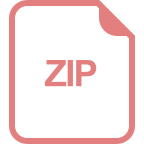
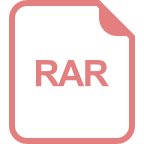
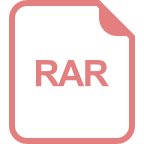















