图像配准Python
时间: 2023-11-19 16:52:16 浏览: 60
好的,关于图像配准Python,我可以给你一些基本的介绍和方法。
图像配准是指将两幅或多幅图像进行对齐,使它们在空间上重合。在Python中,有很多库可以用来进行图像配准,比如OpenCV、scikit-image等。
其中,OpenCV是一个非常强大的计算机视觉库,提供了很多图像处理和计算机视觉算法。在OpenCV中,可以使用cv2.findHomography()函数来进行图像配准。
具体步骤如下:
1. 读取待配准的两幅图像;
2. 提取两幅图像的特征点;
3. 使用特征点匹配算法(如SIFT、SURF、ORB等)找到两幅图像中相同的特征点;
4. 使用cv2.findHomography()函数计算变换矩阵;
5. 使用cv2.warpPerspective()函数将待配准的图像进行变换。
相关问题
图像配准python
图像配准是将两个或多个图像对齐的过程,使得它们在空间上具有相同的位置和方向。在Python中,可以使用OpenCV和scikit-image等库来实现图像配准。
一种常用的图像配准方法是基于特征的配准。以下是使用OpenCV和scikit-image库进行图像配准的示例代码:
使用OpenCV进行特征提取和匹配:
```python
import cv2
# 读取两个图像
image1 = cv2.imread('image1.jpg', 0)
image2 = cv2.imread('image2.jpg', 0)
# 创建SIFT特征提取器
sift = cv2.SIFT_create()
# 在两个图像上检测关键点和描述符
keypoints1, descriptors1 = sift.detectAndCompute(image1, None)
keypoints2, descriptors2 = sift.detectAndCompute(image2, None)
# 创建FLANN匹配器
matcher = cv2.FlannBasedMatcher()
# 使用knnMatch进行特征匹配
matches = matcher.knnMatch(descriptors1, descriptors2, k=2)
# 进行比值测试以剔除错误匹配
good_matches = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good_matches.append(m)
# 获取匹配点的坐标
points1 = [keypoints1[m.queryIdx].pt for m in good_matches]
points2 = [keypoints2[m.trainIdx].pt for m in good_matches]
# 将坐标转换为numpy数组
points1 = np.float32(points1).reshape(-1, 1, 2)
points2 = np.float32(points2).reshape(-1, 1, 2)
# 使用findHomography计算变换矩阵
M, mask = cv2.findHomography(points1, points2, cv2.RANSAC, 5.0)
# 应用变换矩阵将图像1配准到图像2
result = cv2.warpPerspective(image1, M, (image2.shape[1], image2.shape[0]))
# 显示配准结果
cv2.imshow('Registered Image', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
使用scikit-image进行基于相似性变换的配准:
```python
from skimage.feature import match_template
import matplotlib.pyplot as plt
# 读取两个图像
image1 = plt.imread('image1.jpg')
image2 = plt.imread('image2.jpg')
# 使用match_template进行相似性匹配
result = match_template(image1, image2)
# 获取最佳匹配位置
y, x = np.unravel_index(np.argmax(result), result.shape)
# 计算平移向量
shift = (x - image1.shape[1], y - image1.shape[0])
# 将图像1平移以配准到图像2
registered_image = np.roll(image1, shift, axis=(0, 1))
# 显示配准结果
plt.imshow(registered_image)
plt.axis('off')
plt.show()
```
这些示例代码分别展示了使用OpenCV和scikit-image库进行图像配准的方法。你可以根据具体的需求选择适合的方法来配准图像。
图像配准 python
图像配准(Image Registration)是指将两个或多个图像(或图像序列)的坐标系进行转换,使它们在相同比例尺度下对齐,以便进行比较、分析和集成。在Python中,可以使用OpenCV和scikit-image库实现图像配准。
1. OpenCV实现图像配准
OpenCV是一个用于计算机视觉的开源库,它提供了多种图像处理和计算机视觉算法。在OpenCV中,可以通过以下步骤实现图像配准:
(1)读取需要配准的图像
import cv2
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
(2)提取图像特征点
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
(3)匹配特征点
matcher = cv2.FlannBasedMatcher()
matches = matcher.knnMatch(des1, des2, k=2)
(4)筛选匹配点
good_matches = []
for m, n in matches:
if m.distance < 0.7 * n.distance:
good_matches.append(m)
(5)计算变换矩阵
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
(6)对图像进行变换
result = cv2.warpPerspective(img1, M, (img2.shape[1], img2.shape[0]))
result = cv2.addWeighted(result, 0.5, img2, 0.5, 0)
2. scikit-image实现图像配准
scikit-image是一个用于图像处理的Python库,它提供了多种图像处理和计算机视觉算法。在scikit-image中,可以通过以下步骤实现图像配准:
(1)读取需要配准的图像
from skimage import io
img1 = io.imread('image1.jpg')
img2 = io.imread('image2.jpg')
(2)提取图像特征点
from skimage.feature import ORB
orb = ORB(n_keypoints=1000)
kp1 = orb.detect(img1)
kp2 = orb.detect(img2)
(3)计算特征点描述符
from skimage.feature import match_descriptors
des1 = orb.extract(img1, kp1)
des2 = orb.extract(img2, kp2)
(4)匹配特征点
from skimage.feature import match_descriptors
matches = match_descriptors(des1, des2)
(5)计算变换矩阵
from skimage.transform import FundamentalMatrixTransform
model, inliers = ransac((src, dst), FundamentalMatrixTransform, min_samples=8,
residual_threshold=1, max_trials=100)
M = model.params
(6)对图像进行变换
from skimage.transform import warp
result = warp(img1, M, output_shape=(img2.shape[0], img2.shape[1]))
result = np.maximum(result, img2)
相关推荐
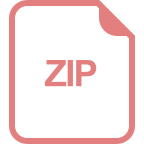
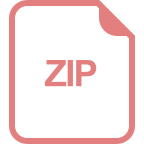
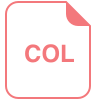
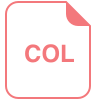
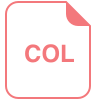
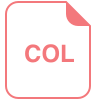
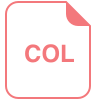







