学生成绩管理系统c++用while switch
时间: 2023-12-19 12:06:47 浏览: 92
学生成绩管理系统是一种用C++编写的程序,它可以帮助学校或老师管理学生的成绩信息。该程序通常包括以下功能:添加学生信息、删除学生信息、修改学生信息、查询学生信息、统计学生总数、计算学生平均分等。在实现这些功能时,通常会使用while循环和switch语句来实现菜单的显示和用户输入的处理。通过while循环,程序可以一直运行,直到用户选择退出程序。而通过switch语句,程序可以根据用户的选择执行相应的操作。在具体实现时,还需要使用链表等数据结构来存储学生信息,同时也需要使用指针等C++语言的特性来实现各种操作。
相关问题
学生成绩管理系统c++
好的,学生成绩管理系统是一个较为常见的小型项目,可以用C++编写实现。
首先,需要设计数据结构,可以使用结构体来表示学生信息和成绩信息。例如:
```c++
struct Student {
string name;
int id;
int age;
string major;
// 其他信息
};
struct Score {
int chinese;
int math;
int english;
// 其他科目成绩
};
```
然后,可以定义一个容器来存储学生信息和成绩信息,可以使用vector、map等STL容器。例如:
```c++
vector<Student> students; // 用于存储学生信息
map<int, Score> scores; // 用于存储成绩信息,键为学生编号
```
接下来,可以定义一些函数来实现学生成绩管理系统的各种功能,例如:
```c++
// 添加学生信息
void addStudent() {
Student student;
// 输入学生信息
students.push_back(student);
}
// 添加学生成绩
void addScore() {
int id;
Score score;
// 输入学生编号和成绩信息
scores[id] = score;
}
// 查询学生信息
void queryStudent() {
int id;
// 根据学生编号查找学生信息
// 输出学生信息
}
// 查询学生成绩
void queryScore() {
int id;
// 根据学生编号查找成绩信息
// 输出成绩信息
}
```
最后,在主函数中调用以上函数来完成相应的功能即可。例如:
```c++
int main() {
while (true) {
// 显示菜单,让用户选择操作
int choice;
cin >> choice;
switch (choice) {
case 1:
addStudent();
break;
case 2:
addScore();
break;
case 3:
queryStudent();
break;
case 4:
queryScore();
break;
case 0:
exit(0);
default:
cout << "Invalid choice." << endl;
}
}
}
```
当然,以上只是简单的代码框架,具体的实现还需要考虑很多细节问题,例如输入格式、错误处理等。
数据结构学生成绩管理系统C++
好的,下面是一个简单的数据结构学生成绩管理系统的C++代码示例,包括添加学生信息、查询学生信息、修改学生信息和删除学生信息等功能:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 学生信息结构体
struct Student {
string name; // 姓名
int id; // 学号
float score; // 成绩
};
// 添加学生信息
void addStudent(vector<Student>& students) {
Student student;
cout << "请输入学生姓名:";
cin >> student.name;
cout << "请输入学生学号:";
cin >> student.id;
cout << "请输入学生成绩:";
cin >> student.score;
students.push_back(student);
cout << "添加成功!" << endl;
}
// 查询学生信息
void queryStudent(vector<Student>& students) {
int id;
cout << "请输入要查询的学生学号:";
cin >> id;
for (int i = 0; i < students.size(); i++) {
if (students[i].id == id) {
cout << "学生姓名:" << students[i].name << endl;
cout << "学生学号:" << students[i].id << endl;
cout << "学生成绩:" << students[i].score << endl;
return;
}
}
cout << "未找到该学生信息!" << endl;
}
// 修改学生信息
void modifyStudent(vector<Student>& students) {
int id;
cout << "请输入要修改的学生学号:";
cin >> id;
for (int i = 0; i < students.size(); i++) {
if (students[i].id == id) {
cout << "请输入新的学生姓名:";
cin >> students[i].name;
cout << "请输入新的学生成绩:";
cin >> students[i].score;
cout << "修改成功!" << endl;
return;
}
}
cout << "未找到该学生信息!" << endl;
}
// 删除学生信息
void deleteStudent(vector<Student>& students) {
int id;
cout << "请输入要删除的学生学号:";
cin >> id;
for (int i = 0; i < students.size(); i++) {
if (students[i].id == id) {
students.erase(students.begin() + i);
cout << "删除成功!" << endl;
return;
}
}
cout << "未找到该学生信息!" << endl;
}
// 主函数
int main() {
vector<Student> students;
int choice;
while (true) {
cout << "请选择要进行的操作:" << endl;
cout << "1. 添加学生信息" << endl;
cout << "2. 查询学生信息" << endl;
cout << "3. 修改学生信息" << endl;
cout << "4. 删除学生信息" << endl;
cout << "5. 退出程序" << endl;
cin >> choice;
switch (choice) {
case 1:
addStudent(students);
break;
case 2:
queryStudent(students);
break;
case 3:
modifyStudent(students);
break;
case 4:
deleteStudent(students);
break;
case 5:
exit(0);
default:
cout << "输入错误,请重新输入!" << endl;
}
}
return 0;
}
```
这只是一个简单的示例代码,实际应用中可能需要更多的功能和更严谨的逻辑处理。
阅读全文
相关推荐



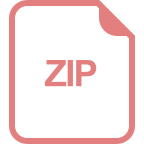









