学生成绩管理系统c++链表结构
时间: 2024-06-29 19:01:21 浏览: 119
学生成绩管理系统中使用C++链表结构可以有效地存储和管理大量的学生信息,因为链表数据结构具有动态性和灵活性,能够方便地添加、删除和修改元素。以下是使用C++链表实现学生成绩管理系统的一个简单概述:
1. **定义节点结构**:
定义一个`StudentNode`类,包含学生的基本属性(如ID、姓名、成绩等)以及指向下一个节点的指针。
```cpp
class StudentNode {
public:
int id;
string name;
double score;
StudentNode* next;
};
```
2. **链表头结点**:
创建一个`StudentList`类,包含一个指向链表头结点的指针,并可能包含一些辅助方法,如初始化、查找、插入、删除等。
```cpp
class StudentList {
private:
StudentNode* head;
public:
StudentList();
~StudentList();
// 插入新学生
void insertStudent(StudentNode* newNode);
// 删除学生
void deleteStudent(int id);
// 查找学生
bool findStudent(int id, StudentNode*& node);
};
```
3. **核心操作**:
- `insertStudent`: 在链表末尾插入新的学生节点。
- `deleteStudent`: 根据学生的ID找到并删除对应节点。
- `findStudent`: 查找特定ID的学生,如果找到返回节点,否则返回false。
```cpp
// 实现插入、删除和查找的方法
void StudentList::insertStudent(StudentNode* newNode) {
if (!head) {
head = newNode;
} else {
StudentNode* current = head;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
}
bool StudentList::findStudent(int id, StudentNode*& node) {
if (!head) return false;
StudentNode* current = head;
while (current && current->id != id) {
current = current->next;
}
node = current;
return current != nullptr;
}
// 删除方法
void StudentList::deleteStudent(int id) {
if (!head) return;
if (head->id == id) {
StudentNode* temp = head;
head = head->next;
delete temp;
return;
}
StudentNode* current = head;
while (current->next && current->next->id != id) {
current = current->next;
}
if (current->next) {
current->next = current->next->next;
delete current->next;
}
}
```
阅读全文
相关推荐



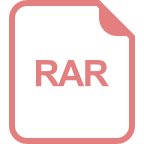




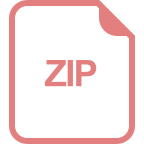
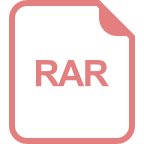
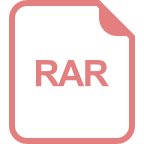





